The class Store has to implement the following functionalities 1 public class Store 2 { 3 4 //Fields TODO 5 6 //Initializes the fields of the store object 7 public Store(String shopName) 8 { 9 //TODO 10 } 11 12 //Adds a product with name = id and price = price to the catalogue of products sold by the StoreChain 13 //Returns true if the operation is successful (i.e., you can add a new product that doesn’t already exist) 14 public static boolean addNewProduct(String id, double price) 15 { 16 //TODO 17 } 18 19 //Removes a product from the catalogue of the StoreChain 20 //Returns the removed Product if the operation is successful (i.e, the product existed in the catalogue), null otherwise 21 public static Product removeFromCatalogue(String id) 22 { 23 //TODO 24 } 25 26 //Changes the price of a product in the catalogue of the StoreChain 27 //Returns true if the operation is successful (i.e., you reprice an existing product) 28 public static boolean repriceProduct(String id, double price) 29 { 30 //TODO 31 } 32 33 //Stocks a product (buys a certain quantity) in a store of the StoreChain If the product is already stocked in the store, adds the new quantity to the already available amount. 34 //Returns true if the operation is successful (i.e., the product is ( still) available in the catalogue of the StoreChain) 35 public boolean stockProduct(String id, int quantity) { 36 //TODO 37 } 38 39 //Sells a product from the store (reducing the quantity in the stock accordingly): a store could even sell a product which is still in its stock, but no longer available in the catalogue of the StoreChain 40 //Returns true if the operation is successful (ie., you have enough product to be sold) 41 public boolean sellProduct(String id, int quantity) { 42 //TODO 43 } 44 45 //Prints the contents of a catalogue: product name and price (one product per line) 46 public static void printCatalogue() { 47 //TODO 48 } 49 50 //Prints the stock of the current store object: product, price and quantity (one per line) 51 public void printStoreStock() { 52 //TODO 53 } 54 }
The class Store has to implement the following functionalities
1 public class Store
2 {
3
4 //Fields TODO
5
6 //Initializes the fields of the store object
7 public Store(String shopName)
8 {
9 //TODO
10 }
11
12 //Adds a product with name = id and price = price to the catalogue of
products sold by the StoreChain
13 //Returns true if the operation is successful (i.e., you can add a new
product that doesn’t already exist)
14 public static boolean addNewProduct(String id, double price)
15 {
16 //TODO
17 }
18
19 //Removes a product from the catalogue of the StoreChain
20 //Returns the removed Product if the operation is successful (i.e, the
product existed in the catalogue), null otherwise
21 public static Product removeFromCatalogue(String id)
22 {
23 //TODO
24 }
25
26 //Changes the price of a product in the catalogue of the StoreChain
27 //Returns true if the operation is successful (i.e., you reprice an
existing product)
28 public static boolean repriceProduct(String id, double price)
29 {
30 //TODO
31 }
32
33 //Stocks a product (buys a certain quantity) in a store of the StoreChain
If the product is already stocked in the store, adds the new quantity
to the already available amount.
34 //Returns true if the operation is successful (i.e., the product is (
still) available in the catalogue of the StoreChain)
35 public boolean stockProduct(String id, int quantity) {
36 //TODO
37 }
38
39 //Sells a product from the store (reducing the quantity in the stock
accordingly): a store could even sell a product which is still in its
stock, but no longer available in the catalogue of the StoreChain
40 //Returns true if the operation is successful (ie., you have enough
product to be sold)
41 public boolean sellProduct(String id, int quantity) {
42 //TODO
43 }
44
45 //Prints the contents of a catalogue: product name and price (one product
per line)
46 public static void printCatalogue() {
47 //TODO
48 }
49
50 //Prints the stock of the current store object: product, price and
quantity (one per line)
51 public void printStoreStock() {
52 //TODO
53 }
54 }

Step by step
Solved in 3 steps with 1 images

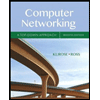
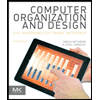
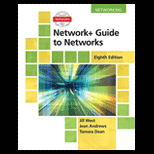
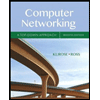
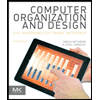
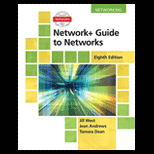
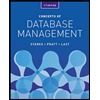
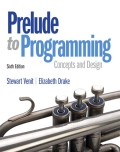
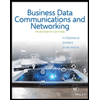