Returns the count of the numbers from 1 to N, inclusive, that are divisible by argument a or argument b, but not both
import unittest
# ------------------------------------------------------------
def enumerate6(N, a, b) :
'''
Assumes that N, a, b are positive integers.
Returns the count of the numbers from 1 to N, inclusive,
that are divisible by argument a or argument b, but not both
For example, enumerate6(10, 2, 5) returns 5,
since 2, 4, 5, 6, 8 are the numbers from 1 to 10 that
are divisible by 2 or 5 but not both
'''
pass
# --------------------------------------------------------------
# The Testing
# --------------------------------------------------------------
class myTests(unittest.TestCase):
def test1(self):
ans = enumerate6(10, 2, 5)
self.assertEqual(ans, 5)
def test2(self):
ans = enumerate6(12, 2, 5)
self.assertEqual(ans, 6)
def test3(self):
ans = enumerate6(5000, 4, 2)
self.assertEqual(ans, 1875)
def test4(self):
ans = enumerate6(50000, 3, 8)
self.assertEqual(ans, 18750)
def test5(self):
ans = enumerate6(12131, 7, 2)
self.assertEqual(ans, 6066)
if __name__ == '__main__':
unittest.main(exit=True)

Step by step
Solved in 4 steps with 2 images

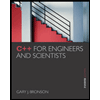
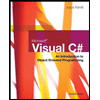
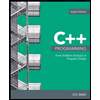
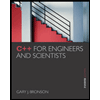
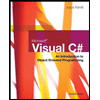
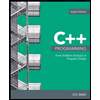