So I am trying to write a code that will tell you if a year is a leap year or not but so far it is only acknowledging centuries (1600, 1700, 1800, etc.). Any advice on where my code seems wrong? input_year = int(input()) def is_leap_year(input_year): if input_year % 4 == 0: if input_year % 100 == 0: if input_year % 400 == 0: return True else: return False else: return False else: return False if is_leap_year(input_year): print(input_year, '- leap year') else: print(input_year, '- not a leap year')
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
So I am trying to write a code that will tell you if a year is a leap year or not but so far it is only acknowledging centuries (1600, 1700, 1800, etc.). Any advice on where my code seems wrong?
input_year = int(input())
def is_leap_year(input_year):
if input_year % 4 == 0:
if input_year % 100 == 0:
if input_year % 400 == 0:
return True
else:
return False
else:
return False
else:
return False
if is_leap_year(input_year):
print(input_year, '- leap year')
else:
print(input_year, '- not a leap year')

let's break down the logic of the code:
Input Year: The code starts by taking user input for a year as an integer.
is_leap_year
Function:- This function checks whether a given year is a leap year or not based on the rules for leap years:
- A year is a leap year if it is divisible by 4.
- However, if a year is divisible by 100, it is not a leap year, unless it is also divisible by 400, in which case it is a leap year.
- This function checks whether a given year is a leap year or not based on the rules for leap years:
Return Statement:
- The function uses a single
return
statement to check both conditions:- It first checks if the year is divisible by 4 (
input_year % 4 == 0
). - Then, it checks that the year is either not divisible by 100 (
input_year % 100 != 0
) or it is divisible by 400 (input_year % 400 == 0
). This condition ensures that years like 1700, 1800, and 1900 are not considered leap years, but years like 1600 and 2000 are.
- It first checks if the year is divisible by 4 (
- The function uses a single
Printing the Result:
- Finally, the code calls the
is_leap_year
function with the input year and prints the result. If the function returnsTrue
, it prints that it's a leap year; otherwise, it prints that it's not a leap year.
- Finally, the code calls the
This logic correctly identifies leap years according to the rules, making it an accurate and concise way to determine leap years in Python.
Step by step
Solved in 3 steps

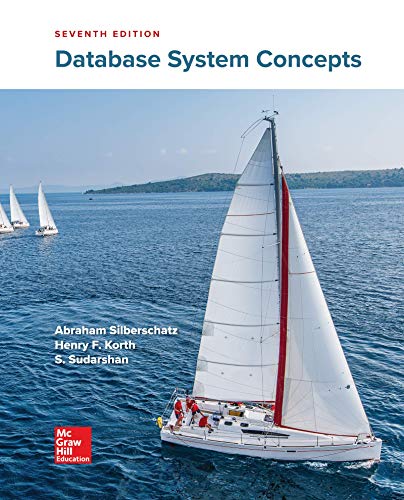
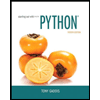
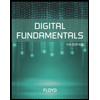
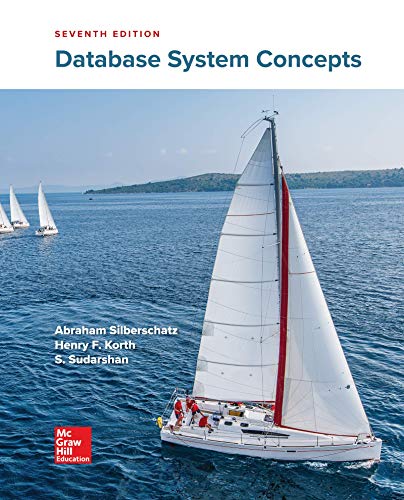
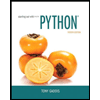
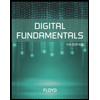
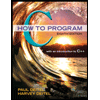
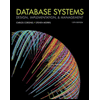
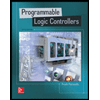