QUESTION Checks whether the parenthesis in str are balanced using the stack. Returns 1, if balanced 0, it unbaianced An expression has balanced parenthesis if it satisfies the following conditions: 1. The first observed parenthesis cannot be a closing parenthesis 2. All opening parentheses should have matching closing parenthesis 3. The parentheses cannot be intertwined but can be nested */
PLEASE USE THESE CODES FOR SOLVİNG C QUESİTON
typedef struct LINKED_STACK_NODE_s *LINKED_STACK_NODE;
typedef struct LINKED_STACK_NODE_s{
LINKED_STACK_NODE next;
void *data;
} LINKED_STACK_NODE_t[1];
typedef struct LINKED_STACK_s{
LINKED_STACK_NODE head;
} LINKED_STACK_t[1], *LINKED_STACK;
// Creates and initializes a linked stack and returns its pointer
LINKED_STACK linked_stack_init()
{
LINKED_STACK new;
new = (LINKED_STACK) malloc(sizeof(LINKED_STACK_t));
if (new !=NULL)
{
new->head = NULL;
else
{
printf("problem cannot allocate memory");
}
return new;
}
// Deallocates the memory allocated by the stack
void linked_stack_free(LINKED_STACK stack)
{
while(stack->head !=NULL)
{
linked_stack_pop(stack);
}
free(stack);
}
// Pushes data into the stack following FIFO principle
void linked_stack_push(LINKED_STACK stack, void *data)
{
LINKED_STACK_NODE node = (LINKED_STACK_NODE)malloc(sizeof(LINKED_STACK_NODE_t));
if(node != NULL)
{
node->data = data ;
node->next = stack -> head;
stack->head = node ;
}
else
{
printf("error! about memory allocating ");
}
}
// Pops and returns one element from the stack following FIFO principle
void *linked_stack_pop(LINKED_STACK stack)
{
LINKED_STACK_NODE node;
void *res = NULL;
if (stack->head != NULL)
{
node = stack->head;
res = node->data;
stack->head = node->next;
free (node);
}
else
{
printf("eroor about empty stack is popped");
}
return res;
}
// Returns the element at the top of the stack (PLEASE FILL IT)
void *linked_stack_top(LINKED_STACK stack);
// Returns 1 if the stack is empty, 0 otherwise (PLEASE FILL IT)
int linked_stack_is_empty(LINKED_STACK stack)
{
if
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

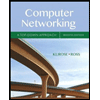
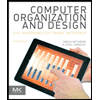
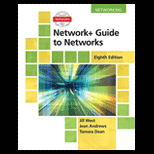
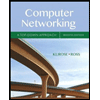
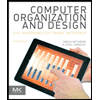
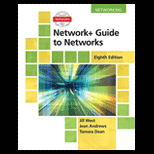
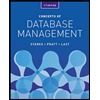
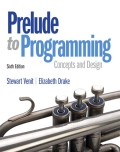
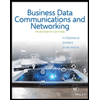