The following code includes an array of StudentInfo instances: using System; using System.Collections.Generic; using System.Linq; namespace ConsoleApp2 { class LINQtoArray { static void Main(string[] args) { int[] array = { 2, 6, 4, 12, 7, 8, 9, 13, 2 }; var orderedFilteredArray = from element in array where element < 7 orderby element select element; PrintArray(orderedFilteredArray, "All values less than 7 and sorted:"); StudentInfo[] students ={ new StudentInfo("Smith", "John", 12345, "5 Bournbrook Rd"), new StudentInfo("Brown", "Alan", 23412, "Dawlish Rd"), new StudentInfo("Smith","Colin", 41253, "23 Bristol Rd"), new StudentInfo("Hughes", "Richard", 52314, "18 Prichatts Rd"), new StudentInfo("Murphy", "Paul", 16352, "37 College Rd") }; // Filter a range of ID numbers var idRange = from s in students where s.ID > 19999 && s.ID <= 49999 select s; PrintArray(idRange, "Students with ID in Range 2000 to 49999"); // Order by last name and then first name var nameSorted = from s in students orderby s.LastName, s.FirstName select s; PrintArray(nameSorted, "Students sorted in last name, first name order"); } public static void PrintArray(IEnumerable arr, string message) { Console.WriteLine("{0}", message); foreach (var element in arr) Console.WriteLine(" {0}", element); Console.WriteLine(); } } class StudentInfo { public StudentInfo(string ln, string fn, int id, string a) { lastName = ln; firstName = fn; idNumber = id; address = a; } public override string ToString() { return firstName + " " + lastName + " " + idNumber + " " + address; } public string FirstName { get { return firstName; } } public string LastName { get { return lastName; } } public string Address { get { return address; } } public int ID { get { return idNumber; } } private string firstName, lastName; private int idNumber; private string address; } } Create an array of KeanStaff objects using above code as a template. Sort your Objects by salary.
The following code includes an array of StudentInfo instances:
using System;
using System.Collections.Generic;
using System.Linq;
namespace ConsoleApp2
{
class LINQtoArray
{
static void Main(string[] args)
{
int[] array = { 2, 6, 4, 12, 7, 8, 9, 13, 2 };
var orderedFilteredArray =
from element in array
where element < 7
orderby element
select element;
PrintArray(orderedFilteredArray,
"All values less than 7 and sorted:");
StudentInfo[] students ={
new StudentInfo("Smith", "John", 12345, "5 Bournbrook Rd"),
new StudentInfo("Brown", "Alan", 23412, "Dawlish Rd"),
new StudentInfo("Smith","Colin", 41253, "23 Bristol Rd"),
new StudentInfo("Hughes", "Richard", 52314, "18 Prichatts Rd"),
new StudentInfo("Murphy", "Paul", 16352, "37 College Rd") };
// Filter a range of ID numbers
var idRange =
from s in students
where s.ID > 19999 && s.ID <= 49999
select s;
PrintArray(idRange, "Students with ID in Range 2000 to 49999");
// Order by last name and then first name
var nameSorted =
from s in students
orderby s.LastName, s.FirstName
select s;
PrintArray(nameSorted, "Students sorted in last name, first name order");
}
public static void PrintArray<T>(IEnumerable<T> arr, string message)
{
Console.WriteLine("{0}", message);
foreach (var element in arr)
Console.WriteLine(" {0}", element);
Console.WriteLine();
}
}
class StudentInfo
{
public StudentInfo(string ln, string fn, int id, string a)
{
lastName = ln; firstName = fn; idNumber = id; address = a;
}
public override string ToString()
{
return firstName + " " + lastName + " " + idNumber + " " + address;
}
public string FirstName { get { return firstName; } }
public string LastName { get { return lastName; } }
public string Address { get { return address; } }
public int ID { get { return idNumber; } }
private string firstName, lastName;
private int idNumber;
private string address;
}
}
Create an array of KeanStaff objects using above code as a template. Sort your Objects by salary.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

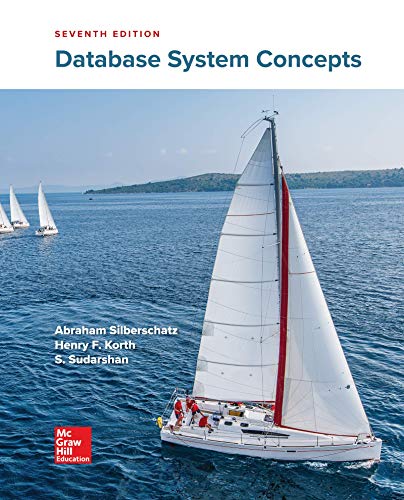
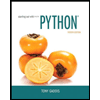
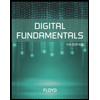
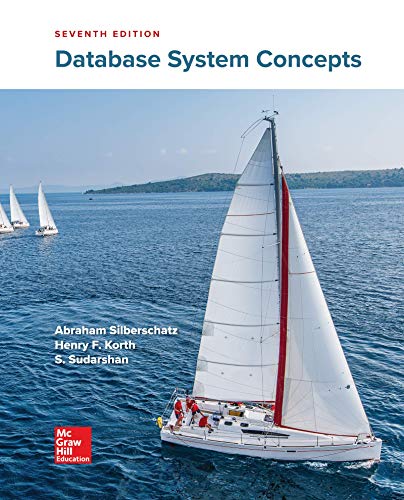
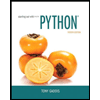
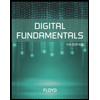
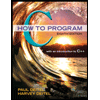
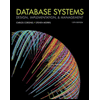
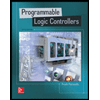