that (possibly new) base. The only Python int function you are allowed to use is int(s,b) where s is a string of digits and ba base. Here is a quick session to remind you of its function: 1 >> int ('101' ,2) 2 5 3 > int ('100' ,2) 4 4 5 > int ('1001',3) 6 28 Deliverables Problem 5 • Complete the functions make_number, convert, add and mul • You will need to use aside from % and // • The only Python function you can use is int(x,b) • Provide docstrings for the functions
that (possibly new) base. The only Python int function you are allowed to use is int(s,b) where s is a string of digits and ba base. Here is a quick session to remind you of its function: 1 >> int ('101' ,2) 2 5 3 > int ('100' ,2) 4 4 5 > int ('1001',3) 6 28 Deliverables Problem 5 • Complete the functions make_number, convert, add and mul • You will need to use aside from % and // • The only Python function you can use is int(x,b) • Provide docstrings for the functions
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![In this problem, you use a data structure that allows extended functionality with bases. For any sequence of digits \(d_n, d_{n-1} \cdots d_0\) in a base \(b\), we will use this structure:
- **Wild Number** ::= \([\langle \text{string} \rangle, b]\)
- \(\langle \text{string} \rangle ::= "d_n, d_{n-1}, \cdots, d_0"\)
where \(b\) is the base and the string is a string of digits. We will call our numbers wild numbers (WN). For example,
```
1 ['101', 2]
2 ['100', 2]
3 ['5', 10]
```
are three WNs. The first is in binary (it's equivalent to \(5_{10}\)), the second is binary (it’s equivalent to \(4_{10}\)) and the third is decimal 5. You will implement several functions whose use is shown here:
```
1 n1, n2 = 5, 4
2 base2, base10 = 2, 10
3 x1, y1 = make_number(n1, base2), make_number(n2, base2)
4 print(x1, y1)
5
6 print(convert(x1, base10))
7 print(add_(x1, y1, base10))
8 print(add_(x1, y1, base2))
9 print(convert(add_(x1, y1, base2), base10))
10 print(mul_(x1, y1, base2))
11 print(convert(mul_(x1, y1, base2), base10))
```
The output is:
```
1 ['101', 2] | ['100', 2]
2 ['5', 10]
3 ['9', 10]
4 ['1001', 2]
5 ['9', 10]
6 ['10100', 2]
7 ['20', 10]
```
The function **make_number(n, b)** takes an actual integer \(n\) (Python) and base \(b\) (integer greater than one) and creates a WN. The function **convert(WN, b)** converts an existing W](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8e2a772e-1ea3-4d63-a454-a2f39f414658%2F00bbe8c3-94f5-4f4a-9f65-2cd7b138321b%2Ftrnaec_processed.jpeg&w=3840&q=75)
Transcribed Image Text:In this problem, you use a data structure that allows extended functionality with bases. For any sequence of digits \(d_n, d_{n-1} \cdots d_0\) in a base \(b\), we will use this structure:
- **Wild Number** ::= \([\langle \text{string} \rangle, b]\)
- \(\langle \text{string} \rangle ::= "d_n, d_{n-1}, \cdots, d_0"\)
where \(b\) is the base and the string is a string of digits. We will call our numbers wild numbers (WN). For example,
```
1 ['101', 2]
2 ['100', 2]
3 ['5', 10]
```
are three WNs. The first is in binary (it's equivalent to \(5_{10}\)), the second is binary (it’s equivalent to \(4_{10}\)) and the third is decimal 5. You will implement several functions whose use is shown here:
```
1 n1, n2 = 5, 4
2 base2, base10 = 2, 10
3 x1, y1 = make_number(n1, base2), make_number(n2, base2)
4 print(x1, y1)
5
6 print(convert(x1, base10))
7 print(add_(x1, y1, base10))
8 print(add_(x1, y1, base2))
9 print(convert(add_(x1, y1, base2), base10))
10 print(mul_(x1, y1, base2))
11 print(convert(mul_(x1, y1, base2), base10))
```
The output is:
```
1 ['101', 2] | ['100', 2]
2 ['5', 10]
3 ['9', 10]
4 ['1001', 2]
5 ['9', 10]
6 ['10100', 2]
7 ['20', 10]
```
The function **make_number(n, b)** takes an actual integer \(n\) (Python) and base \(b\) (integer greater than one) and creates a WN. The function **convert(WN, b)** converts an existing W

Transcribed Image Text:**Python Integer Conversion Function**
In this session, we explore using the Python `int` function to convert a string of digits in a specific base into an integer. The syntax used is `int(s, b)`, where `s` is the string to be converted and `b` is the base.
**Examples:**
```python
>>> int('101', 2)
5
>>> int('100', 2)
4
>>> int('1001', 3)
28
```
**Deliverables for Problem 5:**
- Complete the functions `make_number`, `convert`, `add_`, and `mul_`.
- Utilize operations aside from `%` and `//`.
- The only Python function permitted is `int(x, b)`.
- Provide docstrings for the functions.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
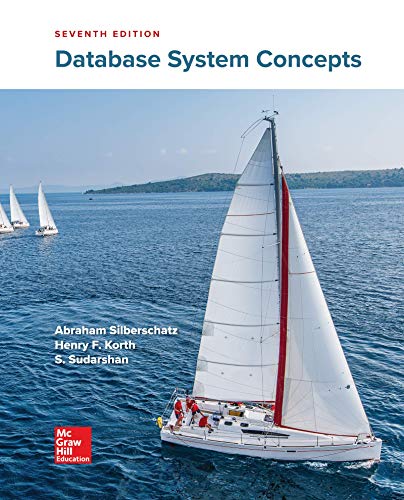
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
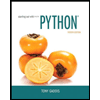
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
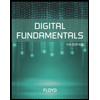
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
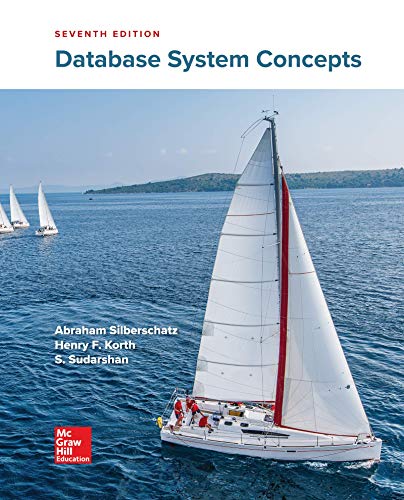
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
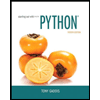
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
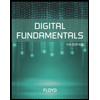
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
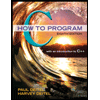
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
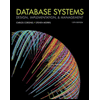
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
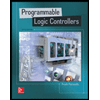
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education