Question 3 Answer this question using ONLY ONE .cpp file. Write a complete program based on the following requirements and sample output given. (a) Create constant global variable GLOBAL_CONST with value 4. (b) Create a class named Skills with the following members: i. Private data members: • A float type array score with 4 elements • A string type array name with 4 elements ii. Public member functions: • A default constructor that sets the elements of name[i]with these strings: "Strength" • Declare class Avatar as a friend "Agility" "Speed" "Endurance" (c) Create a class name Avatar with the following members: i. Private data members: • A string type variable name • A Skills class object s ii. Public member functions: • void setName(...) This function receives a string value from main() as argument and sets to the name data member • void setSkills() This function displays the "Enter skills scores (0-50)" and then prompts the user to enter the scores for each skill as shown in the sample output, and store in the score array of s. Note: You can use a for loop to loop through the elements of name[i] to print the skill names and score[i] to set their scores in s. • string getName() Accessor function to return name. • void displayChart() This function displays the scores in a chart form, as shown in the sample output. Note: You can use nested loops to do the display. The outer loop to go through the name[i] of s to print the skill names, then the inner loop to go through the score[i] values of s to print out the symbol "=". (d) In the main(): i. Declare variables wherever necessary. Create an array object of class Avatar with 2 elements. Display the "Character Skills Chart" header. Use a loop: • Prompt the user to enter their Avatar's name. • Call setName (...) of the object array element with the user entered name as argument. • Call setSkills() and displayChart() functions of the object array element. ii. iii. iv.
Question 3 Answer this question using ONLY ONE .cpp file. Write a complete program based on the following requirements and sample output given. (a) Create constant global variable GLOBAL_CONST with value 4. (b) Create a class named Skills with the following members: i. Private data members: • A float type array score with 4 elements • A string type array name with 4 elements ii. Public member functions: • A default constructor that sets the elements of name[i]with these strings: "Strength" • Declare class Avatar as a friend "Agility" "Speed" "Endurance" (c) Create a class name Avatar with the following members: i. Private data members: • A string type variable name • A Skills class object s ii. Public member functions: • void setName(...) This function receives a string value from main() as argument and sets to the name data member • void setSkills() This function displays the "Enter skills scores (0-50)" and then prompts the user to enter the scores for each skill as shown in the sample output, and store in the score array of s. Note: You can use a for loop to loop through the elements of name[i] to print the skill names and score[i] to set their scores in s. • string getName() Accessor function to return name. • void displayChart() This function displays the scores in a chart form, as shown in the sample output. Note: You can use nested loops to do the display. The outer loop to go through the name[i] of s to print the skill names, then the inner loop to go through the score[i] values of s to print out the symbol "=". (d) In the main(): i. Declare variables wherever necessary. Create an array object of class Avatar with 2 elements. Display the "Character Skills Chart" header. Use a loop: • Prompt the user to enter their Avatar's name. • Call setName (...) of the object array element with the user entered name as argument. • Call setSkills() and displayChart() functions of the object array element. ii. iii. iv.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
c++

Transcribed Image Text:SAMPLE OUTPUT:
~~~~~
~~
Character Skills Chart
Enter Avatar Name: Korra
Enter skills scores (0-50)
Strength: 45
Agility: 37
Speed: 29
Endurance: 16
Korra's skills chart.
Strength:
Agility:
Speed:
Endurance:
============>
======>
=====>
=====>
Enter Avatar Name: Roku
Enter skills scores (0-50)
Strength: 37
Agility: 47
Speed: 28
Endurance: 33
Roku's skills chart.
Strength:
Agility:
Speed:
==>
=====>
=======>
Endurance:
======>
![Question 3
Answer this question using ONLY ONE .cpp file.
Write a complete program based on the following requirements and sample output given.
(a) Create constant global variable GLOBAL_CONST with value 4.
(b) Create a class named Skills with the following members:
i.
Private data members:
• A float type array score with 4 elements
• A string type array name with 4 elements
ii.
Public member functions:
• A default constructor that sets the elements of name[i] with these strings:
"Strength"
• Declare class Avatar as a friend
"Agility"
"Speed"
"Endurance"
(c) Create a class name Avatar with the following members:
i.
Private data members:
• A string type variable name
• A Skills class object s
ii.
Public member functions:
• void setName(...)
This function receives a string value from main() as argument and sets to the
name data member
• void setSkills()
This function displays the "Enter skills scores (0-50)" and then
prompts the user to enter the scores for each skill as shown in the sample output,
and store in the score array of s.
Note: You can use a for loop to loop through the elements of name[i] to print
the skill names and score[i] to set their scores in s.
• string getName()
Accessor function to return name.
• void displayChart()
This function displays the scores in a chart form, as shown in the sample output.
Note: You can use nested loops to do the display. The outer loop to go through
the name[i] of s to print the skill names, then the inner loop to go through
the score[i] values of s to print out the symbol "=".
(d) In the main():
i.
Declare variables wherever necessary.
Create an array object of class Avatar with 2 elements.
Display the "Character Skills Chart" header.
Use a loop:
• Prompt the user to enter their Avatar's name.
• Call setName(...) of the object array element with the user entered name as
ii.
iii.
iv.
argument.
• Call setSkills() and displayChart()functions of the object array element.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7851d256-4545-4725-85b8-f6d36c1b8a0d%2F5aaa1475-e144-41ce-9cab-bf87e9a131cd%2F7l42sw_processed.png&w=3840&q=75)
Transcribed Image Text:Question 3
Answer this question using ONLY ONE .cpp file.
Write a complete program based on the following requirements and sample output given.
(a) Create constant global variable GLOBAL_CONST with value 4.
(b) Create a class named Skills with the following members:
i.
Private data members:
• A float type array score with 4 elements
• A string type array name with 4 elements
ii.
Public member functions:
• A default constructor that sets the elements of name[i] with these strings:
"Strength"
• Declare class Avatar as a friend
"Agility"
"Speed"
"Endurance"
(c) Create a class name Avatar with the following members:
i.
Private data members:
• A string type variable name
• A Skills class object s
ii.
Public member functions:
• void setName(...)
This function receives a string value from main() as argument and sets to the
name data member
• void setSkills()
This function displays the "Enter skills scores (0-50)" and then
prompts the user to enter the scores for each skill as shown in the sample output,
and store in the score array of s.
Note: You can use a for loop to loop through the elements of name[i] to print
the skill names and score[i] to set their scores in s.
• string getName()
Accessor function to return name.
• void displayChart()
This function displays the scores in a chart form, as shown in the sample output.
Note: You can use nested loops to do the display. The outer loop to go through
the name[i] of s to print the skill names, then the inner loop to go through
the score[i] values of s to print out the symbol "=".
(d) In the main():
i.
Declare variables wherever necessary.
Create an array object of class Avatar with 2 elements.
Display the "Character Skills Chart" header.
Use a loop:
• Prompt the user to enter their Avatar's name.
• Call setName(...) of the object array element with the user entered name as
ii.
iii.
iv.
argument.
• Call setSkills() and displayChart()functions of the object array element.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
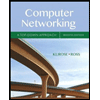
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
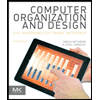
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
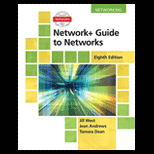
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
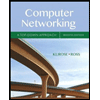
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
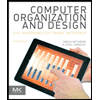
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
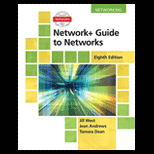
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
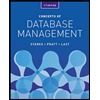
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
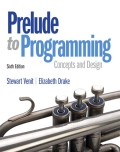
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
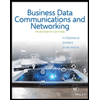
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY