Read the specification for the Train class, its constructor, and the getUnicodeString method. 2. Create a JUnit test class for its constructor in the appropriate package based
I was told to
- Read the specification for the Train class, its constructor, and the getUnicodeString method.
2. Create a JUnit test class for its constructor in the appropriate package based
(You may have to build the class package yourself)
I don't understand how to make a Junit test class. My code is listed below.
/**
* Represents a train with some number of cars,
* that will be printed using Unicode characters.
*
* @author
* @version
*/
public class Train {
private int numCars;
private static String ENGINE = "?";
private static String CABOOSE = "?";
private static final String RAILCAR = "?";
/**
* Creates a train with the given number of cars.
* If the train has zero cars it is just an engine and caboose.
*
* @precondition numCars >= 0
* @postcondition getNumCars()==numCars
*
* @param numCars the number of cars (excepting engine and caboose)
* for the train
*/
public Train(int numCars) {
if (numCars < 0) {
throw new IllegalArgumentException("numCars should be > 0");
}
this.numCars = numCars;
}
/**
* Gets the number cars in the train,
* not including the engine and caboose.
*
* @return the number of cars
*/
public int getNumCars() {
return this.numCars;
}
/**
* Returns the total length of the train.
*
* @return the total length of the train, including the
* engine and caboose
*/
public int length() {
return this.numCars + 2;
}
/**
* Builds a unicode train consisting of an engine,
* followed by getNumCars() railcars, followed by a
* caboose. A train will always have at least an engine
* and a caboose.
*
* @return a Unicode string as described above.
*/
public String getUnicodeString() {
// replace the return statement with your solution
return null;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 4 images

Thank you for your response! I am supposed to use a for-loop to generate the railcars. Any idea how I can do this? Also the value of these Strings are all emojis even though they may show as questions marks. I hope this makes my question more clear!
private static String ENGINE = "?";
private static String CABOOSE = "?";
private static final String RAILCAR = "?";
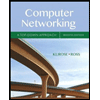
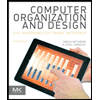
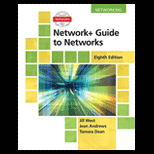
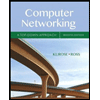
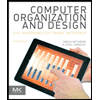
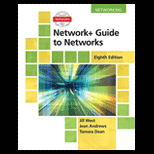
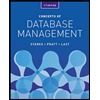
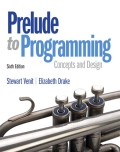
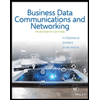