Task 1: Write down a complete C/C++ program to test your linear linked list implementation. Additionally, write another function which will be used to list the linked list content. Complete your implementation using the following code: header = insertBack(header,2); header = insertBack(header,4); header = insertBack(header,6); DisplayList(header); header = insertFront(header,1); DisplayList(header); insertAfter(header->next->next,5); DisplayList(header); header = deleteFront(header); DisplayList(header); header = deleteBack(header); DisplayList(header); deleteAfter(header->next); DisplayList(header); Task 2: Write a function that moves a node forward in the given linked list. Take the pointer of the node to be moved as a parameter. void moveforwardlist (struct node *, struct node *); Task 3: Write a function that moves a node backward in the given linked list. Take the pointer of the node to be moved as a parameter. void movebackwardlist (struct node *, struct node *); Task 4: Write a function that loads the linked list with the given values of an array. Consider array and its size as input parameter to the function. void loadlist (struct node *, int [ ], int );
Task 1: Write down a complete C/C++ program to test your linear linked list implementation. Additionally, write another function which will be used to list the linked list content. Complete your implementation using the following code:
header = insertBack(header,2);
header = insertBack(header,4);
header = insertBack(header,6);
DisplayList(header);
header = insertFront(header,1);
DisplayList(header);
insertAfter(header->next->next,5);
DisplayList(header);
header = deleteFront(header);
DisplayList(header);
header = deleteBack(header);
DisplayList(header);
deleteAfter(header->next);
DisplayList(header);
Task 2: Write a function that moves a node forward in the given linked list. Take the pointer of the node to be moved as a parameter.
void moveforwardlist (struct node *, struct node *);
Task 3: Write a function that moves a node backward in the given linked list. Take the pointer of the node to be moved as a parameter.
void movebackwardlist (struct node *, struct node *);
Task 4: Write a function that loads the linked list with the given values of an array. Consider array and its size as input parameter to the function.
void loadlist (struct node *, int [ ], int );

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

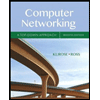
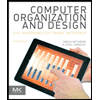
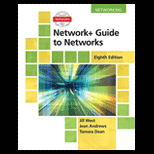
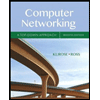
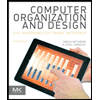
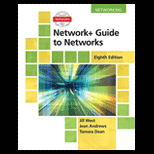
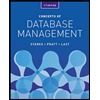
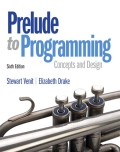
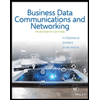