QUESTION 2 Write a complete C++ program using pointer concepts to calculate the total flooring cost of a house that has several rooms. Only one type of flooring is provided which in this case is linoleum flooring. Linoleums (sort of tiles) come with several colors, dimensions, and prices. Therefore, the program should provide a feature that allows the customer to choose the type of linoleum he or she prefers. Each linoleum has a code representing its color, dimension, and price. For example, the code W08100150 represents that the linoleum's a) color is white (i.e., taken from the first character) b) width is 8 inches (ie., taken from the second and third characters) c) length is 10 inches (i.e., taken from the fourth and fifth characters ) d) price is RM 1.50 (i.e., taken from the last four characters ) For convenience, you are provided the structure chart that represents how your program should function (Figure 2). Flooring Cost Calculate Cost Choose Calculate Room Linoleum Sizes Determine Number Determine Room of Room Dimensions Figure 2: Structure Chart Your program should define the following functions:
QUESTION 2 Write a complete C++ program using pointer concepts to calculate the total flooring cost of a house that has several rooms. Only one type of flooring is provided which in this case is linoleum flooring. Linoleums (sort of tiles) come with several colors, dimensions, and prices. Therefore, the program should provide a feature that allows the customer to choose the type of linoleum he or she prefers. Each linoleum has a code representing its color, dimension, and price. For example, the code W08100150 represents that the linoleum's a) color is white (i.e., taken from the first character) b) width is 8 inches (ie., taken from the second and third characters) c) length is 10 inches (i.e., taken from the fourth and fifth characters ) d) price is RM 1.50 (i.e., taken from the last four characters ) For convenience, you are provided the structure chart that represents how your program should function (Figure 2). Flooring Cost Calculate Cost Choose Calculate Room Linoleum Sizes Determine Number Determine Room of Room Dimensions Figure 2: Structure Chart Your program should define the following functions:
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%

Transcribed Image Text:QUESTION 2
Write a complete C++ program using pointer concepts to calculate the total flooring cost of a
house that has several rooms. Only one type of flooring is provided which in this case is
linoleum flooring. Linoleums (sort of tiles) come with several colors, dimensions, and prices.
Therefore, the program should provide a feature that allows the customer to choose the type of
linoleum he or she prefers. Each linoleum has a code representing its color, dimension, and
price. For example, the code W08100150 represents that the linoleum's
a) color is white (i.e., taken from the first character)
b) width is 8 inches (i.e., taken from the second and third characters)
c) length is 10 inches (i.e., taken from the fourth and fifth characters )
d) price is RM 1.50 (i.e., taken from the last four characters )
For convenience, you are provided the structure chart that represents how your program should
function (Figure 2).
Flooring Cost
Choose
Calculate Room
Calculate
Linoleum
Sizes
Cost
Determine Number
Determine Room
of Room
Dimensions
Figure 2: Structure Chart
Your program should define the following functions:

Transcribed Image Text:a) readRoomsSize. This function will read the width and length of each room (which are
obtained from the user), and calculate the area size of the room. The size should be stored
in a dynamic array. The function should return a pointer that points to the array of rooms'
sizes.
b) extractLinolnfo. This function will extract the width, length, and price of a linoleum from
the linoleum code.
The output of the program should be printed into a file. Figure 3 shows an example run of the
program. Note that the text in italic indicates input entered by the user. Figure 4 shows the
output file produced.
What is the code of linoleum to use?: W08100150
How many rooms to floor?: 4
Enter the width and length of each room:
Room 1 -> 20.5 18.0
Room +2 -> 10.0 12.5
Room +3 -> 10.0 12.5
Room +4 -> 09.0 12.0
The flooring cost has been written into the output file
Figure 3: Example Run
Linoleum Size: 0.67 Feet x 0.83 Feet
Linoleum Price: RM 1.50 per piece
Room No.
Size (sqft)
Number of Linoleum (pieces)
665
Cost
1
369.00
RM 997.50
2
125.00
225
RM 337.50
125.00
225
RM 337.50
108.00
195
RM 292.50
Total Linole um Required: 1310 Pieces
Total Cost: RM 1965.00
Figure 4: Example Output File
Note:
The number of linoleum is calculated as Room Size / Linoleum Size. It should be
rounded up to the next nearest integer. For example, 12.1 pieces should be considered
as 13 pieces.
1 foot is equivalent to 12 inches
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 7 images

Recommended textbooks for you
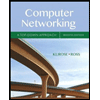
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
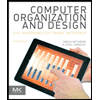
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
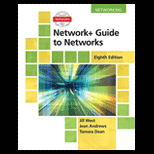
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
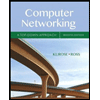
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
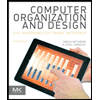
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
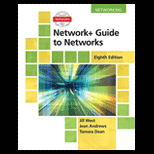
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
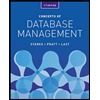
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
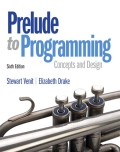
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
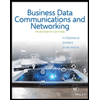
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY