Suppose the following 4-dimensional array String[][][][] transactions contains the information of transactions made in a store. The first dimension refers to which user, the second dimension refers to which order, the third dimension refers to which item, and the fourth dimension contains a unique identification code for the item and the cost of the item. For example, transactions [1][0][1] represents the item 1 in order 0 made by user 1, and refers to the one-dimensional array {"2205", "99.99"} which means the item code is "2205" and user 1 spent $99.99 on this item in this order. Please note both the item code and the cost are in String format. You may copy the following definition of the array to your program. String[] transactions = { // user 0 { {{"1101", "22.50"}, {"2103", "5.67"} }, // order 0 of user 0 {{"1101", "12.56"}, {"3405", "33.87"}, {"2205", "99.99"}}, // order 1 of user 0 {{"2401", "14.23"}} // order 2 of user 0 // user 1 { {{"2101","12.55"}, {"2205", "99.99"} }, // order 0 of user 1 {{"1101", "18.97"}, {"2402", "10.01"} }, // order 1 of user 1 }, // user 2 { {{"2205", "99.99"} }, // order 0 of user 2 }, // user 3 { {{"2401", "7.87"} }, // order 0 of user 3 {{"1101", "15.50"}, {"2402", "12.23"} }, // order 1 of user 3 }, // user 4 { {{"2401", "18.37"}}, // order 0 of user 4 {{"3405", "20.70"}, {"2401", "8.98"} }, // order 1 of user 4 {{"1101", "17.68"}, {"2505", "2.43"}, {"4040", "10.99"}} // order 2 of user 4
Suppose the following 4-dimensional array String[][][][] transactions contains the information of transactions made in a store. The first dimension refers to which user, the second dimension refers to which order, the third dimension refers to which item, and the fourth dimension contains a unique identification code for the item and the cost of the item. For example, transactions [1][0][1] represents the item 1 in order 0 made by user 1, and refers to the one-dimensional array {"2205", "99.99"} which means the item code is "2205" and user 1 spent $99.99 on this item in this order. Please note both the item code and the cost are in String format. You may copy the following definition of the array to your program. String[] transactions = { // user 0 { {{"1101", "22.50"}, {"2103", "5.67"} }, // order 0 of user 0 {{"1101", "12.56"}, {"3405", "33.87"}, {"2205", "99.99"}}, // order 1 of user 0 {{"2401", "14.23"}} // order 2 of user 0 // user 1 { {{"2101","12.55"}, {"2205", "99.99"} }, // order 0 of user 1 {{"1101", "18.97"}, {"2402", "10.01"} }, // order 1 of user 1 }, // user 2 { {{"2205", "99.99"} }, // order 0 of user 2 }, // user 3 { {{"2401", "7.87"} }, // order 0 of user 3 {{"1101", "15.50"}, {"2402", "12.23"} }, // order 1 of user 3 }, // user 4 { {{"2401", "18.37"}}, // order 0 of user 4 {{"3405", "20.70"}, {"2401", "8.98"} }, // order 1 of user 4 {{"1101", "17.68"}, {"2505", "2.43"}, {"4040", "10.99"}} // order 2 of user 4
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write the program in java and do what in the instructions says please do not plagarise from other sources or copy anything from others
![Suppose the following 4-dimensional array String[][][][] transactions contains the
information of transactions made in a store. The first dimension refers to which user,
the second dimension refers to which order, the third dimension refers to which item,
and the fourth dimension contains a unique identification code for the item and the
cost of the item. For example, transactions [1][0][1] represents the item 1 in order 0
made by user 1, and refers to the one-dimensional array {"2205","99.99"} which
means the item code is "2205" and user 1 spent $99.99 on this item in this order.
Please note both the item code and the cost are in String format. You may copy the
following definition of the array to your program.
String[][][][] transactions = {
// user 0
{
};
{{"1101", "22.50"}, {"2103", "5.67"} }, // order 0 of user 0
{{"1101", "12.56"}, {"3405", "33.87"}, {"2205", "99.99"}}, // order 1 of user 0
{{"2401", "14.23"}} // order 2 of user 0
},
// user 1
{
{{"2101", "12.55"}, {"2205", "99.99"} }, // order 0 of user 1
{{"1101", "18.97"}, {"2402", "10.01"} }, // order 1 of user 1
},
// user 2
{
}
{{"2205", "99.99"} }, // order 0 of user 2
},
// user 3
{
{{"2401", "7.87"} }, // order 0 of user 3
{{"1101", "15.50"}, {"2402", "12.23"} }, // order 1 of user 3
},
// user 4
{
{{"2401", "18.37"}}, // order 0 of user 4
{{"3405", "20.70"}, {"2401", "8.98"} }, // order 1 of user 4
{{"1101", "17.68"}, {"2505", "2.43"}, {"4040", "10.99"}} // order 2 of user 4](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F83cebb0c-db38-4f0e-a0d3-5fd209e24564%2Feea0d9fd-9d67-49d1-8c64-956aefce00bf%2Foxjhxlr_processed.png&w=3840&q=75)
Transcribed Image Text:Suppose the following 4-dimensional array String[][][][] transactions contains the
information of transactions made in a store. The first dimension refers to which user,
the second dimension refers to which order, the third dimension refers to which item,
and the fourth dimension contains a unique identification code for the item and the
cost of the item. For example, transactions [1][0][1] represents the item 1 in order 0
made by user 1, and refers to the one-dimensional array {"2205","99.99"} which
means the item code is "2205" and user 1 spent $99.99 on this item in this order.
Please note both the item code and the cost are in String format. You may copy the
following definition of the array to your program.
String[][][][] transactions = {
// user 0
{
};
{{"1101", "22.50"}, {"2103", "5.67"} }, // order 0 of user 0
{{"1101", "12.56"}, {"3405", "33.87"}, {"2205", "99.99"}}, // order 1 of user 0
{{"2401", "14.23"}} // order 2 of user 0
},
// user 1
{
{{"2101", "12.55"}, {"2205", "99.99"} }, // order 0 of user 1
{{"1101", "18.97"}, {"2402", "10.01"} }, // order 1 of user 1
},
// user 2
{
}
{{"2205", "99.99"} }, // order 0 of user 2
},
// user 3
{
{{"2401", "7.87"} }, // order 0 of user 3
{{"1101", "15.50"}, {"2402", "12.23"} }, // order 1 of user 3
},
// user 4
{
{{"2401", "18.37"}}, // order 0 of user 4
{{"3405", "20.70"}, {"2401", "8.98"} }, // order 1 of user 4
{{"1101", "17.68"}, {"2505", "2.43"}, {"4040", "10.99"}} // order 2 of user 4

Transcribed Image Text:Write a program that prints out summary for each user, including the total of each
order made by the user and the total spending of the user across orders. Print out all
the totals with a precision of 2 digits. Draw pictures to help you understand the
structure of the array. A sample run is as follows:
Summary for user 0:
Order 0 total: $28.17
Order 1 total: $146.42
Order 2 total: $14.23
Total spending: $188.82
Summary for user 1:
Order 0 total: $112.54
Order 1 total: $28.98
Total spending: $141.52
Summary for user 2:
Order 0 total: $99.99
Total spending: $99.99
Summary for user 3:
Order 0 total: $7.87
Order 1 total: $27.73
Total spending: $35.60
Summary for user 4:
Order 0 total: $18.37
Order 1 total: $29.68
Order 2 total: $31.10
Total spending: $79.15
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
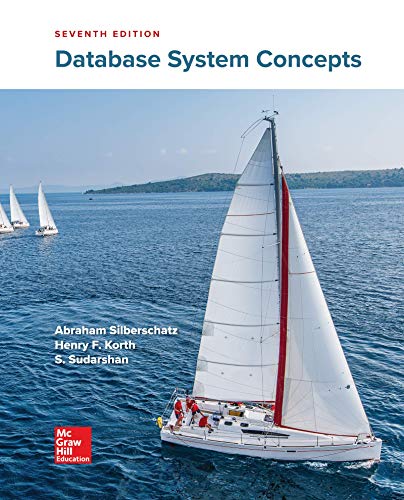
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
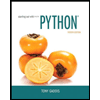
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
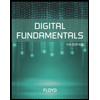
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
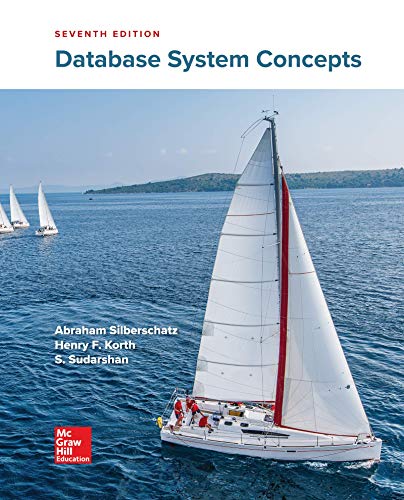
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
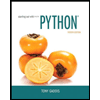
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
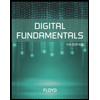
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
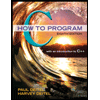
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
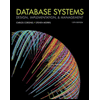
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
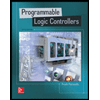
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education