Redesign the topsort algorithm below so that it selects the last node in each iteration rather than the first. Please use python ad show all work so I can better understand the problem. def topsort(G): count = dict((u, 0) for u in G) for u in G: # The in-degree for each node for v in G[u]: count[v] += 1 Q = [u for u in G if count[u] == 0] S - () while Q: # Count every in-edge # Valid initial nodes # The result # while we have start nodes... # Pick one # Use it as first of the rest u - Q. pop() S. append (u) for v in G[u]: count[v] -- 1 if count[v] == 0: Q.append(v) # "Uncount" its out-edges # New valid start nodes? # Deal with them next return S
Redesign the topsort algorithm below so that it selects the last node in each iteration rather than the first. Please use python ad show all work so I can better understand the problem. def topsort(G): count = dict((u, 0) for u in G) for u in G: # The in-degree for each node for v in G[u]: count[v] += 1 Q = [u for u in G if count[u] == 0] S - () while Q: # Count every in-edge # Valid initial nodes # The result # while we have start nodes... # Pick one # Use it as first of the rest u - Q. pop() S. append (u) for v in G[u]: count[v] -- 1 if count[v] == 0: Q.append(v) # "Uncount" its out-edges # New valid start nodes? # Deal with them next return S
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![**Topological Sort Algorithm Explanation and Redesign**
This image contains a Python function `topsort(G)` that performs a topological sort on a directed acyclic graph (DAG) represented as a dictionary `G`. The algorithm uses an in-degree method to determine a valid sort order. Here's a detailed breakdown:
### Original Algorithm:
1. **Calculate In-degrees:**
- The `count` dictionary stores the in-degree (number of incoming edges) for each node. It is initialized by setting each node's count to 0 and then incremented for every incoming edge to that node.
2. **Initialize Queue:**
- The list `Q` collects nodes with an in-degree of 0. These nodes have no dependencies and can be processed initially.
3. **Process Nodes:**
- An empty list `S` is initialized to hold the sorted order of nodes.
- Using a while loop, the algorithm continues while there are nodes in `Q`.
- Pop a node `u` from `Q`, append it to `S`, and decrement the in-degree of all its neighbors.
- If any neighbor’s in-degree becomes 0, it is added to `Q` for future processing.
### Requested Modification:
- The task is to redesign the algorithm to select and process the last node in the queue `Q` at each iteration rather than the first.
### Redesigned Algorithm:
To adjust the implementation so that it selects the last node in each iteration:
```python
def topsort(G):
count = dict((u, 0) for u in G) # The in-degree for each node
for u in G:
for v in G[u]:
count[v] += 1 # Count every in-edge
Q = [u for u in G if count[u] == 0] # Valid initial nodes
S = [] # The result
while Q: # While we have start nodes...
u = Q.pop() # Pick the last one
S.append(u) # Use it as first of the rest
for v in G[u]:
count[v] -= 1 # "Uncount" its out-edges
if count[v] == 0: # New valid start nodes?
Q.append(v) # Deal with them next
return S
```
### Explanation of Changes:
- **](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd7c4f06c-9313-4d00-9732-0b7c91b56bd5%2Ff449d289-07c8-445a-9b34-befd0fc97d52%2Fp25b682_processed.png&w=3840&q=75)
Transcribed Image Text:**Topological Sort Algorithm Explanation and Redesign**
This image contains a Python function `topsort(G)` that performs a topological sort on a directed acyclic graph (DAG) represented as a dictionary `G`. The algorithm uses an in-degree method to determine a valid sort order. Here's a detailed breakdown:
### Original Algorithm:
1. **Calculate In-degrees:**
- The `count` dictionary stores the in-degree (number of incoming edges) for each node. It is initialized by setting each node's count to 0 and then incremented for every incoming edge to that node.
2. **Initialize Queue:**
- The list `Q` collects nodes with an in-degree of 0. These nodes have no dependencies and can be processed initially.
3. **Process Nodes:**
- An empty list `S` is initialized to hold the sorted order of nodes.
- Using a while loop, the algorithm continues while there are nodes in `Q`.
- Pop a node `u` from `Q`, append it to `S`, and decrement the in-degree of all its neighbors.
- If any neighbor’s in-degree becomes 0, it is added to `Q` for future processing.
### Requested Modification:
- The task is to redesign the algorithm to select and process the last node in the queue `Q` at each iteration rather than the first.
### Redesigned Algorithm:
To adjust the implementation so that it selects the last node in each iteration:
```python
def topsort(G):
count = dict((u, 0) for u in G) # The in-degree for each node
for u in G:
for v in G[u]:
count[v] += 1 # Count every in-edge
Q = [u for u in G if count[u] == 0] # Valid initial nodes
S = [] # The result
while Q: # While we have start nodes...
u = Q.pop() # Pick the last one
S.append(u) # Use it as first of the rest
for v in G[u]:
count[v] -= 1 # "Uncount" its out-edges
if count[v] == 0: # New valid start nodes?
Q.append(v) # Deal with them next
return S
```
### Explanation of Changes:
- **
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
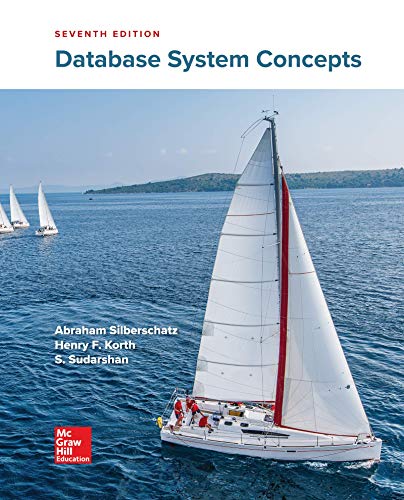
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
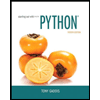
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
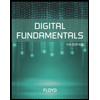
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
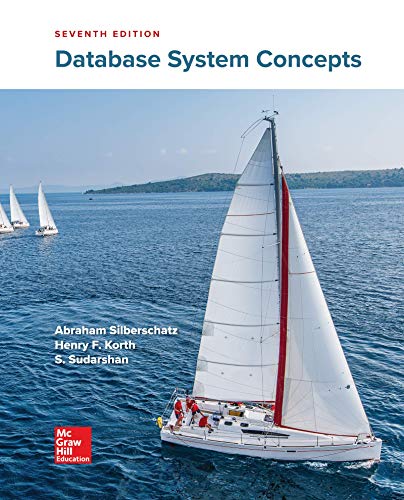
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
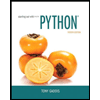
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
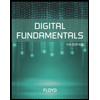
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
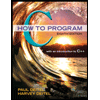
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
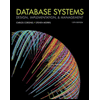
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
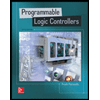
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education