Task 2: Write a program to implement Binary search. Count numbers of comparison you have made to search particular element.
Q: Quick sort the given data (if the Partion function is called once). A[ ] = {30, 35, 50, 45, 15,…
A: One of the divide and conquer algorithms is Quick Sort. It selects an element as a pivot initially…
Q: Write a program to test the sequential search algorithm that you wrote of this chapter. Use either…
A: Program code: //include the required header files#include<iostream>using namespace…
Q: solve in python coding 3. Selection Sort • The idea in a selection sort is that you locate the…
A: CODE: def index(array,step,size,min_index): for i in range(step + 1, size): if array[i]…
Q: f an array is sorted in this order, the values are stored from highest to lowest.a. asymptoticb.…
A: answer is d. descending wrong answers asymptotic logarithmic ascending
Q: Exercise 1: In this problem, we would like to implement a variation of the Bubble Sort algorithm.…
A: Bubble Sort Algorithm Start the program Call the sort function for given array. Check the element…
Q: Write a function checks if an array has any duplicates by taking each element, then iterating over…
A: The code below examines each element in turn before iterating over the entire array to see if the…
Q: please code in python Forbidden concepts: arrays/lists (data structures), recursion, custom classes…
A: function main(): Start Read k for row = 1 to k+1 follow step 4 to 5 for column = 1 to k+1 follow…
Q: Complete largest_at_position with the help of longest_chain. Can't use recursion, or any imports
A: There is a given binary matrix, we need to find if there exists any rectangle or square in the given…
Q: #### Part 1 Write a Python function (`insertion_sort`) that implements the insertion sort…
A: Insertion sort1def insertion_sort(values): 2 comparisons = 0 3 for i in range(1, len(values)):…
Q: Given an ordered array of n elements, partition the elements into two arrays such that the elements…
A: We have an ordered array of n elements. We need to write a program that will make a partition of…
Q: Assume you are implementing an application where a large set of data elements is kept in a sorted…
A: Given:
Q: Given the following array declaration: int list[] = {6, 1, 9, 4, 0, 7, 5}; When performing a linear…
A: We need 4 comparisons to do while performing linear search approach to find that whether 0 I present…
Q: 1. Initialize i = ip and count = 0 2. Use an array „dest‟ to hold the required substring 3. Repeat…
A: 1. Initialize i = ip and count = 02. Use an array „dest‟ to hold the required substring3. Repeat…
Q: Define a function named create_words_dictionary (words_list) which takes a list of words as a…
A: HI THEREI AM ADDING ANSWER BELOWPLEASE GO THROUGH ITTHANK YOU
Q: Complete the binary search function below using while loop. int search(int a[], int t,…
A: GIVEN: Complete the binary search function below using while loop. int search(int…
Q: Write a loop that sets newScores to oldScores shifted once left, with element 0 copied to the end.…
A: This is a C++ programing element involving basic knowledge of loop and swapping of elements. This…
Q: Python Write a program that automatically generates a Python list of 25 random integers between…
A: Importing library to generate random number. Using function randInt() to generate integer random…
Q: Declare two different arrays dynamically having a user input for the elements. Store the names in it…
A: Here I have first asked the user to enter the size of the array and then using the new operator I…
Q: Description Write a program that asks the user for an integer value greater than zero. It will…
A: Here I have created the function named printDown and printUp(). In the printDown() function I have…
Q: * allSame returns true if all of the elements in list have the same value. * allSame returns false…
A: In this question we have to code for the given problem statement Let's code and hope this helps if…
Q: Complete the binary search function below using while loop. int search(int a[, int t, int 1, int r)0
A: Here I written C program for given problem with function below. I hope you like it.
Q: def remove_after_kth(items, k=1): Given a list of items, some of which may be duplicated, create…
A: In this program, I have created a function remove_after_kth which will print the desired list and…
Q: ents of A. What type of sorting is this? Write the algorithm and also mention the name of this…
A: Q: Suppose you are given an array A of n elements. Your task is to sort n numbers stored in array…
Q: Prompt: In Python language, wrte a function that applies the logistic sigmoid function to all…
A: Sigmoid activation function is used to reduce the loss during the time of training because it…
Q: using python language • Create the following list of numbers: [3,7,5,2,9,7] • Write a while loop…
A: Algorithm: 1. Start 2. Initialize list 3. Initialize i to 0 4. Check while loop condition 5. If…
Q: inary search. The ordered array produced by the sort should be passed to the search routine which…
A: EXPLANATION First, read the inputs and store them into the array. After that read a number to be…
Q: Design a function: int search (float a[ ], int n, float x); The function searches in array a of size…
A: The array is a linear data structure that stores elements of the same data type. The size of the…
Q: Python Write a program that allows the user to add, view, and delete names in an array list. The…
A: 1. Initialize Names List: - Create an empty list called `names` to store names.2. Main Program…
Q: 1. You have an array of 4 ints which is sorted from lowest to highest. You also have a function…
A: The bubble sort works by comparing each item in the list with the item next to it, and swapping them…
Q: 6. Import Module random, create a list of integers from 1 to 45, mix up the ordering of the list,…
A: Note: The program has been provided in python programming language. Thank you. The random.choices()…
Q: Tracey wrote the definition of a function, remove_last (phrase), which takes in a string phrase,…
A: PYTHON INTRODUCTION :- Python is a programming language that has a design philosophy that emphasizes…
Q: Write the code to determine if an item in in the array.
A: Approach: Here, we use a linear search that compares an item to be searched with each array element.…
Q: Write a program that first reads in the name of an input file and then reads the input file using…
A: def read_file(filename): file = None # open file try: file = open(filename) except: print("Unable…
Q: Creat a void fuction void myUpdate(string dictionary[], int wordCount, string oldWord, string…
A: I have provided the working code, and output. Since no language was mentioned in question, I have…
Q: Create a partially-full array that can hold up to 1000 (x,y) coordinate pairs. In order to do this,…
A: Actually, array is a collection of elements.
Q: Assume that empName and empID are two parallel arrays of size numEmp that hold employee data. Write…
A: Algorithm: 1. empID[] //containing the employee ID 2. empName[] //containing the names of…
Q: (Linear Search) Often, we are given a list of unsorted numbers and we would like to search for a…
A: NOTE - I have used Python programming language. Here I have created a function named lin_search().…
Q: Binary search can only be performed on a/an ………… Sorted array/list Random
A: Binary Search: Search a sorted array by repeatedly dividing the search interval in half. Begin with…
Q: Search of Strings 1. Write a version of the selection sort algorithm presented in the unit, which is…
A: #include <iostream>#include <vector>using namespace std; void…
Q: 3. Selection Sort • The idea in a selection sort is that you locate the largest item out of a set of…
A: Selection sort is a simple sorting algorithm. This sort algorithm is an in-place comparison-based…


Step by step
Solved in 3 steps with 1 images

- Question 1 Create a program which will ask the user to enter a list of names and provide a poker tournament order. It should do the following: Ask the user how many people are playing Ask the user to enter each name Print out a sorted list of names Print out a randomized list of names to determine who sits where To store the players, use a vector of strings, e.g. vector<string> players. Use the builtin sort and random shuffle (or shuffle) algorithms. Use range-based for loops to print out your lists.***Please help to write unique program do not copy an paste from another source*** Write a sub that creates an array day of size 7 and then populates it with the days of the week (Monday, Tuesday, ..., Sunday). Write a for loop for this array to write its content on to the first Excel sheet in column A. Write another sub that creates an array day(). No size should be mentioned. Redefine the array one size at a time to store the day of the week and repeat i for the 7 days. Now print the values in the array using a for loop to column B. Write a third sub that creates an array day(). No size should be mentioned. Redefine the array one size at a time. But use Redim Preserve keyword to store the day of the week and repeat i for the 7 days. Now print the values in the array using a for loop to column C. Submit your xlsm code on blackboard in a single file.C program
- Prob 4 Given a list of x objects, create a program that performs the following operation: -Get the first and last object and place them both at the start of the list. Example: QWERT becomes QTWER Find the worst case time complexity if the solution will be implemented using an array. Give both the EQUATION AND THE BIG-O NOTATION.Prompt: In Python language, write a function that applies the logistic sigmoid function to all elements of a NumPy array. Code: import numpy as np import math import matplotlib.pyplot as plt import pandas as pd def sigmoid(inputArray): modifiedArray = np.zeros(len(inputArray)) #YOUR CODE HERE: return(modifiedArray) def test(): inputs = np.arange(-100, 100, 0.5) outputs = sigmoid(inputs) plt.figure(1) plt.plot(inputs) plt.title('Input') plt.xlabel('Index') plt.ylabel('Value') plt.show() plt.figure(2) plt.plot(outputs,'Black') plt.title('Output') plt.xlabel('Index') plt.ylabel('Value') plt.show() test()Homework 7: Merging two sorted arrays Due date: Saturday March 18. Objectives: Learn to implement algorithms that work with multiple arrays. Understand the Merging algorithm. IMPORTANT. Carefully study Lecture 1 from Week 8 on D2L before you start on this. Sometimes we need to combine the values in two sorted sequences to produce a larger sorted sequence. This process is called Merging. As an example, if the first sequence (S1)contains the numbers 2 3 4 4 5 9 and the second sequence (S2) contains the numbers 1 4 5 8 9 11 12 13 17 the output sequence will contain 1 2 3 4 4 4 5 5 8 9 9 11 12 13 17 Each sequence can be stored in an array. The strategy is as follows: Start by comparing the first items in the two input arrays. Write the smaller number to the output array, and move to the the next item in that array. This is repeated until we reach the end of any one of the input arrays; thereafter, we simply copy all the numbers from the other input array to the output array. (In the…
- 01. ""Implementation of the Misra-Gries algorithm.Given a list of items and a value k, it returns the every item in the listthat appears at least n/k times, where n is the length of the array By default, k is set to 2, solving the majority problem. For the majority problem, this algorithm only guarantees that if there isan element that appears more than n/2 times, it will be outputed. If thereis no such element, any arbitrary element is returned by the algorithm.Therefore, we need to iterate through again at the end. But since we have filtredout the suspects, the memory complexity is significantly lower thanit would be to create counter for every element in the list. For example:Input misras_gries([1,4,4,4,5,4,4])Output {'4':5}Input misras_gries([0,0,0,1,1,1,1])Output {'1':4}Input misras_gries([0,0,0,0,1,1,1,2,2],3)Output {'0':4,'1':3}Input misras_gries([0,0,0,1,1,1]Output None. """Imagine you want to use insertion sort for sorting a deck of cards, where the suits are ordered [Clubs, Spades, Diamonds, Hearts]. Thus, all the clubs will be ordered [Ace, 1, 2.., Queen, King], then all the Spades, etc. Create the pseudocode for this problem*
- KEYWORDS: array, for, nested loops. LAB EXERCISE: Program 1: Write a program for implementation of Binary search using function. c\Users\UmarDesktop\cppproj\Debu.oe Enter 5 Nunbers in ascending order : c\Users\Umar\Desktop\cppproj\Debu o O Enter 5 Nunbers in ascending order : 45 67 88 65 Enter iten to be searched : 12 Elenent not found Press any key to continue Enter iten to be searched : Elenent found at index - 1 Press any key to continue Sample Output: Program 1struct grade { char id[10]; int mark; }; Using the student structure given above, create an array of size 5 students. Then write a complete C program to sort the students array based on the students rank. Use the following sorting techniques in your code. Don’t forget to print initial array and final (sorted) array. a. Insertion sort b. Merge sort c. Quick sortRewrite the following loop so it uses pointer notation (with the indirection operator) instead of subscript notation. for (int x = 0; x < 100; x++) cout << array[x] << endl;
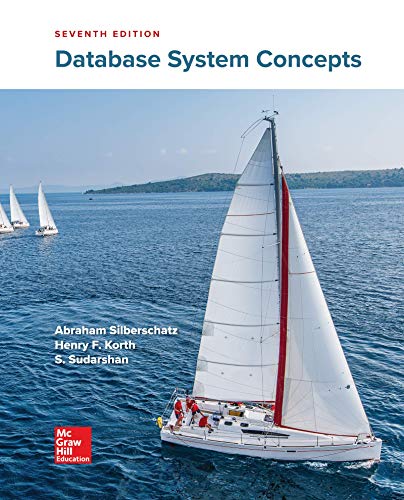
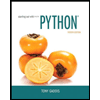
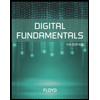
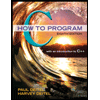
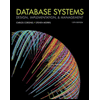
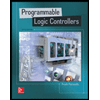
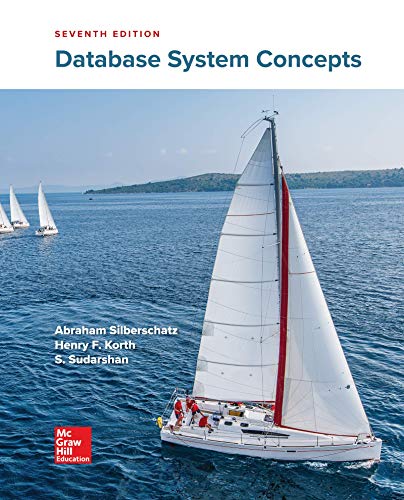
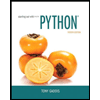
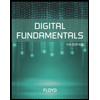
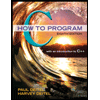
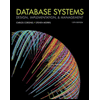
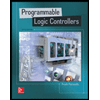