Suppose a BST contains the integer values 1 through 15 and is a perfect binary tree. a) Draw the tree. b) Suppose we call the removeViaReplace method, with r equal to the root of the tree in Part a. List the numbers in child->value at the beginning of each iteration of the while loop in the code below. After that, redraw the tree upon completion of the removeViaReplace function. c) Repeat Part b, but use the tree obtained at the end of Part b as the initial tree this time. static void removeViaReplace(Node* r) { Node* temp = r->right; if (temp->left == nullptr) { r->value = temp->value; r->right = temp->right; delete temp; } else { Node* child = temp->left, * parent = temp; while (child->left != nullptr) { parent = child; child = child->left; } r->value = child->value; parent->left = child->right; delete child; } }
Suppose a BST contains the integer values 1 through 15 and is a perfect binary tree.
a) Draw the tree.
b) Suppose we call the removeViaReplace method, with r equal to the root of the tree in Part a. List the numbers in child->value at the beginning of each iteration of the while loop in the code below. After that, redraw the tree upon completion of the removeViaReplace function.
c) Repeat Part b, but use the tree obtained at the end of Part b as the initial tree this time.
static void removeViaReplace(Node* r) {
Node* temp = r->right;
if (temp->left == nullptr) {
r->value = temp->value;
r->right = temp->right;
delete temp;
}
else {
Node* child = temp->left, * parent = temp;
while (child->left != nullptr) {
parent = child;
child = child->left;
}
r->value = child->value;
parent->left = child->right;
delete child;
}
}

Answer:
we have draw the Binary search tree as a perfect binary tree and also we have explain the function how it working
Step by step
Solved in 3 steps with 1 images

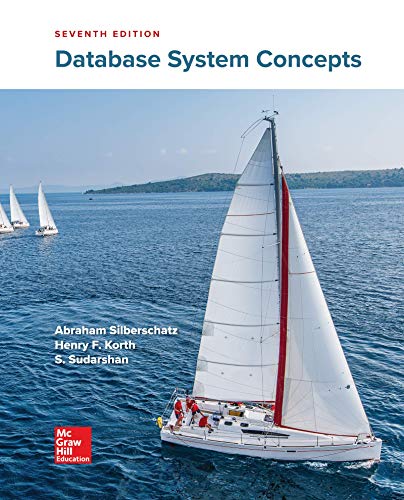
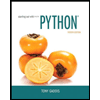
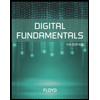
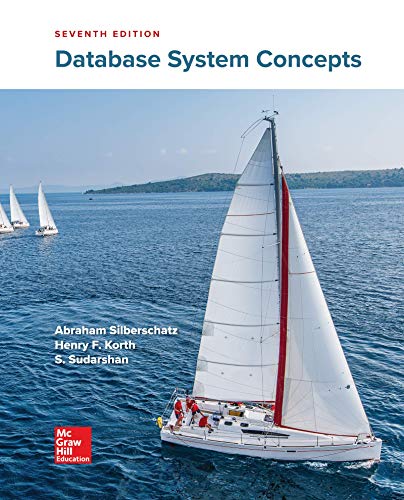
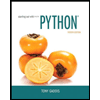
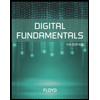
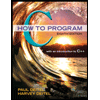
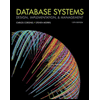
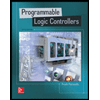