Question #4: Use for statement to write a C program that asks the user to enter a positive integer number n. Then the program prints the following sequence: n , (n - 3) , (n – 6) , (n – 9), ……. , till the smallest non-negative number Example: If the user entered 14, the program should print the sequence: 14 , 11 , 8 , 5 , 2 , If the user entered 12, the program should print the sequence: 12 , 9 , 6 , 3 , 0 , #include int main(void) { //Declare required variables //read a positive integer number n from the keyboard //use for loop to generate and print the sequence printf("< Your name >\n"); printf("< Your ID >\n"); return 0; } Question4.c Output Screenshot Question #5: Write a C program that reads real numbers from a data file named "numbers.txt", one-by-one. For each number, the program checks whether the number is positive or negative, and writes only the positive numbers to a text file named "result.txt". The program must use the necessary code to handle File not found/not created errors. Below are examples of the input and output files 3.14 -25.5 -22.7 10.25 20.0 -18.2 3.14 10.25 20.0 numbers.txt Results.txt #include int main(void) { //Declare required variables //open required files /*executable statements for: 1. reading a number from input file 2. writing only the positive numbers to output file The above 2 steps are repeated as long as the input file has more numbers */ //close the files printf("< Your name >\n"); printf("< Your ID >\n"); return 0; } Question5.c Output Screenshot
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Question #4:
Use for statement to write a C program that asks the user to enter a positive integer number n. Then the program prints the following sequence:
n , (n - 3) , (n – 6) , (n – 9), ……. , till the smallest non-negative number
Example:
If the user entered 14, the program should print the sequence:
14 , 11 , 8 , 5 , 2 ,
If the user entered 12, the program should print the sequence:
12 , 9 , 6 , 3 , 0 ,
#include <stdio.h> int main(void) { //Declare required variables
//read a positive integer number n from the keyboard
//use for loop to generate and print the sequence
printf("< Your name >\n"); printf("< Your ID >\n"); return 0; }
|
Question4.c
|
|
Output Screenshot
|
Question #5:
Write a C program that reads real numbers from a data file named "numbers.txt", one-by-one.
For each number, the program checks whether the number is positive or negative, and writes only the positive numbers to a text file named "result.txt".
The program must use the necessary code to handle File not found/not created errors.
Below are examples of the input and output files
3.14 -25.5 -22.7 10.25 20.0 -18.2 |
|
3.14 10.25 20.0
|
numbers.txt |
|
Results.txt |
#include <stdio.h> int main(void) { //Declare required variables
//open required files
/*executable statements for: 1. reading a number from input file 2. writing only the positive numbers to output file The above 2 steps are repeated as long as the input file has more numbers */
//close the files
printf("< Your name >\n"); printf("< Your ID >\n"); return 0; }
|
Question5.c
|
|
Output Screenshot
|

Step by step
Solved in 2 steps with 2 images

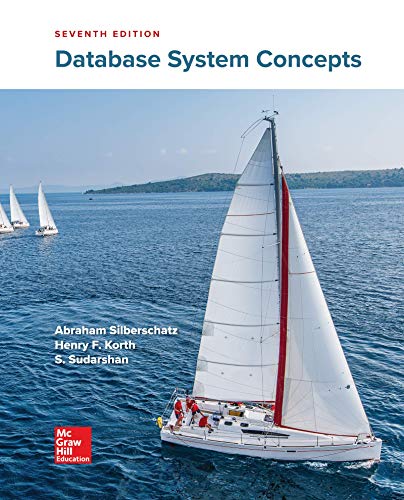
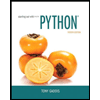
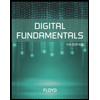
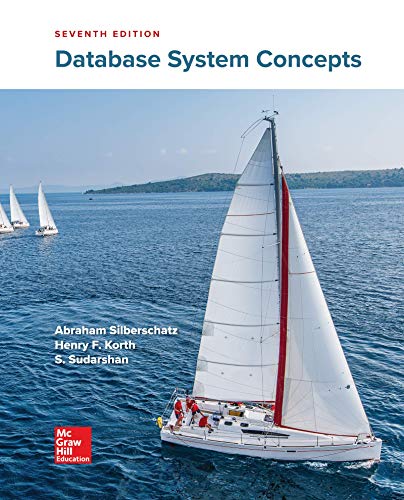
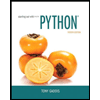
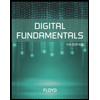
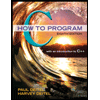
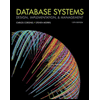
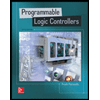