The file DebugFour4.cs has syntax and/or logical errors. Determine the problem(s), and fix the program. // Program computes sales commission based on the following: // Sales up to and including $1,000 -- 5% commission // Up to and including $5,000 -- 5% on first $1,000 and // 7% commission on amount over $1,000 // up to and including $10,000 -- same as before plus $1,000 bonus // over $10,000 - same as all of the above plus // additional $1,500 bonus using System; using static System.Console; using System.Globalization; class DebugFour4 { static void Main() { double sales, commission; string inputString; const int LOWSALES = 1000; const int MEDSALES = 5000; const int HIGHSALES = 10000; const double LOWPCT = 0.05; const double MEDPCT = 0.02; const int BONUS1 = 1000; const int BONUS2 = 1500; WriteLine("What was the sales amount? "); inputString = ReadLine(); sales = Convert.ToDouble(inputString); commission = LOWPCT * sales; if(sales <= LOWSALES) commission += (sales - LOWSALES) * MEDPCT; else if(sales == MEDSALES) commission += BONUS1; else if(sales > HIGHSALES) commission = BONUS2; WriteLine("Sales: {0}\nCommission: {1}", sales.ToString("C", CultureInfo.GetCultureInfo("en-US")), commission.ToString("C", CultureInfo.GetCultureInfo("en-US"))); } }
The file DebugFour4.cs has syntax and/or logical errors. Determine the problem(s), and fix the program. // Program computes sales commission based on the following: // Sales up to and including $1,000 -- 5% commission // Up to and including $5,000 -- 5% on first $1,000 and // 7% commission on amount over $1,000 // up to and including $10,000 -- same as before plus $1,000 bonus // over $10,000 - same as all of the above plus // additional $1,500 bonus using System; using static System.Console; using System.Globalization; class DebugFour4 { static void Main() { double sales, commission; string inputString; const int LOWSALES = 1000; const int MEDSALES = 5000; const int HIGHSALES = 10000; const double LOWPCT = 0.05; const double MEDPCT = 0.02; const int BONUS1 = 1000; const int BONUS2 = 1500; WriteLine("What was the sales amount? "); inputString = ReadLine(); sales = Convert.ToDouble(inputString); commission = LOWPCT * sales; if(sales <= LOWSALES) commission += (sales - LOWSALES) * MEDPCT; else if(sales == MEDSALES) commission += BONUS1; else if(sales > HIGHSALES) commission = BONUS2; WriteLine("Sales: {0}\nCommission: {1}", sales.ToString("C", CultureInfo.GetCultureInfo("en-US")), commission.ToString("C", CultureInfo.GetCultureInfo("en-US"))); } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
The file DebugFour4.cs has syntax and/or logical errors. Determine the problem(s), and fix the
// Program computes sales commission based on the following:
// Sales up to and including $1,000 -- 5% commission
// Up to and including $5,000 -- 5% on first $1,000 and
// 7% commission on amount over $1,000
// up to and including $10,000 -- same as before plus $1,000 bonus
// over $10,000 - same as all of the above plus
// additional $1,500 bonus
using System;
using static System.Console;
using System.Globalization;
class DebugFour4
{
static void Main()
{
double sales, commission;
string inputString;
const int LOWSALES = 1000;
const int MEDSALES = 5000;
const int HIGHSALES = 10000;
const double LOWPCT = 0.05;
const double MEDPCT = 0.02;
const int BONUS1 = 1000;
const int BONUS2 = 1500;
WriteLine("What was the sales amount? ");
inputString = ReadLine();
sales = Convert.ToDouble(inputString);
commission = LOWPCT * sales;
if(sales <= LOWSALES)
commission += (sales - LOWSALES) * MEDPCT;
else
if(sales == MEDSALES)
commission += BONUS1;
else
if(sales > HIGHSALES)
commission = BONUS2;
WriteLine("Sales: {0}\nCommission: {1}",
sales.ToString("C", CultureInfo.GetCultureInfo("en-US")), commission.ToString("C", CultureInfo.GetCultureInfo("en-US")));
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
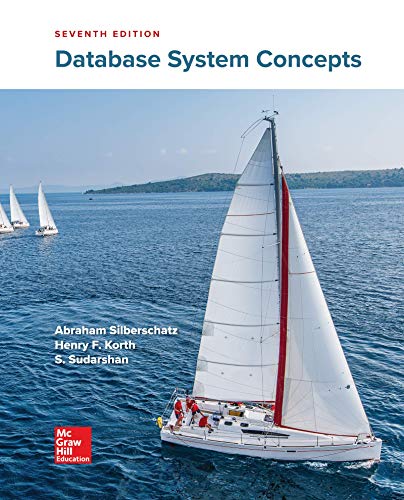
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
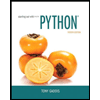
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
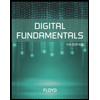
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
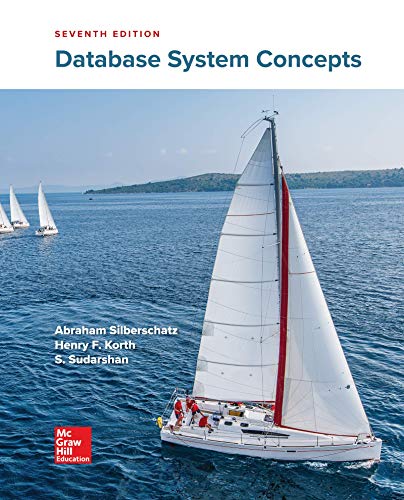
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
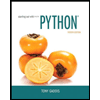
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
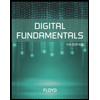
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
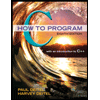
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
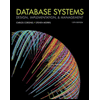
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
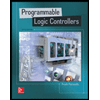
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education