Suppose we have the following C function: long stir (long x) { if (x <= 1) { return 1; } // we're not concerned about small values long result = 0; if (x & 1) { result = x + 5; } else { result = x / 2; // if x is odd } return result; In the assembly implementation of the function below, fill in the three missing jump instructions so that it matches the code above: stir: cmpg $1, trdi labell movą $1, trax retą # return 1 labell: xorg trax, trax # result = 0 testg $1, trdi label2 movą šrdi, trax addą $5, $rax * result x + 5 label3 label2: movą trdi, trax shlq $1, trax * result = x / 2 label3: retą
Suppose we have the following C function: long stir (long x) { if (x <= 1) { return 1; } // we're not concerned about small values long result = 0; if (x & 1) { result = x + 5; } else { result = x / 2; // if x is odd } return result; In the assembly implementation of the function below, fill in the three missing jump instructions so that it matches the code above: stir: cmpg $1, trdi labell movą $1, trax retą # return 1 labell: xorg trax, trax # result = 0 testg $1, trdi label2 movą šrdi, trax addą $5, $rax * result x + 5 label3 label2: movą trdi, trax shlq $1, trax * result = x / 2 label3: retą
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Need help with computer science Question.

Transcribed Image Text:### C Function and Assembly Translation
#### C Function
```c
long stir(long x) {
if (x <= 1) { return 1; } // we're not concerned about small values
long result = 0;
if (x & 1) { // if x is odd
result = x + 5;
} else {
result = x / 2;
}
return result;
}
```
#### Assembly Implementation
The task is to fill in the three missing jump instructions in the assembly code to match the C function above.
```assembly
stir:
cmpq $1, %rdi
<missing jump> # fill this to direct the flow to either label1 or next line
movq $1, %rax # return 1
retq
label1:
xorq %rax, %rax # result = 0
testq $1, %rdi
<missing jump> # fill this based on whether x is odd
movq %rdi, %rax
addq $5, %rax # result = x + 5
<missing jump> # fill this to skip the next block of code
label2:
movq %rdi, %rax
shrq $1, %rax # result = x / 2
label3:
retq
```
### Explanation
The C function `stir` takes a long integer `x` and returns:
- `1` if `x` is less than or equal to `1`.
- `x + 5` if `x` is odd.
- `x / 2` if `x` is even.
The assembly code follows the same logic. The missing jump instructions need to properly direct the control flow:
- **First missing jump**: If `x` is greater than `1`, jump to `label1` to continue the logic for handling odd or even values.
- **Second missing jump**: After checking if `x` is odd with `testq`, jump to `label2` if `x` is not odd (i.e., when even).
- **Third missing jump**: After executing the `x + 5` addition logic, jump to `label3` to return the correct result, bypassing the division logic.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
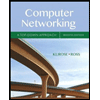
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
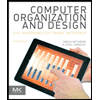
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
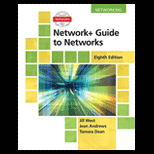
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
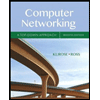
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
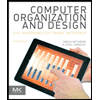
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
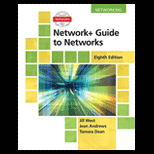
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
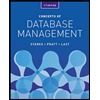
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
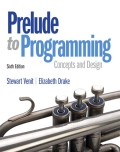
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
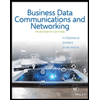
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY