Simple queue implementation please help (looks like a lot but actually isnt, everything is already set up for you to make it even easier) help in any part you can, please be clear, thank you Given an interface for Queue - Without using the java collections interface (ie do not import java.util.List, LinkedList, Stack, Queue...) - Create an implementation of Queue interface provided - For the implementation create a tester to verify the implementation of that
Simple queue implementation
please help (looks like a lot but actually isnt, everything is already set up for you to make it even easier)
help in any part you can, please be clear, thank you
Given an interface for Queue
- Without using the java collections interface (ie do not import java.util.List,
LinkedList, Stack, Queue...)
- Create an implementation of Queue interface provided
- For the implementation create a tester to verify the implementation of that
data structure performs as expected
Wait in line – Queue (fifo)
- Implement the provided Queue interface ( fill out the implementation shell)
- Put your implementation through its paces by exercising each of the methods in
a test harness
- Add to your ‘BusClient’ the following functionality using your Queue
-
o Create (enqueue) 6 riders by name
§ Iterate over the queue, print all riders
o Peek at the queue / print the result
o Remove (dequeue) the head of the queue
§ Iterate over the queue, print all riders
o Add two more riders to the queue
o Peek at the queue & print the result
o Remove the head & print the result
§ Iterate over the queue, print all riders
4 CLASSES TO BEGIN WITH BELOW (edit these classes to fulfill the easy requirements above)
import linkedList.LinkedList;
import linkedList.LinkedListImpl;
import queue.Queue;
import queue.QueueImpl;
import stack.Stack;
import stack.StackImpl;
public class BusClient {
public static void main(String[] args) {
// create implementation, then
System.out.println("----Q U E U E T E S T-------");
//QueueTestMethod...
}
}
----------
package queue;
public interface Queue {
boolean isFull() ;
boolean isEmpty();
// insert elements to the queue
void enQueue(String element);
// delete element from the queue
String deQueue();
// display element of the queue
void display();
//display 'first' element
public String peek();
}
----------
package queue;
public class QueueImpl implements Queue {
private int LENGTH = 6;
private String[] arr = new String[LENGTH];
private int size = 0;
@Override
public boolean isFull() {
if (arr[LENGTH - 1] != null) {
return true;
}
return false;
}
@Override
public boolean isEmpty() {
if(size > 0)
return false;
else
return true;
}
@Override
public void enQueue(String element) {
if(isFull()) {
System.out.println("sorry, full queue");
}else {
if (isEmpty()) {
arr[0] = element;
size++;
}
else {
//shift all elements 1 towards the end
//everybody shift one to the right..
for (int i = size; i > 0; i--) {
arr[i] = arr[i - 1];
}
//add to the 0th position
arr[0] = element;
size++;
}
}
}
@Override
public String deQueue() {
// TODO Auto-generated method stub
return null;
}
@Override
public void display() {
// TODO Auto-generated method stub
}
@Override
public String peek() {
// TODO Auto-generated method stub
return null;
}
}
----------
package queue;
public class QueueTester {
public static void main(String[] args) {
Queue q = new QueueImpl();
q.enQueue("sam");
q.enQueue("pam");
q.enQueue("will");
q.enQueue("jill");
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

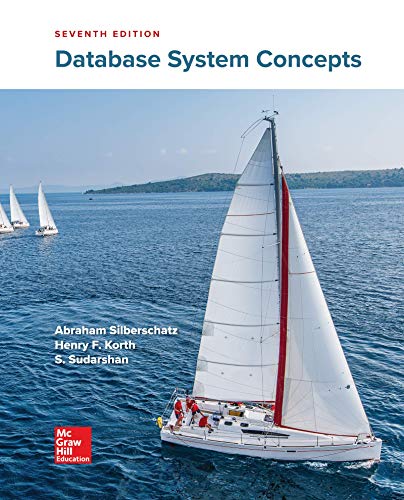
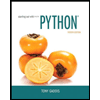
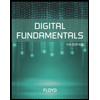
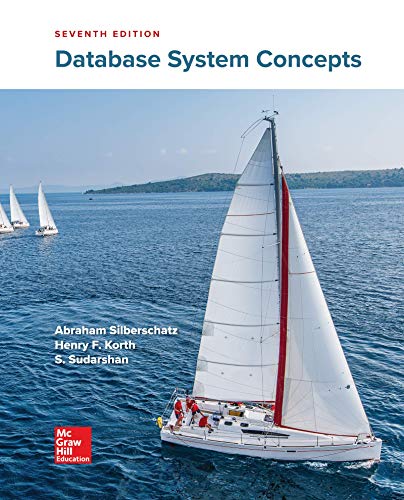
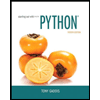
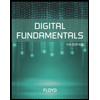
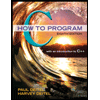
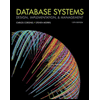
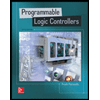