The following class starts to define a Complex number as two numeric (int or float) values, which specify the real and imaginary parts of the Complex number. For example, we can write x = Complex(1,2) to represent the complex number 1+2i (with real part 1 and imaginary part 2) and y = Complex (2,-3) to represent the complex number 2-3i (with real part 2 and imaginary part -3). In the Complex class below, overload (a) the method called by the repr function, producing the standard result (b) the method called by the str function: the correct sign (+ or -) must appear between the real and imaginary parts: for x and y above, str(x) returns '1+2i' and str(y) returns '2-3i'. For Complex(0,0) it returns '0+0i' (c) the addition operator: we can compute the sum of two complex numbers or the sum of a complex number and an int or float (these two types are pure real: each has 0 as its imaginary part) by adding the real parts of each and adding the imaginary parts; 2+3i plus 1+2i is 3+5i; likewise, 2+3i plus 1 (or 1 plus 2+3i) is 3+3i. For any other type of operands, + should raise a TypeError with an appropriate error message. class Complex: # Assume this method initializes the two attributes of a Complex object def __init__(self,real,imaginary): self.r = real self.i = imaginary # overload the method called by repr # overload the method called by str # overload + allowing Complex + Complex, Complex + int, int + Complex, # Complex + float, and float + Complex
The following class starts to define a Complex number as two numeric (int or float) values, which specify the real and imaginary parts of the Complex number. For example, we can write x = Complex(1,2) to represent the complex number 1+2i (with real part 1 and imaginary part 2) and y = Complex (2,-3) to represent the complex number 2-3i (with real part 2 and imaginary part -3).
In the Complex class below, overload
(a) the method called by the repr function, producing the standard result
(b) the method called by the str function: the correct sign (+ or -) must appear between the real and imaginary parts: for x and y above, str(x) returns '1+2i' and str(y) returns '2-3i'. For Complex(0,0) it returns '0+0i'
(c) the addition operator: we can compute the sum of two complex numbers or the sum of a complex number and an int or float (these two types are pure real: each has 0 as its imaginary part) by adding the real parts of each and adding the imaginary parts; 2+3i plus 1+2i is 3+5i; likewise, 2+3i plus 1 (or 1 plus 2+3i) is 3+3i. For any other type of operands, + should raise a TypeError with an appropriate error message.
class Complex:
# Assume this method initializes the two attributes of a Complex object def __init__(self,real,imaginary):
self.r = real self.i = imaginary
# overload the method called by repr # overload the method called by str
# overload + allowing Complex + Complex, Complex + int, int + Complex, # Complex + float, and float + Complex

Class Creation:
- Create a class named Complex.
Initialization Method (init):
- Define an
__init__
method in the Complex class. - Input: real (an int or float), imaginary (an int or float)
- Set
self.r
to the value ofreal
. - Set
self.i
to the value ofimaginary
.
- Define an
Representation Method (repr):
- Define an
__repr__
method in the Complex class. - Return a string representation of the Complex object in the format "real+imaginaryi."
- Example: "3+4i" if real is 3 and imaginary is 4.
- Define an
String Representation Method (str):
- Define an
__str__
method in the Complex class. - Check if the imaginary part (
self.i
) is greater than or equal to 0. - If true:
- Return a string in the format "real+imaginaryi."
- If false:
- Return a string in the format "realimaginaryi" with no '+' sign before the imaginary part.
- Example: "3+4i" if real is 3 and imaginary is 4, and "3-4i" if real is 3 and imaginary is -4.
- Define an
Addition Method (add):
- Define an
__add__
method in the Complex class. - Input:
other
(another Complex object or int or float) - Check the type of
other
:- If
other
is a Complex object:- Add the real parts and imaginary parts of
self
andother
. - Create a new Complex object with the result.
- Add the real parts and imaginary parts of
- If
other
is an int or float:- Add the real part of
self
andother
. - Keep the imaginary part of
self
unchanged. - Create a new Complex object with the result.
- Add the real part of
- If
other
is not a Complex, int, or float:- Raise a TypeError with an appropriate error message.
- If
- Define an
End of the Complex Class.
Step by step
Solved in 5 steps with 2 images

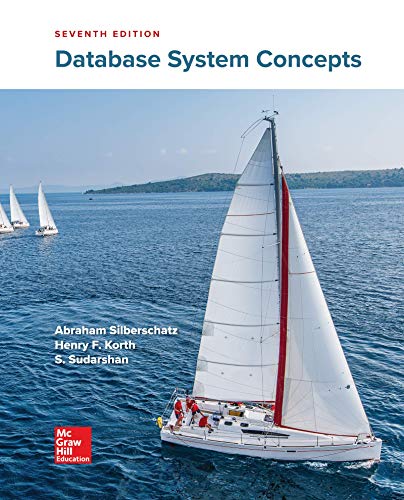
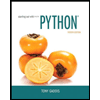
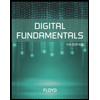
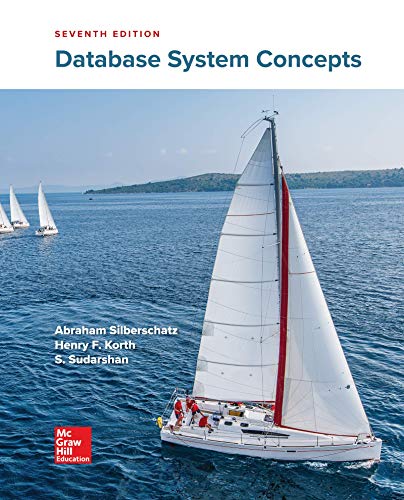
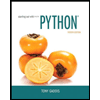
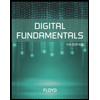
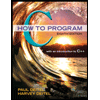
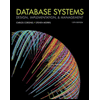
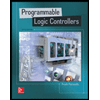