Using C++ Write a program that uses for loops to perform the following steps
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
Using C++ Write a program that uses for loops to perform the following steps



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

How do I print the Uppercase letters without the commas?
Thank you. It also asks to 'output all uppercase letters' in case 1 and 2.
Test Case 1 has inputs
4
36
Test Case 2 has inputs
987
1001
The pictures I sent is the
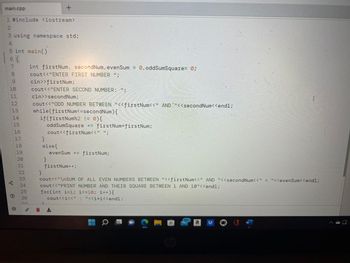
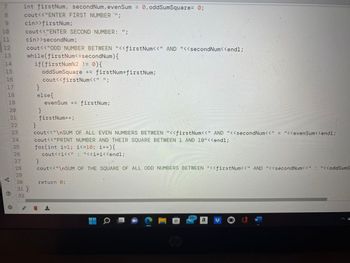
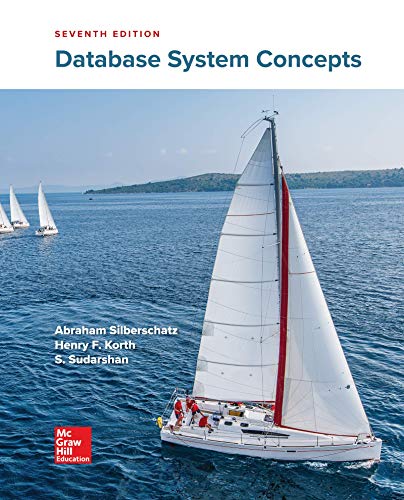
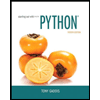
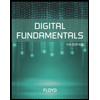
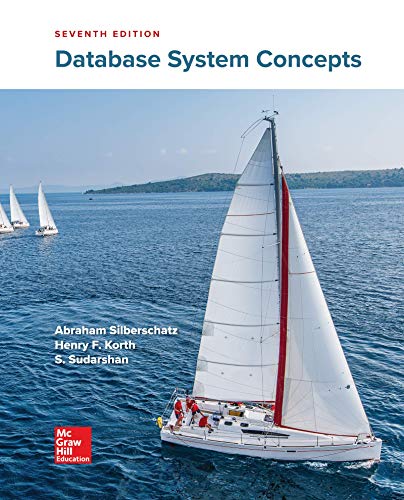
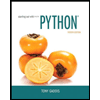
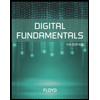
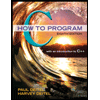
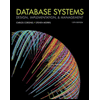
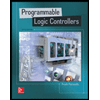