The AdvancedCalculator class represents a calculator that supports multiple expressions over many lines, as well as the use of variables. Lines will be split by semicolons (;), and every token will be separated by a single space. Each line will start with a variable name, then an =' character, then a mathematical expression. The last line will ask to returm a mathematical expression too. You can assume that an expression will not reference variables that have not been defined yet. You can assume that variable names will be consistent and case sensitive. A valid variable name is a non-empty string of alphanumeric characters, the first of which must be a letter. You must use a Calculator to evaluate each expression in this class, otherwise, no credit will be given. Attributes Туре Name Description str expressions The expressions this calculator will evaluate dict states A dictionary mapping variable names to their float values
Please help with 3rd section (in images below), code for first and second class included below. Do not change the function names or given starter code in the script. Each class has different requirements, read them carefully. Do not use the exec or eval functions, nor are you allowed to use regular expressions (re module). All methods that output a string must return the string, not print it. If you are unable to complete a method, use the pass statement to avoid syntax errors.
class Stack:
def __init__(self):
self.stack = []
def pop(self):
if len(self.stack) < 1:
return None
return self.stack.pop()
def push(self, item):
self.stack.append(item)
def size(self):
return len(self.stack)
def peek(self):
return self.stack[self.size() - 1]
def isEmpty(self):
if len(self.stack) < 1:
return True
return False
class Calculator:
def __init__(self):
self.__expr = None
@property
def getExpr(self):
return self.__expr
def setExpr(self, new_expr):
if isinstance(new_expr, str):
self.__expr = new_expr
else:
print('setExpr error: Invalid expression')
return None
def _isNumber(self, txt):
# return True if txt is a string can be convered to a float
try:
float(txt)
return True
except:
return False
def getPriority(self, ch):
# return the priority of an operator
if ch == '^': return 3
if ch == '(': return 0
if ch == '/' or ch == '*': return 2
if ch == '+' or ch == '-': return 1
return 999
def _getPostfix(self, txt):
# convert an expression from infix to postfix
postfixStack = Stack()
postfix = ""
self.setExpr(txt)
ls = self.__expr.split(' ')
ls.insert(0, '(')
ls.append(')')
for st in ls:
# check whether st is float number
if self._isNumber(st):
postfix = postfix + str(float(st)) + ' '
# check if st is an open parenthesis
elif st == '(':
postfixStack.push(st)
# check if st is a close parenthesis
elif st == ')':
while postfixStack.peek() != '(':
postfix = postfix + postfixStack.pop() + ' '
postfixStack.pop()
# when st is an operator
else:
p1 = self.getPriority(st)
p2 = self.getPriority(postfixStack.peek())
while p1 <= p2:
postfix = postfix + postfixStack.pop() + ' '
p2 = self.getPriority(postfixStack.peek())
postfixStack.push(st)
if not postfixStack.isEmpty():
print("Invalid expression")
return None
return postfix
pass
def calculate(self):
# calculate the expression and return its value, if fails return None
if not isinstance(self.__expr, str) or len(self.__expr) <= 0:
print("Argument error in calculate")
return None
calcStack = Stack()
# convert an expression from infix to postfix
postfix = self._getPostfix(self.__expr)
if postfix is None:
return None
ls = postfix.split(' ')
ls.pop()
for st in ls:
# check if st is an float number
if self._isNumber(st):
calcStack.push(float(st))
# when st is an operator
else:
# pop two number from the stack
x = calcStack.pop()
y = calcStack.pop()
if st == '+':
calcStack.push(y + x)
elif st == '-':
calcStack.push(y - x)
elif st == '*':
calcStack.push(y * x)
elif st == '/' and x != 0:
calcStack.push(y / x)
elif st == '^':
calcStack.push(y ** x)
val = calcStack.pop()
if not calcStack.isEmpty():
print("Invalid expression")
return None
return val
pass

![Section 3: The AdvancedCalculator class
_isVariable(self, word)
Determines if the input is a valid variable name (see above for rules for names). The string
methods str.isalpha) and str.isalnum() could be helpful here.
Input (excluding self)
word The string to check if it is a valid variable name
str
Output
bool True if word is a variable name, False otherwise
_replaceVariables(self, expr)
Replaces all variables in the input expression with the current value of those variables saved
in self.states.
Input (excluding self)
expr The input expression that may contain variables
str
Output
The expression with all variables replaced with their values
None Nothing is returned if expression has invalid variables or uses a variable that has not
str
been defined
calculateExpressions(self)
Evaluates each expression saved in self.expressions. For each line, replace all variables in
the expression with their values, then use a Calculator object to evaluate the expression and
update self.states. This method returns a dictionary that shows the progression of the
calculations, with the key being the line evaluated and the value being the current state of
self.states after that line is evaluated. The dictionary must include a key named _return_' with
the return value as its value.
Hint: the str.split(sep) method can be helpful for separating lines, as well as separating the variable
from the expression (for the lines that are formatted as var = expr). The str.strip() method removes
the white space before and after a string. Don't forget dictionaries are mutable objects!
>> 'hi;there'.split(';')
['hi', 'there']
>>> 'hi=there'.split('=')
['hi', 'there']
>>>' hi
there
.strip()
' hi
there'
Output
The different states of self.states as the calculation progresses.
None Nothing is returned if there was an error replacing variables
dict](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F76de5ad5-a780-4d7f-a00a-85f0792f2226%2F7f8143c1-20b1-41aa-8d36-3dcd9140ba7b%2Fyw7z8r_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

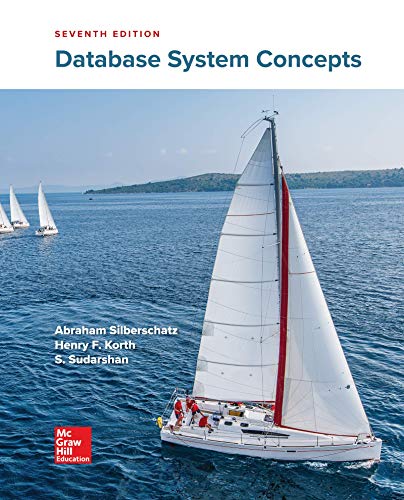
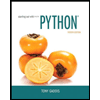
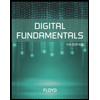
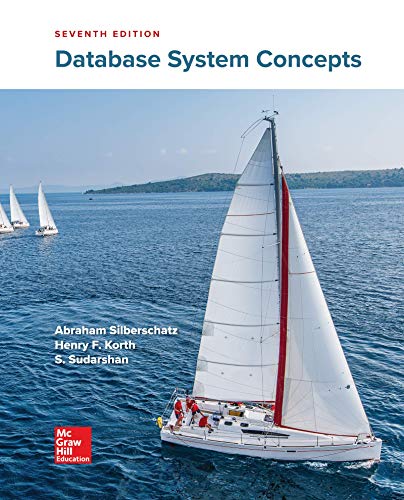
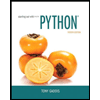
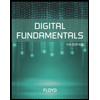
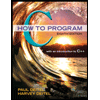
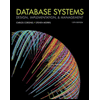
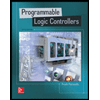