TASK 8. Repeat TASKs 4-7. Change Summation Integers problem to Finding the minimum problem. Make sure you properly wrote/updated all text messages, method names, and math calculations. Hint: You can use java.lang.Math.min() method. Example: System.out.printf("The min of the integers %4d and %4d and %4d is %7d\n", a, b, c, MinTest(a, b, c)); Submit the source code files (.java files) and the console output screenshots
TASK 8. Repeat TASKs 4-7. Change Summation Integers problem to Finding the minimum problem. Make sure you properly wrote/updated all text messages, method names, and math calculations. Hint: You can use java.lang.Math.min() method. Example: System.out.printf("The min of the integers %4d and %4d and %4d is %7d\n", a, b, c, MinTest(a, b, c)); Submit the source code files (.java files) and the console output screenshots
Chapter8: Advanced Method Concepts
Section: Chapter Questions
Problem 8RQ
Related questions
Question
TASK 8.
Repeat TASKs 4-7. Change Summation Integers problem to Finding the minimum
problem. Make sure you properly wrote/updated all text messages, method names,
and math calculations.
Hint: You can use java.lang.Math.min() method.
Example: System.out.printf("The min of the integers %4d and %4d and %4d
is %7d\n", a, b, c, MinTest(a, b, c));
Submit the source code files (.java files) and the console output screenshots
![## Java Program Code Explanation
### Code Example 1
```java
import java.util.Scanner;
public class ReportSum4 {
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
// Prompt the user to enter the first integer
System.out.println("Enter the value for 'a' and press Enter: ");
int a = sc.nextInt();
// Prompt the user to enter the second integer
System.out.println("Enter the value for 'b' and press Enter: ");
int b = sc.nextInt();
// Display the result
System.out.printf("Provided Integers: %4d and %4d, the total is %5d\n", a, b, sum(a, b));
}
public static int sum(int num1, int num2) {
return num1 + num2;
}
}
```
**Explanation:**
- The program initializes a scanner to take console input.
- It prompts the user to input two integers (`a` and `b`).
- It calculates the sum of the two integers using the `sum` method.
- Finally, it prints the result formatted to show the two integers and their sum.
### Code Example 2
```java
import java.util.Scanner;
public class ReportSum5 {
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
// Prompt user to enter the number of integers
System.out.println("Enter the number of integers you will provide: ");
int n = sc.nextInt();
// Prompt the user to enter the first integer
System.out.println("Enter the value for 'a' and press Enter: ");
int a = sc.nextInt();
// Prompt the user to enter the second integer
System.out.println("Enter the value for 'b' and press Enter: ");
int b = sc.nextInt();
int c = 0;
// If count is 3, prompt user to enter the third integer
if (n == 3) {
System.out.println("Enter the value for 'c' and press Enter: ");
c = sc.nextInt();
System.out.printf("Provided Integers: %3d and %3d and %3d, the total is %5d\n", a, b, c, sum(a, b, c));
} else {
System.out.printf](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe4e72d86-d216-4977-8ee6-fe536869a207%2Fee12f55e-1a4b-4824-b461-c4f86ec9d914%2Fv4q4bpu_processed.jpeg&w=3840&q=75)
Transcribed Image Text:## Java Program Code Explanation
### Code Example 1
```java
import java.util.Scanner;
public class ReportSum4 {
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
// Prompt the user to enter the first integer
System.out.println("Enter the value for 'a' and press Enter: ");
int a = sc.nextInt();
// Prompt the user to enter the second integer
System.out.println("Enter the value for 'b' and press Enter: ");
int b = sc.nextInt();
// Display the result
System.out.printf("Provided Integers: %4d and %4d, the total is %5d\n", a, b, sum(a, b));
}
public static int sum(int num1, int num2) {
return num1 + num2;
}
}
```
**Explanation:**
- The program initializes a scanner to take console input.
- It prompts the user to input two integers (`a` and `b`).
- It calculates the sum of the two integers using the `sum` method.
- Finally, it prints the result formatted to show the two integers and their sum.
### Code Example 2
```java
import java.util.Scanner;
public class ReportSum5 {
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
// Prompt user to enter the number of integers
System.out.println("Enter the number of integers you will provide: ");
int n = sc.nextInt();
// Prompt the user to enter the first integer
System.out.println("Enter the value for 'a' and press Enter: ");
int a = sc.nextInt();
// Prompt the user to enter the second integer
System.out.println("Enter the value for 'b' and press Enter: ");
int b = sc.nextInt();
int c = 0;
// If count is 3, prompt user to enter the third integer
if (n == 3) {
System.out.println("Enter the value for 'c' and press Enter: ");
c = sc.nextInt();
System.out.printf("Provided Integers: %3d and %3d and %3d, the total is %5d\n", a, b, c, sum(a, b, c));
} else {
System.out.printf
![### Task 6 & 7: Understanding Overloaded Methods in Java
This example demonstrates method overloading and the use of variable arguments in Java.
#### Code Explanation:
1. **Method Overloading:**
- The code defines three versions of the `sum` method, each taking a different number of integer arguments (2, 3, and 4 integers, respectively).
```java
public static int sum(int num1, int num2) {
return num1 + num2;
}
public static int sum(int num1, int num2, int num3) {
return num1 + num2 + num3;
}
public static int sum(int num1, int num2, int num3, int num4) {
return num1 + num2 + num3 + num4;
}
```
2. **Main Method:**
- The `main` method in the `chapter7` class calls the `sum` method with various sets of integer arguments.
```java
public class chapter7 {
public static void main(String[] args) {
sum(34, 3);
sum(34, 3, 3);
sum(34, 3, 3, 2);
sum(34, 3, 3, 2, 56);
sum(34, 3, 3, 2, 56, 5);
sum(34, 3, 3, 2, 56, 55, 990);
}
}
```
3. **Variable Arguments:**
- There is an additional `sum` method using variable arguments, allowing any number of integers as inputs. It sums all the provided numbers and prints the result.
```java
public static void sum(int... numbers) {
int sum = 0;
for (int i : numbers)
sum += i;
System.out.println("The sum of numbers is " + sum);
}
```
#### Key Concepts:
- **Method Overloading:** Defining multiple methods with the same name but different parameter lists. It allows methods to handle different types or amounts of data.
- **Variable Arguments (Varargs):** Allows a method to accept zero or multiple arguments. It is represented by three dots `(...)` after the data type, enabling the](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe4e72d86-d216-4977-8ee6-fe536869a207%2Fee12f55e-1a4b-4824-b461-c4f86ec9d914%2F38h4p4o_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### Task 6 & 7: Understanding Overloaded Methods in Java
This example demonstrates method overloading and the use of variable arguments in Java.
#### Code Explanation:
1. **Method Overloading:**
- The code defines three versions of the `sum` method, each taking a different number of integer arguments (2, 3, and 4 integers, respectively).
```java
public static int sum(int num1, int num2) {
return num1 + num2;
}
public static int sum(int num1, int num2, int num3) {
return num1 + num2 + num3;
}
public static int sum(int num1, int num2, int num3, int num4) {
return num1 + num2 + num3 + num4;
}
```
2. **Main Method:**
- The `main` method in the `chapter7` class calls the `sum` method with various sets of integer arguments.
```java
public class chapter7 {
public static void main(String[] args) {
sum(34, 3);
sum(34, 3, 3);
sum(34, 3, 3, 2);
sum(34, 3, 3, 2, 56);
sum(34, 3, 3, 2, 56, 5);
sum(34, 3, 3, 2, 56, 55, 990);
}
}
```
3. **Variable Arguments:**
- There is an additional `sum` method using variable arguments, allowing any number of integers as inputs. It sums all the provided numbers and prints the result.
```java
public static void sum(int... numbers) {
int sum = 0;
for (int i : numbers)
sum += i;
System.out.println("The sum of numbers is " + sum);
}
```
#### Key Concepts:
- **Method Overloading:** Defining multiple methods with the same name but different parameter lists. It allows methods to handle different types or amounts of data.
- **Variable Arguments (Varargs):** Allows a method to accept zero or multiple arguments. It is represented by three dots `(...)` after the data type, enabling the
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
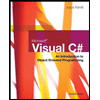
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
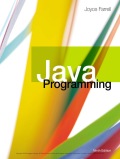
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
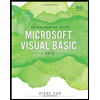
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
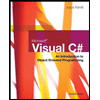
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
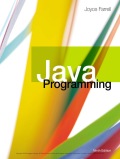
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
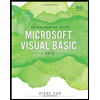
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
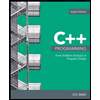
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
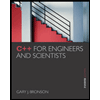
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage