The file Parameters.java contains a program to test the variable-length method average. 1. Read the code. Fill in the code to compute mean1 and mean2. Notice that you are calling the same method, but with a different number of parameters. 2. Add a call to find the average of a single integer, say 8. Print the result of the call. Is the result what you expected? 3. Add a call with an empty parameter list and print the result. Is the result what you expected? 4. Add a method called minimum to return the minimum value of the parameter list. Invoke this method in the main() and test it with different input numbers. //******************************************************* // Parameters.java // // Illustrates the concept of a variable parameter list. //******************************************************* import java.util.Scanner; public class Parameters { //----------------------------------------------- // Calls the average and minimum methods with // different numbers of parameters. //----------------------------------------------- public static void main(String[] args) { double mean1, mean2; mean1 = // Your code:calculate average of 11,12 and 18 mean2 = // Your code:calculate average of 11,12,18 and 75; System.out.println ("mean1 = " + mean1); System.out.println ("mean2 = " + mean2); } //---------------------------------------------- // Returns the average of its parameters. //---------------------------------------------- public static double average (int ... list) { double result = 0.0; if (list.length != 0) { int sum = 0; for (int num: list) sum += num; result = (double)sum / list.length; } return result; } }
The file Parameters.java contains a program to test the variable-length method average.
1. Read the code. Fill in the code to compute mean1 and mean2. Notice that you are calling the same method, but with a different number of parameters.
2. Add a call to find the average of a single integer, say 8. Print the result of the call. Is the result what you expected?
3. Add a call with an empty parameter list and print the result. Is the result
what you expected?
4. Add a method called minimum to return the minimum value of the
parameter list. Invoke this method in the main() and test it with different
input numbers.
//*******************************************************
// Parameters.java
//
// Illustrates the concept of a variable parameter list.
//*******************************************************
import java.util.Scanner;
public class Parameters
{
//-----------------------------------------------
// Calls the average and minimum methods with
// different numbers of parameters.
//-----------------------------------------------
public static void main(String[] args)
{
double mean1, mean2;
mean1 = // Your code:calculate average of 11,12 and 18
mean2 = // Your code:calculate average of 11,12,18 and 75;
System.out.println ("mean1 = " + mean1);
System.out.println ("mean2 = " + mean2);
}
//----------------------------------------------
// Returns the average of its parameters.
//----------------------------------------------
public static double average (int ... list)
{
double result = 0.0;
if (list.length != 0)
{
int sum = 0;
for (int num: list)
sum += num;
result = (double)sum / list.length;
}
return result;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

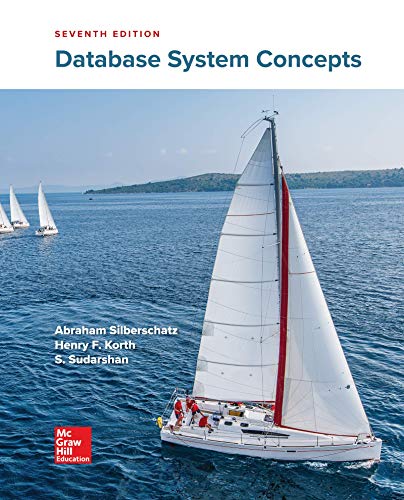
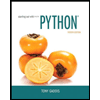
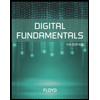
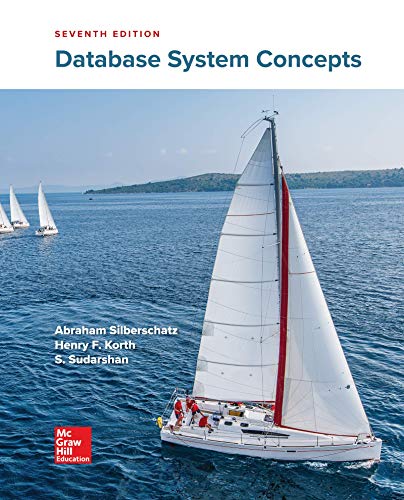
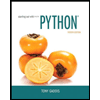
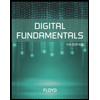
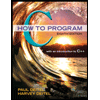
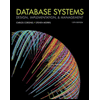
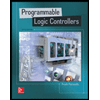