runtime complexity
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I need help finding the runtime complexity Off the following code.
![Here is the transcription of the image, suitable for display on an educational website:
---
/* In the worst-case scenario: what is the value of count for a sorted array A of size 'n'? */
```java
static int count = 0;
public static boolean binS(int[] A, int val) {
return binS(A, val, 0, A.length-1);
}
public static boolean binS(int[] A, int val, int l, int h) {
count++;
if( l > h ) {
return false;
}
int m = (l+h)/2;
if( A[m] == val )
return true;
else if( A[m] < val )
return binS(A, val, m+1, h);
else
return binS(A, val, l, m-1);
}
```
/* How would you describe the runtime of the above code?
In the best-case scenario, what is the value of count in the above code? */
---
**Explanation:**
The code above implements a recursive binary search algorithm for a sorted array `A`. Key points include:
- **Count Variable**: An integer `count` is initialized to zero and incremented with each recursive call to `binS`. This tracks the number of calls made in the search process.
- **Function binS**:
- **Parameters**: Takes an array `A`, a value `val`, and optionally, low `l` and high `h` indices for searching the subarray.
- **Base Condition**: If `l > h`, it returns `false`, indicating the value is not found.
- **Middle Calculation**: Computes the midpoint `m` as `(l + h) / 2`.
- **Comparison**: Checks if the middle element `A[m]` equals the target value `val`.
- If equal, returns `true`.
- If `A[m]` is less than `val`, searches the right subarray.
- Otherwise, searches the left subarray.
- **Runtime Considerations**:
- **Worst-Case Scenario**: The value of `count` will be O(log n), indicating the number of recursive calls made when the element is not found.
- **Best-Case Scenario**: `count` will be 1 if the middle element is the](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F84b9759d-3bd7-481b-9da5-01ee09be0953%2Ff8e1a08a-2d97-471d-aaf6-40741bf9bd1f%2Fb250mum_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Here is the transcription of the image, suitable for display on an educational website:
---
/* In the worst-case scenario: what is the value of count for a sorted array A of size 'n'? */
```java
static int count = 0;
public static boolean binS(int[] A, int val) {
return binS(A, val, 0, A.length-1);
}
public static boolean binS(int[] A, int val, int l, int h) {
count++;
if( l > h ) {
return false;
}
int m = (l+h)/2;
if( A[m] == val )
return true;
else if( A[m] < val )
return binS(A, val, m+1, h);
else
return binS(A, val, l, m-1);
}
```
/* How would you describe the runtime of the above code?
In the best-case scenario, what is the value of count in the above code? */
---
**Explanation:**
The code above implements a recursive binary search algorithm for a sorted array `A`. Key points include:
- **Count Variable**: An integer `count` is initialized to zero and incremented with each recursive call to `binS`. This tracks the number of calls made in the search process.
- **Function binS**:
- **Parameters**: Takes an array `A`, a value `val`, and optionally, low `l` and high `h` indices for searching the subarray.
- **Base Condition**: If `l > h`, it returns `false`, indicating the value is not found.
- **Middle Calculation**: Computes the midpoint `m` as `(l + h) / 2`.
- **Comparison**: Checks if the middle element `A[m]` equals the target value `val`.
- If equal, returns `true`.
- If `A[m]` is less than `val`, searches the right subarray.
- Otherwise, searches the left subarray.
- **Runtime Considerations**:
- **Worst-Case Scenario**: The value of `count` will be O(log n), indicating the number of recursive calls made when the element is not found.
- **Best-Case Scenario**: `count` will be 1 if the middle element is the
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
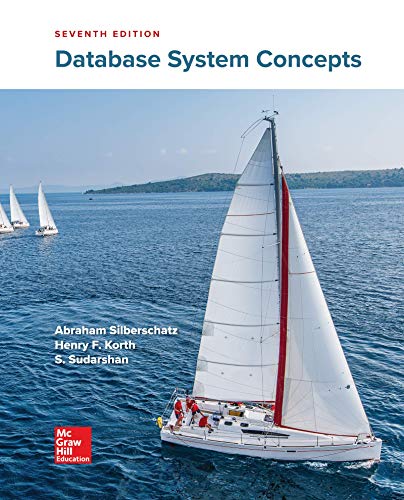
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
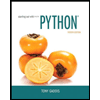
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
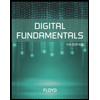
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
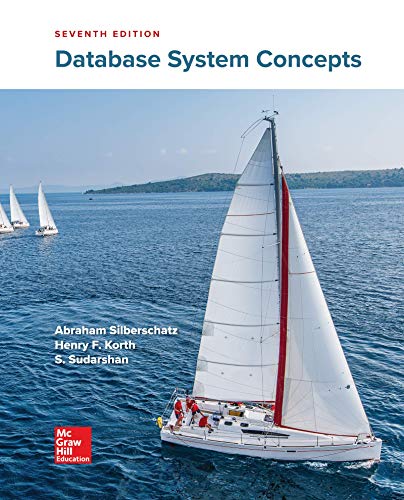
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
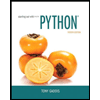
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
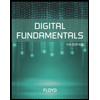
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
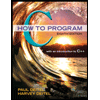
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
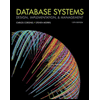
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
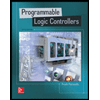
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education