selection vs. insertion sort
for given A and B s :
import random
A = random.sample(range(0, 101), random.randint(1,100))
A.sort
print(A)
B = random.sample(range(0, 101), random.randint(1,100))
B.sort(reverse=True)
print(B)
Conduct doubling experiments to compare the run time of selection vs. insertion sort (for both A and B). Plot the results (both
case of array) for array sizes n = 2, 4, ... 1024. insertion and selection sort are provided below
import time
import numpy as np
### Selection Sort ###
def selection_sort(A):
for i in range(len(A)):
min_idx = i
for j in range(i + 1, len(A)):
if A[min_idx] > A[j]:
min_idx = j
A[i], A[min_idx] = A[min_idx], A[i]
return A
### Insertion Sort ###
def insertion_sort(A):
n = len(A)
count = 0
for i in range(1,n):
k = i
while k > 0 and A[k] < A[k-1]:
A[k], A[k-1] = A[k-1], A[k]
k = k - 1
count += 1
return count, A
provide code and result

Step by step
Solved in 2 steps

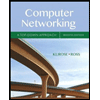
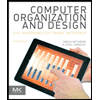
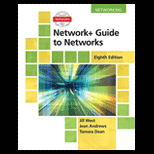
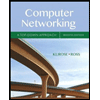
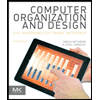
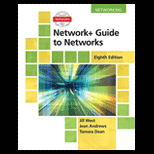
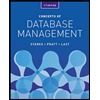
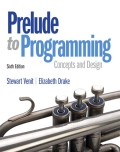
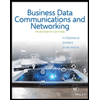