Some students have organized an election to determine the new leaders of their school club. Each member of the club has a unique ID that they can use when they vote. However, the election managers suspect that some members have voted twice. Write a program to read in member IDs and check if there are any repeated values. If an ID has already been used, output a line in this format: REPEAT: The ID BE45N6 has already been counted! Stop reading input after reading the value "STOP". Then output a line showing how many unique members voted in this format After excluding repeats, 45 unique members voted. X Full Screen ¢ p5.pp O include O New using namespace std3; int main() { // TODO: Write your code here cout "REPEAT: The ID" "AABBAB" * has already been counted!" endl; 10 11 cout "After excluding repeats, " «e " unique members voted." endl; 12 }
Some students have organized an election to determine the new leaders of their school club. Each member of the club has a unique ID that they can use when they vote. However, the election managers suspect that some members have voted twice. Write a program to read in member IDs and check if there are any repeated values. If an ID has already been used, output a line in this format: REPEAT: The ID BE45N6 has already been counted! Stop reading input after reading the value "STOP". Then output a line showing how many unique members voted in this format After excluding repeats, 45 unique members voted. X Full Screen ¢ p5.pp O include O New using namespace std3; int main() { // TODO: Write your code here cout "REPEAT: The ID" "AABBAB" * has already been counted!" endl; 10 11 cout "After excluding repeats, " «e " unique members voted." endl; 12 }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:**Project Overview: Detecting Duplicates in School Election Voting**
Some students have organized an election to determine the new leaders of their school club. Each member of the club has a unique ID that they use when voting. However, the election managers suspect that some members have voted twice.
**Task:**
Write a program to read in member IDs and check if there are any repeated values. If an ID has already been used, output a line in this format:
`REPEAT: The ID BE45N6 has already been counted!`
Stop reading input after the value "STOP" is encountered. Then output a line showing how many unique members voted in this format:
`After excluding repeats, 45 unique members voted.`
**Code Snippet Explanation:**
Below is a simple C++ code structure to solve the problem:
```cpp
#include <iostream>
using namespace std;
int main() {
// TODO: Write your code here
cout << "REPEAT: The ID " << "AAA00A" << " has already been counted!" << endl;
cout << "After excluding repeats, " << 0 << " unique members voted." << endl;
}
```
**Code Explanation:**
- The program is structured to demonstrate how to identify duplicate votes in an election.
- Initially, it includes the iostream library to handle input and output operations.
- Using the `std` namespace simplifies the code by allowing direct access to functions like `cout`.
- The `main()` function is where the program execution begins.
- `TODO` comments indicate where you should write the actual logic to process member IDs, check for duplicates, and tally unique votes.
- Two `cout` statements demonstrate the expected output format for when a duplicate ID is detected and when displaying the count of unique votes.
The code structure will need logic for storing and checking IDs, responding to duplicates, and calculating the total number of unique votes to be fully functional.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
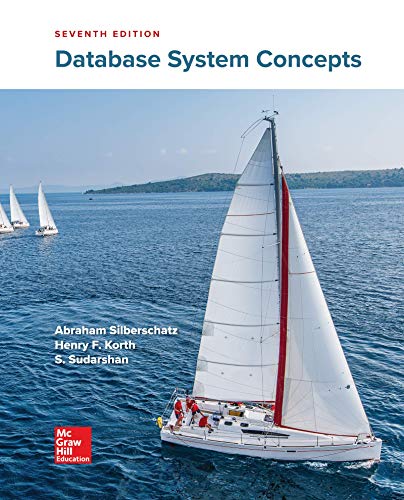
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
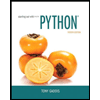
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
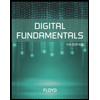
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
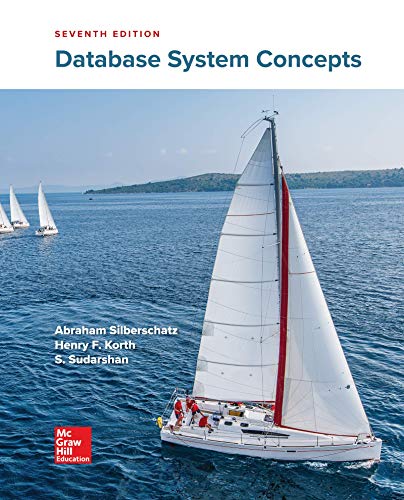
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
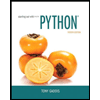
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
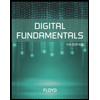
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
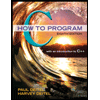
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
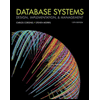
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
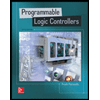
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education