Question 4. Write a function called word_points that takes a word as a string and outputs the scrabble score of that word. Test your function in the following cell. [101]: def word_points (word): "Takes a word, returns a scrabble score for that word.''' score = return score Input In [101] score = SyntaxError: invalid syntax [102]: ## TESTS These should return True, True, True, True (word_points("a") == 1, word_points ("cab") == 7, word_points ("quadrangle") == 21, word_points("dormancy") == 16, word_points("")== 0) [102] (False, False, False, False, False)
Question 4. Write a function called word_points that takes a word as a string and outputs the scrabble score of that word. Test your function in the following cell. [101]: def word_points (word): "Takes a word, returns a scrabble score for that word.''' score = return score Input In [101] score = SyntaxError: invalid syntax [102]: ## TESTS These should return True, True, True, True (word_points("a") == 1, word_points ("cab") == 7, word_points ("quadrangle") == 21, word_points("dormancy") == 16, word_points("")== 0) [102] (False, False, False, False, False)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please help with 4 and 5.
![**Question 4:** Write a function called `word_points` that takes a word as a string and outputs the Scrabble score of that word. Test your function in the following cell.
```python
def word_points(word):
'''Takes a word, returns a scrabble score for that word.'''
score =
return score
```
Error:
```
Input In [101]
score =
^
SyntaxError: invalid syntax
```
### Tests
These should return `True, True, True, True`:
```python
(word_points("a") == 1, word_points("cab") == 7, word_points("quadrangle") == 21,
word_points("dormancy") == 16, word_points("") == 0)
```
Current return:
```
(False, False, False, False, False)
```
**Question 5:** The Scrabble game box provides a bag with tiles, each displaying a letter, and some letters are repeated. (There are 9 tiles with A, 2 with B, 1 with Z, etc.) Run the cell below to obtain the content of that bag. Not all words can be constructed with the provided tiles; for example, you cannot construct the word `pizza` because there is only one tile with Z.
Find the ten highest-scoring words not more than 10 letters long that can be built without exceeding the number of tiles of each letter in `letter_numbers`.
*Hint: For each word you need to find: (i) its length; (ii) its Scrabble score; (iii) validity (can it be constructed with the provided tiles? Write a function that checks the validity!)*
```python
# This python dictionary counts the number of tiles in the scrabble box that have each letter:
letter_numbers = { "A":9, "B":2, "C":2, "D":4, "E":12, "F":2, "G":3, "H":2, "I":9, \
"J":1, "K":1, "L":4, "M":2, "N":6, "O":8, "P":2, "Q":1, "R":6, \
"S":4, "T":6, "U":4, "V":2, "W":2, "X":1, "](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0724d636-1660-41e9-be6d-29c03d60387f%2F29cd73b0-859e-4e28-bd39-17a0fc478f85%2Fdbz7lxt_processed.png&w=3840&q=75)
Transcribed Image Text:**Question 4:** Write a function called `word_points` that takes a word as a string and outputs the Scrabble score of that word. Test your function in the following cell.
```python
def word_points(word):
'''Takes a word, returns a scrabble score for that word.'''
score =
return score
```
Error:
```
Input In [101]
score =
^
SyntaxError: invalid syntax
```
### Tests
These should return `True, True, True, True`:
```python
(word_points("a") == 1, word_points("cab") == 7, word_points("quadrangle") == 21,
word_points("dormancy") == 16, word_points("") == 0)
```
Current return:
```
(False, False, False, False, False)
```
**Question 5:** The Scrabble game box provides a bag with tiles, each displaying a letter, and some letters are repeated. (There are 9 tiles with A, 2 with B, 1 with Z, etc.) Run the cell below to obtain the content of that bag. Not all words can be constructed with the provided tiles; for example, you cannot construct the word `pizza` because there is only one tile with Z.
Find the ten highest-scoring words not more than 10 letters long that can be built without exceeding the number of tiles of each letter in `letter_numbers`.
*Hint: For each word you need to find: (i) its length; (ii) its Scrabble score; (iii) validity (can it be constructed with the provided tiles? Write a function that checks the validity!)*
```python
# This python dictionary counts the number of tiles in the scrabble box that have each letter:
letter_numbers = { "A":9, "B":2, "C":2, "D":4, "E":12, "F":2, "G":3, "H":2, "I":9, \
"J":1, "K":1, "L":4, "M":2, "N":6, "O":8, "P":2, "Q":1, "R":6, \
"S":4, "T":6, "U":4, "V":2, "W":2, "X":1, "
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
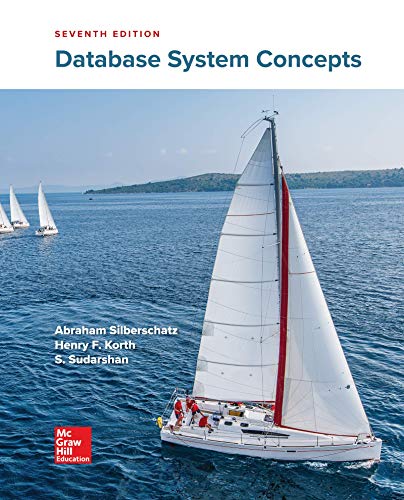
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
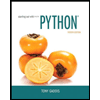
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
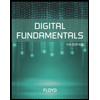
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
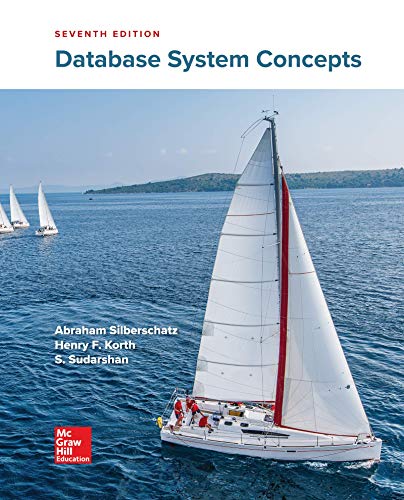
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
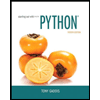
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
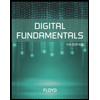
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
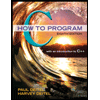
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
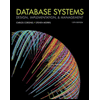
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
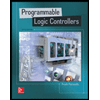
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education