Task 3- Student Information Unpacking (Topics: Nested collections, flow-control, multi-file, strings) Requires: utilities.py-> variables students and studentsInfo Task 3 is to print out a list of students and their information. This information is stored in two variables (a list and a dictionary of lists). The data is organized as shown in the diagram below. Specifically, the variable students is a list 47 strings of student names; the variable studentsInfo is a dictionary where the keys indicate information about the students. Each key is associated with a list of integers that indexes into the students list variable. The keys in the dictionary indicate the following information: 'Year 1' to 'Year 4' is what year a student is studying. For example, 'Sasha' is a 'Year 2' student (see below). Keys 'CS','DM','BZ','SE' indicate the students' program of study (e.g., 'CS' is comp sci, 'DM' is digital media, 'BZ' is business, and 'SE' is software engineering). For example, 'Solar' is a 'CS' student. Keys 'On Campus' and 'Off Campus' indicate the students' living arrangements. For example, 'Mahmoud' lives 'On Campus'. index 1 3 4 5 45 46 students - ['Juhong', 'Sasha', 'solar', 'Ali', 'Amy', 'Gopi', -, 'Mahmoud', 'Rutha'] studentsInfo - { "Year 1': [42, 24, 12, 37, 35, 23, 32, 5, 34, 19], "Year 2': [1, 28, 36, 46, 30, 15, 6, 33, 13, 7, 18, 44], "Year 3': [26, 11, 21, 3, 2, 14, 31, 27], *Year 4': [25, 4, 20, е, 16, 17, 40, 39, 29, 22, 41, 9, 8, 10, 38, 43, 45], "CS': [24, 1, 3, 12, 2, зе, 23, 34, з, 45, 6, 22, 39, 11, 14, 28, 15, 26, 21, 7], "DM': [44, 18, 16, 40, 29, 13, 46], "BZ': [10, 5, 9, 31, 41, 20, 8, 25, 17, 42, 35, 43, 38, e], "SE': [4, 19, 27, 32, 36, 37], "On Campus': [24, 13, 6, 3, 22, 4, 23, 12, 15, m, 33, 40, 43, 35, 8, 45], 'Off Campus': [30, 1, 37, 36, 41, 16, 9, 25, 29, 17, e, 18, 31, 44, 5, 32, 2, 20, 28] Task 3 should behave as follows: 1) Use the two variables above to print out each student's information sorted by the student's name (see below). 2) Format the output for each student as follows: Vladen (Year 3) Program: CS Housing: On Campus
Task 3- Student Information Unpacking (Topics: Nested collections, flow-control, multi-file, strings) Requires: utilities.py-> variables students and studentsInfo Task 3 is to print out a list of students and their information. This information is stored in two variables (a list and a dictionary of lists). The data is organized as shown in the diagram below. Specifically, the variable students is a list 47 strings of student names; the variable studentsInfo is a dictionary where the keys indicate information about the students. Each key is associated with a list of integers that indexes into the students list variable. The keys in the dictionary indicate the following information: 'Year 1' to 'Year 4' is what year a student is studying. For example, 'Sasha' is a 'Year 2' student (see below). Keys 'CS','DM','BZ','SE' indicate the students' program of study (e.g., 'CS' is comp sci, 'DM' is digital media, 'BZ' is business, and 'SE' is software engineering). For example, 'Solar' is a 'CS' student. Keys 'On Campus' and 'Off Campus' indicate the students' living arrangements. For example, 'Mahmoud' lives 'On Campus'. index 1 3 4 5 45 46 students - ['Juhong', 'Sasha', 'solar', 'Ali', 'Amy', 'Gopi', -, 'Mahmoud', 'Rutha'] studentsInfo - { "Year 1': [42, 24, 12, 37, 35, 23, 32, 5, 34, 19], "Year 2': [1, 28, 36, 46, 30, 15, 6, 33, 13, 7, 18, 44], "Year 3': [26, 11, 21, 3, 2, 14, 31, 27], *Year 4': [25, 4, 20, е, 16, 17, 40, 39, 29, 22, 41, 9, 8, 10, 38, 43, 45], "CS': [24, 1, 3, 12, 2, зе, 23, 34, з, 45, 6, 22, 39, 11, 14, 28, 15, 26, 21, 7], "DM': [44, 18, 16, 40, 29, 13, 46], "BZ': [10, 5, 9, 31, 41, 20, 8, 25, 17, 42, 35, 43, 38, e], "SE': [4, 19, 27, 32, 36, 37], "On Campus': [24, 13, 6, 3, 22, 4, 23, 12, 15, m, 33, 40, 43, 35, 8, 45], 'Off Campus': [30, 1, 37, 36, 41, 16, 9, 25, 29, 17, e, 18, 31, 44, 5, 32, 2, 20, 28] Task 3 should behave as follows: 1) Use the two variables above to print out each student's information sorted by the student's name (see below). 2) Format the output for each student as follows: Vladen (Year 3) Program: CS Housing: On Campus
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
This should be in Python
Here is a pastebin link to all the students info and names -> https://pastebin.com/su38xFbQ
![Task 3- Student Information Unpacking (Topics: Nested collections, flow-control, multi-file, strings)
Requires: utilities.py -> variables students and studentsInfo
Task 3 is to print out a list of students and their information. This information is stored in two variables (a list and a
dictionary of lists). The data is organized as shown in the diagram below. Specifically, the variable students is a list 47
strings of student names; the variable studentsInfo is a dictionary where the keys indicate information about the
students. Each key is associated with a list of integers that indexes into the students list variable.
The keys in the dictionary indicate the following information: 'Year 1' to 'Year 4' is what year a student is
studying. For example, 'Sasha' is a 'Year 2' student (see below). Keys 'CS', 'DM','BZ','SE' indicate the
students' program of study (e.g., 'CS' is comp sci, 'DM' is digital media, 'BZ' is business, and ' SE' is software
engineering). For example, 'Solar' is a 'CS' student. Keys 'On Campus ' and 'Off Campus' indicate the
students' living arrangements. For example, 'Mahmoud' lives 'On Campus'.
index
1
2
3
4
45
46
students - ['Juhong', 'Sasha', 'Solar', 'Ali', 'Amy', 'Gopi', ., 'Mahmoud', 'Rutha']
studentsInfo = {
'Year 1': [42, 24, 12, 37, 35, 23, 32, 5, 34, 19],
'Year 2': [1, 28, 36, 46, 30, 15, 6, 33, 13, 7, 18, 44],
"Year 3': [26, 11, 21, 3, 2, 14, 31, 27],
'Year 4': [25, 4, 20, e, 16, 17, 40, 39, 29, 22, 41, 9, 8, 10, 38, 43, 45],
'cs': [24, 1, 33, 12, 2, 30, 23, 34, 3, 45, 6, 22, 39, 11, 14, 28, 15, 26, 21, 7],
'DM': [44, 18, 16, 40, 29, 13, 46],
"BZ': [10, 5, 9, 31, 41, 20, 8, 25, 17, 42, 35, 43, 38, e],
"SE': [4, 19, 27, 32, 36, 37],
"On Campus': [24, 13, 6, 3, 22, 4, 23, 12, 15,
'off Campus': [30, 1, 37, 36, 41, 16, 9, 25, 29, 17, e, 18, 31, 44, 5, 32, 2, 20, 28]
33, 40, 43, 35, 8, 45],
***
Task 3 should behave as follows:
1) Use the two variables above to print out each student's information sorted by the student's name (see below).
2) Format the output for each student as follows:
Vladen (Year 3) Program: CS Housing: On Campus
ΛΛΛΛΛΛΛΛΛ
There should be ten characters for each name. If a name has less than ten characters, you'll need to pad it with leading
spaces. Using ten characters for the name ensures the final output of each student aligns, as shown below.
Example of task 3 running -- see video link to see an example of the full list of names being printed.
Abisai (Year 4) Program: BZ Housing: On Campus
Ajay (Year 2) Program: SE Housing: Off Campus
Alexi (Year 4) Program: DM Housing: On Campus
Ali (Year 3) Program: CS Housing: On Campus
Amy (Year 4) Program: SE Housing: On Campus
..
Ursula (Year 2) Program: CS Housing: Off Campus
Vladen (Year 3) Program: CS Housing: On Campus
Wen (Year 1) Program: SE Housing: Off Campus
Program: CS Housing: On Campus
Weng-Fai (Year
Yasu (Year 4) Program: CS Housing: On Campus
Yuki (Year 4) Program: BZ Housing: On Campus
Yunjo (Year 2) Program: DM Housing: Off Campus](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fbeb43acd-4bfb-4c49-84a8-7d6db7b72498%2F53c47e03-ba7e-4f8d-b4fe-b2b98c636312%2F1wfe47_processed.png&w=3840&q=75)
Transcribed Image Text:Task 3- Student Information Unpacking (Topics: Nested collections, flow-control, multi-file, strings)
Requires: utilities.py -> variables students and studentsInfo
Task 3 is to print out a list of students and their information. This information is stored in two variables (a list and a
dictionary of lists). The data is organized as shown in the diagram below. Specifically, the variable students is a list 47
strings of student names; the variable studentsInfo is a dictionary where the keys indicate information about the
students. Each key is associated with a list of integers that indexes into the students list variable.
The keys in the dictionary indicate the following information: 'Year 1' to 'Year 4' is what year a student is
studying. For example, 'Sasha' is a 'Year 2' student (see below). Keys 'CS', 'DM','BZ','SE' indicate the
students' program of study (e.g., 'CS' is comp sci, 'DM' is digital media, 'BZ' is business, and ' SE' is software
engineering). For example, 'Solar' is a 'CS' student. Keys 'On Campus ' and 'Off Campus' indicate the
students' living arrangements. For example, 'Mahmoud' lives 'On Campus'.
index
1
2
3
4
45
46
students - ['Juhong', 'Sasha', 'Solar', 'Ali', 'Amy', 'Gopi', ., 'Mahmoud', 'Rutha']
studentsInfo = {
'Year 1': [42, 24, 12, 37, 35, 23, 32, 5, 34, 19],
'Year 2': [1, 28, 36, 46, 30, 15, 6, 33, 13, 7, 18, 44],
"Year 3': [26, 11, 21, 3, 2, 14, 31, 27],
'Year 4': [25, 4, 20, e, 16, 17, 40, 39, 29, 22, 41, 9, 8, 10, 38, 43, 45],
'cs': [24, 1, 33, 12, 2, 30, 23, 34, 3, 45, 6, 22, 39, 11, 14, 28, 15, 26, 21, 7],
'DM': [44, 18, 16, 40, 29, 13, 46],
"BZ': [10, 5, 9, 31, 41, 20, 8, 25, 17, 42, 35, 43, 38, e],
"SE': [4, 19, 27, 32, 36, 37],
"On Campus': [24, 13, 6, 3, 22, 4, 23, 12, 15,
'off Campus': [30, 1, 37, 36, 41, 16, 9, 25, 29, 17, e, 18, 31, 44, 5, 32, 2, 20, 28]
33, 40, 43, 35, 8, 45],
***
Task 3 should behave as follows:
1) Use the two variables above to print out each student's information sorted by the student's name (see below).
2) Format the output for each student as follows:
Vladen (Year 3) Program: CS Housing: On Campus
ΛΛΛΛΛΛΛΛΛ
There should be ten characters for each name. If a name has less than ten characters, you'll need to pad it with leading
spaces. Using ten characters for the name ensures the final output of each student aligns, as shown below.
Example of task 3 running -- see video link to see an example of the full list of names being printed.
Abisai (Year 4) Program: BZ Housing: On Campus
Ajay (Year 2) Program: SE Housing: Off Campus
Alexi (Year 4) Program: DM Housing: On Campus
Ali (Year 3) Program: CS Housing: On Campus
Amy (Year 4) Program: SE Housing: On Campus
..
Ursula (Year 2) Program: CS Housing: Off Campus
Vladen (Year 3) Program: CS Housing: On Campus
Wen (Year 1) Program: SE Housing: Off Campus
Program: CS Housing: On Campus
Weng-Fai (Year
Yasu (Year 4) Program: CS Housing: On Campus
Yuki (Year 4) Program: BZ Housing: On Campus
Yunjo (Year 2) Program: DM Housing: Off Campus
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 6 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
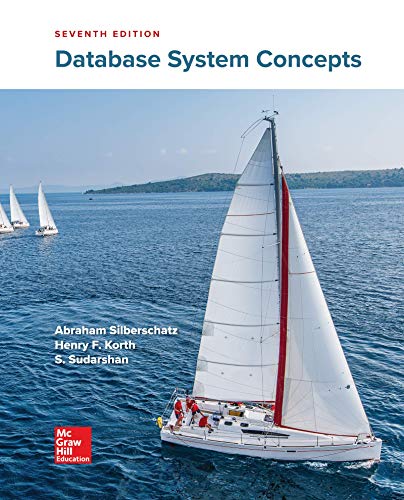
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
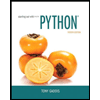
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
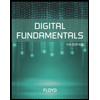
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
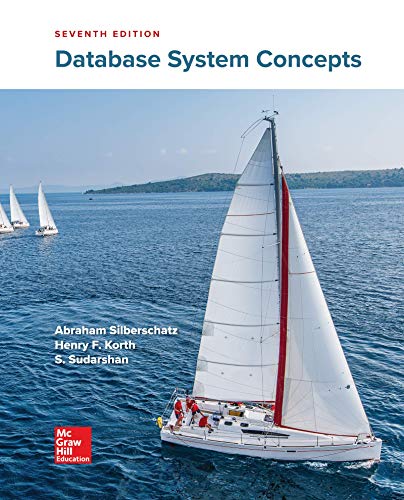
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
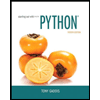
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
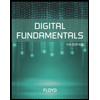
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
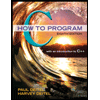
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
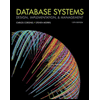
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
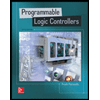
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education