Suppose num is an int variable, declared and initialized. The value of num is between 2 and 12. Write a C# nested-if-else statement to output • "Game status is WON" if num is 7 or 11 • "Game status is LOST" if num is 2, 3 or 12 "Game status is CONTINUE" if num is 4, 5, 6, 8, 9 or 10
Suppose num is an int variable, declared and initialized. The value of num is between 2 and 12. Write a C# nested-if-else statement to output • "Game status is WON" if num is 7 or 11 • "Game status is LOST" if num is 2, 3 or 12 "Game status is CONTINUE" if num is 4, 5, 6, 8, 9 or 10
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
c#

Transcribed Image Text:### Understanding Nested If-Else Statements in C#
In this tutorial, we will discuss how to properly implement a nested if-else statement in C# to determine the game status based on the value of an integer variable `num`. Assume that `num` is an integer variable that has been declared and initialized. The possible values for `num` range between 2 and 12.
Here's what we want our program to do:
- Output "Game status is WON" if `num` is 7 or 11.
- Output "Game status is LOST" if `num` is 2, 3, or 12.
- Output "Game status is CONTINUE" if `num` is 4, 5, 6, 8, 9, or 10.
To achieve this, we will use nested if-else statements. Nested if-else statements allow us to check multiple conditions based on the preceding conditions. Below is a detailed breakdown of how to write this logic in C#:
### Code Explanation
```csharp
int num = /* Initialize num with a value between 2 and 12 */;
if (num == 7 || num == 11)
{
Console.WriteLine("Game status is WON");
}
else
{
if (num == 2 || num == 3 || num == 12)
{
Console.WriteLine("Game status is LOST");
}
else
{
Console.WriteLine("Game status is CONTINUE");
}
}
```
### Step-by-Step Explanation
1. **Condition Check for "WON"**:
- The first if condition checks if `num` is either 7 or 11.
- If true, it prints "Game status is WON".
2. **Condition Check for "LOST"**:
- If the first condition is false, the program executes the else block.
- Within this else block, another if statement checks if `num` is either 2, 3, or 12.
- If true, it prints "Game status is LOST".
3. **Condition Check for "CONTINUE"**:
- If none of the above conditions are satisfied, the final else block is executed.
- This else block catches values of `num` that are 4, 5, 6, 8, 9, or 10.
- It prints "Game status is
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
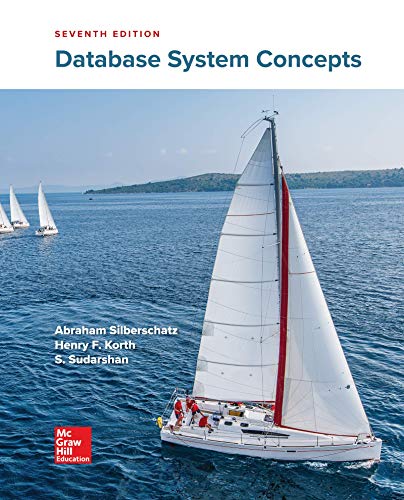
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
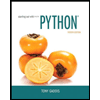
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
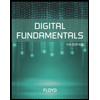
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
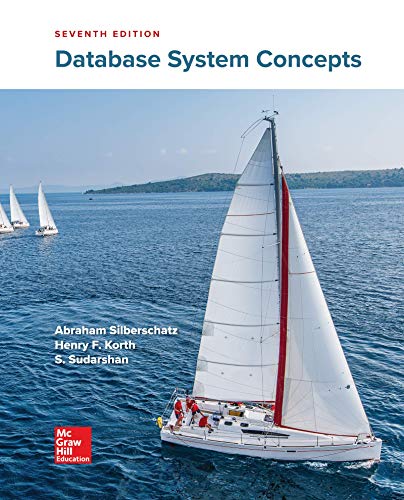
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
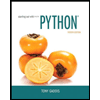
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
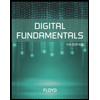
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
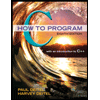
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
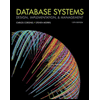
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
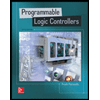
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education