The array contains all 10 different integers. Store them in a linked list in order and print them out.
The array contains all 10 different integers. Store them in a linked list in order and print them out.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Use C++

Transcribed Image Text:```cpp
#include <iostream>
using namespace std;
typedef struct node* NodePtr;
struct node
{
public:
int data;
NodePtr link;
};
void print(NodePtr head)
{
for (NodePtr ptr = head; ptr != NULL; ptr = ptr->link)
cout << ptr->data << " ";
cout << endl;
}
```
### Explanation:
This C++ code defines a simple structure and a function to work with a linked list:
1. **Header and Namespace:**
- `#include <iostream>`: This line includes the input-output stream library which is necessary for using `cout`.
- `using namespace std;`: This line allows direct access to the standard library objects and functions without prefixing them with `std::`.
2. **Node Structure:**
- A node in the linked list is defined using a struct called `node`.
- It contains:
- `int data;`: An integer to store data.
- `NodePtr link;`: A pointer (`NodePtr`) to the next node in the list.
3. **Typedef Declaration:**
- `typedef struct node* NodePtr;` creates a type alias `NodePtr` for a pointer to a `node` structure.
4. **Print Function:**
- `void print(NodePtr head)`: This function takes the head of the list as an argument and prints each node’s data.
- Inside the function, a for-loop iterates through the list until it reaches the end (`NULL`).
- `cout << ptr->data << " ";` outputs the data of each node followed by a space.
- After the loop, `cout << endl;` outputs a newline character.
![**Problem 1**
- The array contains all 10 different integers. Store them in a linked list in order and print them out.
**Array Visualization:**
```
4
3 4
3 4 6
3 4 6 1
3 4 6 1 10
1 3 4 6 9 10
1 3 4 5 6 9 10
1 3 4 5 6 8 9 10
1 3 4 5 6 7 8 9 10
1 2 3 4 5 6 7 8 9 10
```
**C++ Code:**
```cpp
int main()
{
int A[10] = { 4, 3, 6, 1, 10, 9, 5, 8, 7, 2 };
return 0;
}
```
**Explanation of the Task:**
- You are given an array consisting of 10 different integers. The task is to store these integers into a data structure called a linked list, where they should be arranged in order. Finally, you are required to print the ordered sequence of integers.
**Explanation of the Diagram:**
- The diagram on the left visually represents the process of ordering the integers step by step. Each step shows the intermediate ordering of integers as new elements are added:
- Starts with adding the integer 4.
- Then adds 3 and places it in the correct position before 4.
- The process continues, adding each subsequent integer and inserting it into its correct position in the sequence, resulting in a fully ordered sequence by the final step.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fcdbdf4c8-5459-45de-99f8-cd8d71031beb%2F17201c5d-7615-431f-964a-ebf3ab9a9266%2F2pyz15lk_processed.png&w=3840&q=75)
Transcribed Image Text:**Problem 1**
- The array contains all 10 different integers. Store them in a linked list in order and print them out.
**Array Visualization:**
```
4
3 4
3 4 6
3 4 6 1
3 4 6 1 10
1 3 4 6 9 10
1 3 4 5 6 9 10
1 3 4 5 6 8 9 10
1 3 4 5 6 7 8 9 10
1 2 3 4 5 6 7 8 9 10
```
**C++ Code:**
```cpp
int main()
{
int A[10] = { 4, 3, 6, 1, 10, 9, 5, 8, 7, 2 };
return 0;
}
```
**Explanation of the Task:**
- You are given an array consisting of 10 different integers. The task is to store these integers into a data structure called a linked list, where they should be arranged in order. Finally, you are required to print the ordered sequence of integers.
**Explanation of the Diagram:**
- The diagram on the left visually represents the process of ordering the integers step by step. Each step shows the intermediate ordering of integers as new elements are added:
- Starts with adding the integer 4.
- Then adds 3 and places it in the correct position before 4.
- The process continues, adding each subsequent integer and inserting it into its correct position in the sequence, resulting in a fully ordered sequence by the final step.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
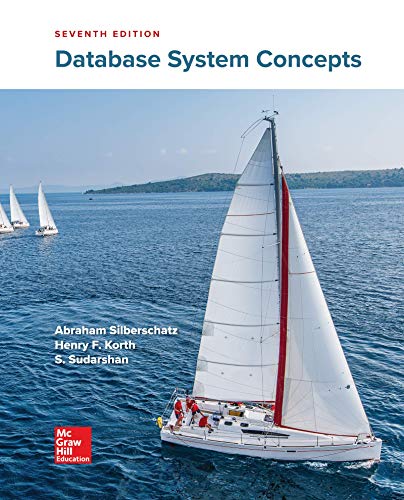
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
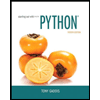
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
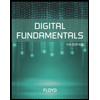
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
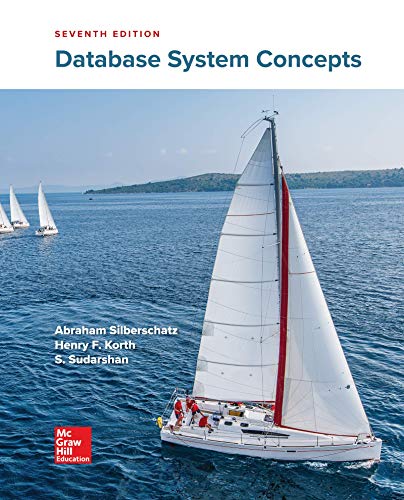
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
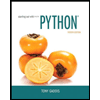
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
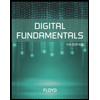
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
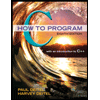
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
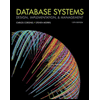
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
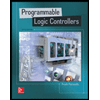
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education