Take the following program and translate it into PEP/9 assembly language: #include using namespace std; int fib(int n) { int temp; if (n <= 0) return 0; else if (n <= 2) return 1; else { temp = fib(n – 1); return temp + fib(n-2); } } int main() { int num; cout << "Which fibonacci number? "; cin >> num; cout << fib(num) << endl; return 0; } You must use equates to access the stack and follow the call to the function as discussed in the book (pass the parameter, return address, return a value and so on). There are NO global variables in the resulting code (except a global message of "Range num? "). It must be able to do sum a range greater than 2. comments would be appreciated
Take the following program and translate it into PEP/9 assembly language:
#include <iostream>
using namespace std;
int fib(int n)
{
int temp;
if (n <= 0)
return 0;
else if (n <= 2)
return 1;
else {
temp = fib(n – 1);
return temp + fib(n-2);
}
}
int main()
{
int num;
cout << "Which fibonacci number? ";
cin >> num;
cout << fib(num) << endl;
return 0;
}
You must use equates to access the stack and follow the call to the function as discussed in the book (pass the parameter, return address, return a value and so on). There are NO global variables in the resulting code (except a global message of "Range num? "). It must be able to do sum a range greater than 2.
comments would be appreciated


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

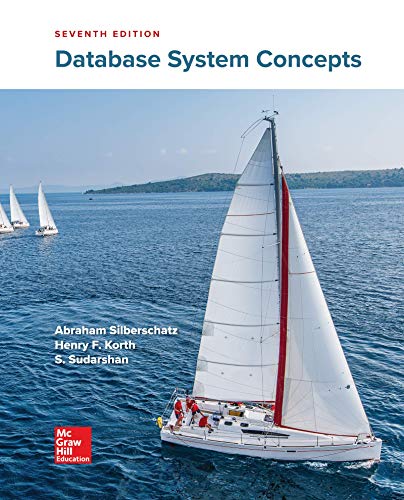
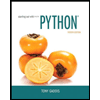
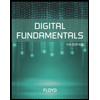
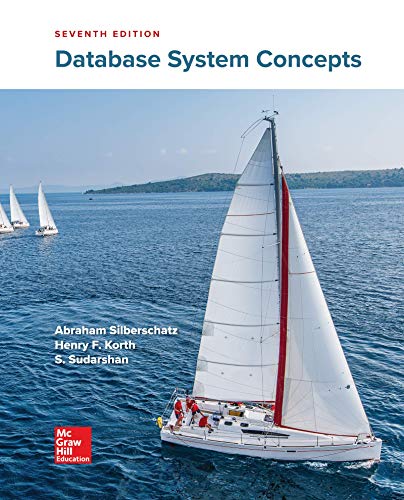
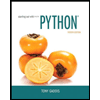
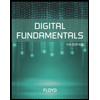
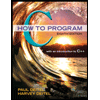
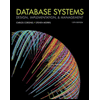
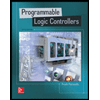