Redo the program given where you have to define and use the following functions: (a) isTriangle - takes 3 sides of a triangle and returns true if they form a triangle, false otherwise. (b) isIsosceles - takes 3 sides of a triangle and returns true if they form an isosceles triangle, false otherwise. (c) isEquilateral - takes 3 sides of a triangle and returns true if they form an equilateral triangle, false otherwise. (d) isRight - takes 3 sides of a triangle and returns true if they form a right triangle, false otherwise. (e) your program should run continuous until the user wants to quit
Redo the program given where you have to define and use the following functions:
(a) isTriangle - takes 3 sides of a triangle and returns true if they form a triangle, false otherwise.
(b) isIsosceles - takes 3 sides of a triangle and returns true if they form an isosceles triangle, false otherwise.
(c) isEquilateral - takes 3 sides of a triangle and returns true if they form an
equilateral triangle, false otherwise.
(d) isRight - takes 3 sides of a triangle and returns true if they form a right triangle, false otherwise.
(e) your program should run continuous until the user wants to quit
The given program:
#include <iostream>
#include <cmath>
using namespace std;
// Constant Declarations
const double E = .0001;
int main()
{
double side1, side2, side3;
bool isTriangle, isRight, isEquilateral, isIsosceles;
cout << "Enter the lengths of the 3 sides of a triangle -- "; cin >> side1 >> side2 >> side3;
isTriangle = side1 + side2 > side3 &&side1 + side3 > side2 && side2 + side3 > side1;
if (isTriangle) {
cout << "This is ";
isEquilateral = fabs(side1 - side2) < E && fabs(side1 - side3) < E;
isIsosceles = fabs(side1 - side2) < E || fabs(side1 - side3) < E || fabs(side2 - side3) < E;
if (isEquilateral) cout << "an equilateral ";
else if (isIsosceles) cout << "an isosceles ";
else cout << "a scalene ";
isRight = fabs(pow(side1, 2) - pow(side2, 2) - pow(side3, 2)) < E || fabs(pow(side2, 2) - pow(side1, 2) - pow(side3, 2)) < E || fabs(pow(side3, 2) - pow(side1, 2) - pow(side2, 2)) < E;
if (isRight)
cout << "and right ";
cout << "triangle" << endl;
}
else cout << "This is not a triangle" << endl;
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

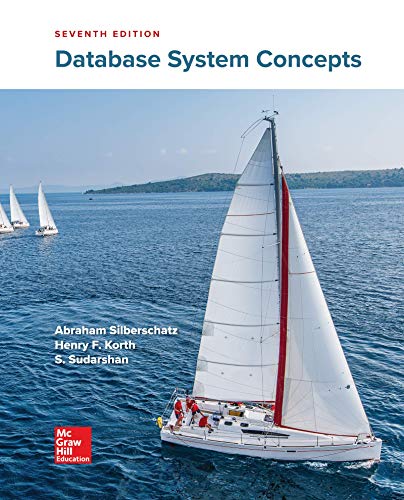
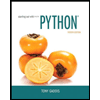
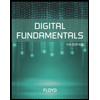
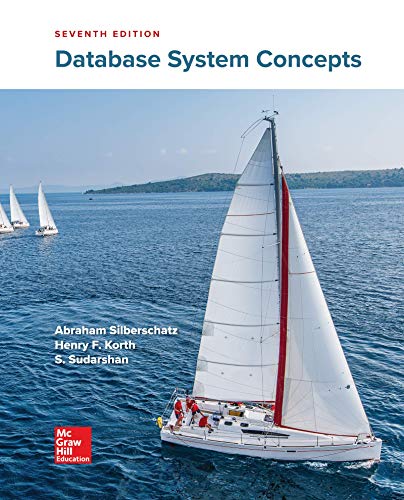
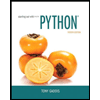
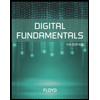
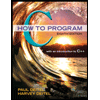
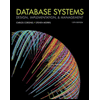
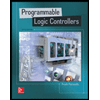