//********************************************************** // TEMPLATE FOR THE INVENTORY SYSTEM //********************************************************** //********************************************************** // CLASS NAME : Menu // DETAILS : THIS IS THE FIRST CLASS TO BE CALLED IN THE MAIN PROGRAM //********************************************************** class menu // DECIDE WHICH MEMBER BECOMES PUBLIC OR PRIVATE { void main_menu(void) ; void edit_menu(void) ; } ; //********************************************************** // CLASS NAME : Product // DETAILS : THIS CLASS CONTAINS FUNCTIONS/METHODS // RELATED TO PRODUCT ITEMS //********************************************************** class product // DECIDE WHICH MEMBER BECOMES PUBLIC OR PRIVATE { //minimum required functions void add_item(void) ; //this function stores the product record in product.txt void delete_item(void) ; //this function deletes a product record void modify_item(void) ; //this function updates a product record void display_item(void) ; //this function displays the product record // as a bonus, you may add a function to sort the records void purchase(void) ; //this function is for purchase transactions // and updates the product record int find_item(int) ; //this function finds a product record /* You can add features that would record every purchase made which will be stored in purchase_record.txt the file may contain info such as TransactionID Item No. Item Name Cost Purchased Total 001 023 Electrical Tape 25.00 3 75.00 001 015 1" PVC Pipe 205 2 410.00 002 053 LED Bulb 9W 110.00 2 220.00 You can also add a functon that can search and display a transaction record from purchase_record.txt using the TransactionID */ int itemcode ; char itemname[30] ; float itemprice ; //this is lacking, you are expected to add the necessary variables } ; //********************************************************** // THIS FUNCTION SHOWS THE MAIN MENU AND CALLS OTHER FUNCTIONS //********************************************************** void menu :: main_menu(void) { char ch ; while (1) { cout <<"1: PURCHASE PRODUCTS" ; cout <<"2: LIST OF PRODUCTS" ; cout <<"3: SEARCH PRODUCT" ; cout <<"4: EDIT PRODUCTS FILE" ; cout <<"5: TRANSACTIONS REPORT" ; cout <<"6: SEARCH TRANSACTION" ; cout <<"0: QUIT" ; cout <<"Enter Your Choice : " ; //your code } } //********************************************************** // THIS FUNCTION SHOWS THE EDIT MENU AND CALLS OTHER FUNCTIONS //********************************************************** void menu :: edit_menu(void) { char ch ; while (1) { cout <<"1: ADD PRODUCTS" ; cout <<"2: MODIFY PRODUCTS" ; cout <<"3: DELETE PRODUCTS" ; cout <<"0: EXIT" ; cout <<"Enter Choice : " ; //your code } } //*********************************************************** // REMEMBER TO IMPLEMEMNT THIS PROJECT USING SEPARATE COMPILATION // YOU ARE FREE TO DECIDE ON HOW TO SEPARATE YOUR CLASSES INTO // THEIR CORRESPONDING INTERFACE AND IMPLEMENTATION FILES // provide proper comments wherever applicable //***********************************************************
//**********************************************************
// TEMPLATE FOR THE INVENTORY SYSTEM
//**********************************************************
//**********************************************************
// CLASS NAME : Menu
// DETAILS : THIS IS THE FIRST CLASS TO BE CALLED IN THE MAIN PROGRAM
//**********************************************************
class menu // DECIDE WHICH MEMBER BECOMES PUBLIC OR PRIVATE
{
void main_menu(void) ;
void edit_menu(void) ;
} ;
//**********************************************************
// CLASS NAME : Product
// DETAILS : THIS CLASS CONTAINS FUNCTIONS/METHODS
// RELATED TO PRODUCT ITEMS
//**********************************************************
class product // DECIDE WHICH MEMBER BECOMES PUBLIC OR PRIVATE
{
//minimum required functions
void add_item(void) ; //this function stores the product record in product.txt
void delete_item(void) ; //this function deletes a product record
void modify_item(void) ; //this function updates a product record
void display_item(void) ; //this function displays the product record
// as a bonus, you may add a function to sort the records
void purchase(void) ; //this function is for purchase transactions
// and updates the product record
int find_item(int) ; //this function finds a product record
/*
You can add features that would record every purchase made
which will be stored in purchase_record.txt
the file may contain info such as
TransactionID Item No. Item Name Cost Purchased Total
001 023 Electrical Tape 25.00 3 75.00
001 015 1" PVC Pipe 205 2 410.00
002 053 LED Bulb 9W 110.00 2 220.00
You can also add a functon that can search and display a transaction record
from purchase_record.txt using the TransactionID
*/
int itemcode ;
char itemname[30] ;
float itemprice ;
//this is lacking, you are expected to add the necessary variables
} ;
//**********************************************************
// THIS FUNCTION SHOWS THE MAIN MENU AND CALLS OTHER FUNCTIONS
//**********************************************************
void menu :: main_menu(void)
{
char ch ;
while (1)
{
cout <<"1: PURCHASE PRODUCTS" ;
cout <<"2: LIST OF PRODUCTS" ;
cout <<"3: SEARCH PRODUCT" ;
cout <<"4: EDIT PRODUCTS FILE" ;
cout <<"5: TRANSACTIONS REPORT" ;
cout <<"6: SEARCH TRANSACTION" ;
cout <<"0: QUIT" ;
cout <<"Enter Your Choice : " ;
//your code
}
}
//**********************************************************
// THIS FUNCTION SHOWS THE EDIT MENU AND CALLS OTHER FUNCTIONS
//**********************************************************
void menu :: edit_menu(void)
{
char ch ;
while (1)
{
cout <<"1: ADD PRODUCTS" ;
cout <<"2: MODIFY PRODUCTS" ;
cout <<"3: DELETE PRODUCTS" ;
cout <<"0: EXIT" ;
cout <<"Enter Choice : " ;
//your code
}
}
//***********************************************************
// REMEMBER TO IMPLEMEMNT THIS PROJECT USING SEPARATE COMPILATION
// YOU ARE FREE TO DECIDE ON HOW TO SEPARATE YOUR CLASSES INTO
// THEIR CORRESPONDING INTERFACE AND IMPLEMENTATION FILES
// provide proper comments wherever applicable
//***********************************************************

Step by step
Solved in 2 steps with 9 images

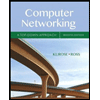
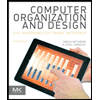
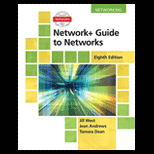
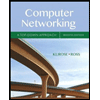
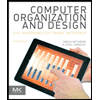
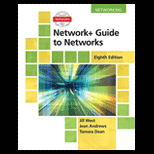
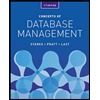
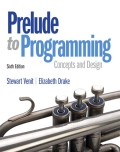
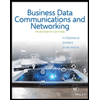