i just need you to add explanation comments after every line of code in this following code i have to stand infront of my teacher and class and i have to explain this code to them so please make sure you give a very detailed explanation for each line of code for the following import tkinter as tk class ATM: def __init__(self): self.accounts = {} def create_account(self): first_name = first_name_entry.get() last_name = last_name_entry.get() dob = dob_entry.get() address = address_entry.get() debit_card = debit_card_entry.get() while len(debit_card) != 16 or not debit_card.isdigit(): debit_card = input("Invalid debit card number. Enter debit card number (16 digits): ") pin = pin_entry.get() while len(pin) != 4 or not pin.isdigit(): pin = input("Invalid pin. Enter 4-digit pin: ") self.accounts[debit_card] = {"first_name": first_name, "last_name": last_name, "dob": dob, "address": address, "pin": pin, "balance": 0} status_label.config(text="Account created successfully!") def login(self): debit_card = login_debit_card_entry.get() pin = login_pin_entry.get() if debit_card in self.accounts and self.accounts[debit_card]["pin"] == pin: while True: choice = login_choice_entry.get() if choice == "1": amount = float(deposit_entry.get()) self.accounts[debit_card]["balance"] += amount status_label.config(text="Deposit successful. Current balance : $%.2f" % self.accounts[debit_card]["balance"]) break elif choice == "2": amount = float(withdraw_entry.get()) if amount <= self.accounts[debit_card]["balance"]: self.accounts[debit_card]["balance"] -= amount status_label.config(text="Withdraw successful. Current balance : $%.2f" % self.accounts[debit_card]["balance"]) break else: status_label.config(text="Insufficient balance.") break elif choice == "3": status_label.config(text="Current balance: $%.2f" % self.accounts[debit_card]["balance"]) break elif choice == "4": status_label.config(text="Logged out.") break else: status_label.config(text="Invalid choice.") break else: status_label.config(text="Invalid debit card or pin.") atm = ATM() # create GUI root = tk.Tk() root.title("ATM software") create_account_frame = tk.Frame(root) create_account_frame.pack(padx=10, pady=10) tk.Label(create_account_frame, text="Enter first name:").grid(row=0, column=0) first_name_entry = tk.Entry(create_account_frame) first_name_entry.grid(row=0, column=1) tk.Label(create_account_frame, text="Enter last name:").grid(row=1, column=0) last_name_entry = tk.Entry(create_account_frame) last_name_entry.grid(row=1, column=1) tk.Label(create_account_frame, text="Enter date of birth (dd/mm/yyyy):").grid(row=2, column=0) dob_entry = tk.Entry(create_account_frame) dob_entry.grid(row=2, column=1) tk.Label(create_account_frame, text="Enter address in this format (111 s 1st ave tucson, az, 85711) :").grid(row=3, column=0) address_entry = tk.Entry(create_account_frame) address_entry.grid(row=3, column=1) tk.Label(create_account_frame, text="Enter debit card number (16 digits):").grid(row=4, column=0) debit_card_entry = tk.Entry(create_account_frame) debit_card_entry.grid(row=4, column=1) tk.Label(create_account_frame, text="Enter 4-digit pin:").grid(row=5, column=0) pin_entry = tk.Entry(create_account_frame, show="*") pin_entry.grid(row=5, column=1) create_account_button = tk.Button(create_account_frame, text="Create account", command=atm.create_account) create_account_button.grid(row=6, column=0, columnspan=2) login_frame = tk.Frame(root) login_frame.pack(padx=10, pady=10) tk.Label(login_frame, text="Enter debit card number:").grid(row=0, column=0) login_debit_card_entry = tk.Entry(login_frame) login_debit_card_entry.grid(row=0, column=1) tk.Label(login_frame, text="Enter 4-digit pin:").grid(row=1, column=0) login_pin_entry = tk.Entry(login_frame, show="*") login_pin_entry.grid(row=1, column=1) tk.Label(login_frame, text="1. Deposit\n2. Withdraw\n3. Check balance\n4. Logout").grid(row=2, column=0) login_choice_entry = tk.Entry(login_frame) login_choice_entry.grid(row=2, column=1) deposit_entry = tk.Entry(login_frame) deposit_entry.grid(row=3, column=0) withdraw_entry = tk.Entry(login_frame) withdraw_entry.grid(row=3, column=1) login_button = tk.Button(login_frame, text="Login", command=atm.login) login_button.grid(row=4, column=0, columnspan=2) status_label = tk.Label(root, text="") status_label.pack() root.mainloop()
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
i just need you to add explanation comments after every line of code in this following code
i have to stand infront of my teacher and class and i have to explain this code to them so please make sure you give a very detailed explanation for each line of code for the following
import tkinter as tk
class ATM:
def __init__(self):
self.accounts = {}
def create_account(self):
first_name = first_name_entry.get()
last_name = last_name_entry.get()
dob = dob_entry.get()
address = address_entry.get()
debit_card = debit_card_entry.get()
while len(debit_card) != 16 or not debit_card.isdigit():
debit_card = input("Invalid debit card number. Enter debit card number (16 digits): ")
pin = pin_entry.get()
while len(pin) != 4 or not pin.isdigit():
pin = input("Invalid pin. Enter 4-digit pin: ")
self.accounts[debit_card] = {"first_name": first_name, "last_name": last_name, "dob": dob, "address": address,
"pin": pin, "balance": 0}
status_label.config(text="Account created successfully!")
def login(self):
debit_card = login_debit_card_entry.get()
pin = login_pin_entry.get()
if debit_card in self.accounts and self.accounts[debit_card]["pin"] == pin:
while True:
choice = login_choice_entry.get()
if choice == "1":
amount = float(deposit_entry.get())
self.accounts[debit_card]["balance"] += amount
status_label.config(text="Deposit successful. Current balance : $%.2f" % self.accounts[debit_card]["balance"])
break
elif choice == "2":
amount = float(withdraw_entry.get())
if amount <= self.accounts[debit_card]["balance"]:
self.accounts[debit_card]["balance"] -= amount
status_label.config(text="Withdraw successful. Current balance : $%.2f" % self.accounts[debit_card]["balance"])
break
else:
status_label.config(text="Insufficient balance.")
break
elif choice == "3":
status_label.config(text="Current balance: $%.2f" % self.accounts[debit_card]["balance"])
break
elif choice == "4":
status_label.config(text="Logged out.")
break
else:
status_label.config(text="Invalid choice.")
break
else:
status_label.config(text="Invalid debit card or pin.")
atm = ATM()
# create GUI
root = tk.Tk()
root.title("ATM software")
create_account_frame = tk.Frame(root)
create_account_frame.pack(padx=10, pady=10)
tk.Label(create_account_frame, text="Enter first name:").grid(row=0, column=0)
first_name_entry = tk.Entry(create_account_frame)
first_name_entry.grid(row=0, column=1)
tk.Label(create_account_frame, text="Enter last name:").grid(row=1, column=0)
last_name_entry = tk.Entry(create_account_frame)
last_name_entry.grid(row=1, column=1)
tk.Label(create_account_frame, text="Enter date of birth (dd/mm/yyyy):").grid(row=2, column=0)
dob_entry = tk.Entry(create_account_frame)
dob_entry.grid(row=2, column=1)
tk.Label(create_account_frame, text="Enter address in this format (111 s 1st ave tucson, az, 85711) :").grid(row=3, column=0)
address_entry = tk.Entry(create_account_frame)
address_entry.grid(row=3, column=1)
tk.Label(create_account_frame, text="Enter debit card number (16 digits):").grid(row=4, column=0)
debit_card_entry = tk.Entry(create_account_frame)
debit_card_entry.grid(row=4, column=1)
tk.Label(create_account_frame, text="Enter 4-digit pin:").grid(row=5, column=0)
pin_entry = tk.Entry(create_account_frame, show="*")
pin_entry.grid(row=5, column=1)
create_account_button = tk.Button(create_account_frame, text="Create account", command=atm.create_account)
create_account_button.grid(row=6, column=0, columnspan=2)
login_frame = tk.Frame(root)
login_frame.pack(padx=10, pady=10)
tk.Label(login_frame, text="Enter debit card number:").grid(row=0, column=0)
login_debit_card_entry = tk.Entry(login_frame)
login_debit_card_entry.grid(row=0, column=1)
tk.Label(login_frame, text="Enter 4-digit pin:").grid(row=1, column=0)
login_pin_entry = tk.Entry(login_frame, show="*")
login_pin_entry.grid(row=1, column=1)
tk.Label(login_frame, text="1. Deposit\n2. Withdraw\n3. Check balance\n4. Logout").grid(row=2, column=0)
login_choice_entry = tk.Entry(login_frame)
login_choice_entry.grid(row=2, column=1)
deposit_entry = tk.Entry(login_frame)
deposit_entry.grid(row=3, column=0)
withdraw_entry = tk.Entry(login_frame)
withdraw_entry.grid(row=3, column=1)
login_button = tk.Button(login_frame, text="Login", command=atm.login)
login_button.grid(row=4, column=0, columnspan=2)
status_label = tk.Label(root, text="")
status_label.pack()
root.mainloop()

Comments in code are lines that are meant for human readers, not the computer. They provide explanations or notes about what the code is doing. These comments help programmers understand the purpose and functionality of different parts of the code, making it easier to collaborate, maintain, and debug the program.
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

the comments explanation is incomplete, ypu didnt provide explanation for each line, you skipped many
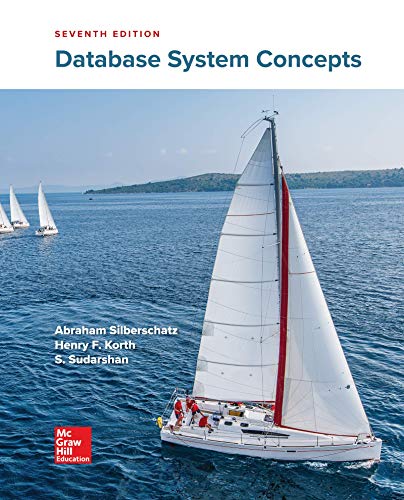
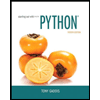
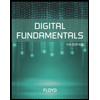
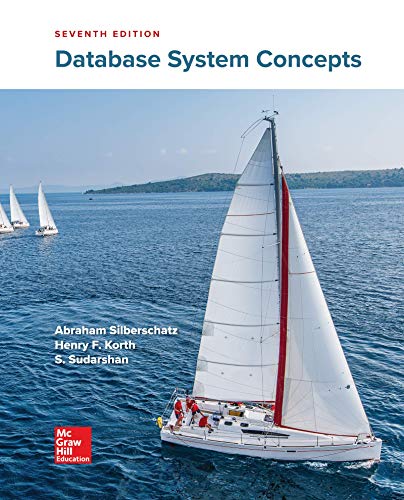
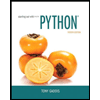
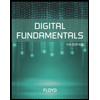
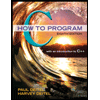
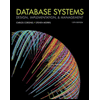
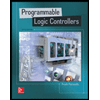