Question 5: Add a method called save to your Person class. This method will write the object out to a binary file. Use the Serializable format. Name the file accountNumber.dat where accountNumber is the credit card number from the object. ================================================== Given file Demo for Question 5 ------------------------------------------- import java.io.*; import java.util.Scanner; public class Demo { public static void main(String[] args) throws IOException, ClassNotFoundException { Person test1 = new Person("Eachelle Balderstone", 30526110612015L, 9866.30, false); Person test2 = new Person("Brand Hallam", 3573877643495486L, 9985.21, false); Person test3 = new Person("Tiphanie Oland", 5100172198301454L, 9315.15, true); test1.save(); test2.save(); test3.save(); Scanner keyboard = new Scanner(System.in); System.out.println("Enter file name"); String filename = keyboard.nextLine(); FileInputStream istream = new FileInputStream(filename); ObjectInputStream ois = new ObjectInputStream(istream); Person p = (Person)ois.readObject(); ois.close(); System.out.println(p); } } ============================================== This code below is from question 3 (part of it is mine and it worked) ------------------------------------------------------------------- import java.util.ArrayList; import java.util.Collections; public class Question3Demo { //Question3Demo was givin in Question 3 //i don't know if Question3Done code is important to the question //Look at my code at the end public static void main(String[] args) { Person test1 = new Person("Eachelle Balderstone", 30526110612015L, 9866.30, false); Person test2 = new Person("Brand Hallam", 3573877643495486L, 9985.21, false); Person test3 = new Person("Tiphanie Oland", 5100172198301454L, 9315.15, true); ArrayList list = new ArrayList<>(); list.add(test1); list.add(test2); list.add(test3); Collections.sort(list); System.out.printf("%20s%20s%10s%10s\n", "Name", "Account Number", "Balance", "Cash Back"); for (Person current : list) { System.out.println(current); } } } //this was MY code for question 3, and it passed(below) class Person implements Comparable { String name; long account; double balance; boolean back; public Person(String name, long account, double balance, boolean back) { this.name = name; this.account = account; this.balance = balance; this.back = back; } @Override public String toString() { String s; if(!back) { s ="No"; } else { s ="Yes"; } return String.format("%20s%20s%10.2f%10s", name, account, balance, s); } @Override public int compareTo(Person st) { int k=name.compareTo(st.name); if(k==0.0) return 0; else if(k<1) return -1; else return 1; } public void save() { //THIS IS WHERE I DO NOT KNOW WHAT TO DO. //"Save" is added on by Question 5 Demo code. } } ======================================================= Overall I am confused by the question and steps to follow.
Question 5: Add a method called save to your Person class. This method will write the object out to a binary file. Use the Serializable format. Name the file accountNumber.dat where accountNumber is the credit card number from the object.
==================================================
Given file Demo for Question 5
-------------------------------------------
import java.io.*;
import java.util.Scanner;
public class Demo {
public static void main(String[] args) throws IOException, ClassNotFoundException {
Person test1 = new Person("Eachelle Balderstone",
30526110612015L,
9866.30,
false);
Person test2 = new Person("Brand Hallam",
3573877643495486L,
9985.21,
false);
Person test3 = new Person("Tiphanie Oland",
5100172198301454L,
9315.15,
true);
test1.save();
test2.save();
test3.save();
Scanner keyboard = new Scanner(System.in);
System.out.println("Enter file name");
String filename = keyboard.nextLine();
FileInputStream istream = new FileInputStream(filename);
ObjectInputStream ois = new ObjectInputStream(istream);
Person p = (Person)ois.readObject();
ois.close();
System.out.println(p);
}
}
==============================================
This code below is from question 3 (part of it is mine and it worked)
-------------------------------------------------------------------
import java.util.ArrayList;
import java.util.Collections;
public class Question3Demo {
//Question3Demo was givin in Question 3
//i don't know if Question3Done code is important to the question
//Look at my code at the end
public static void main(String[] args) {
Person test1 = new Person("Eachelle Balderstone",
30526110612015L,
9866.30,
false);
Person test2 = new Person("Brand Hallam",
3573877643495486L,
9985.21,
false);
Person test3 = new Person("Tiphanie Oland",
5100172198301454L,
9315.15,
true);
ArrayList<Person> list = new ArrayList<>();
list.add(test1);
list.add(test2);
list.add(test3);
Collections.sort(list);
System.out.printf("%20s%20s%10s%10s\n", "Name", "Account Number", "Balance", "Cash Back");
for (Person current : list) {
System.out.println(current);
}
}
}
//this was MY code for question 3, and it passed(below)
class Person implements Comparable<Person>
{
String name;
long account;
double balance;
boolean back;
public Person(String name, long account, double balance, boolean back) {
this.name = name;
this.account = account;
this.balance = balance;
this.back = back;
}
@Override
public String toString() {
String s;
if(!back)
{
s ="No";
}
else
{
s ="Yes";
}
return String.format("%20s%20s%10.2f%10s", name, account, balance, s);
}
@Override
public int compareTo(Person st) {
int k=name.compareTo(st.name);
if(k==0.0)
return 0;
else if(k<1)
return -1;
else
return 1;
}
public void save() {
//THIS IS WHERE I DO NOT KNOW WHAT TO DO.
//"Save" is added on by Question 5 Demo code.
}
}
=======================================================
Overall I am confused by the question and steps to follow.

Required language JAVA:
Step by step
Solved in 3 steps with 3 images

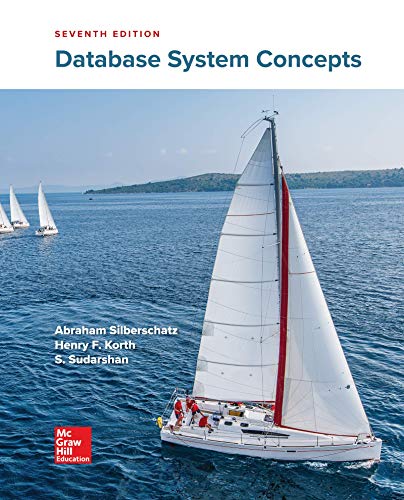
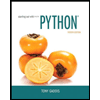
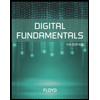
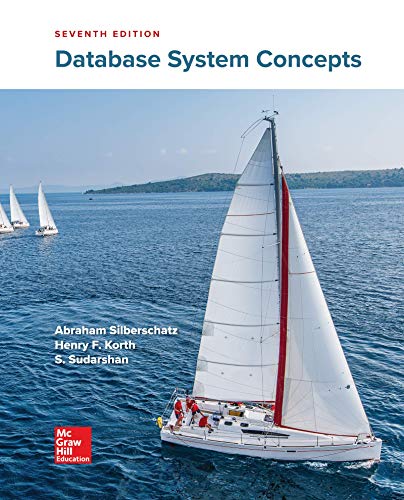
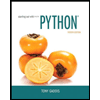
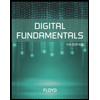
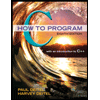
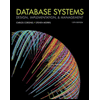
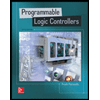