Question #1: Write a C program that implements the following pseudocode: 1. Input an integer number 2. If the number is odd, then a. Add 1 to it b. Divide it by 2 c. Print the result 3. Else if the number is even, then a. Multiply it by 2 b. Print the result 4. Print your name and ID #include int main(void) { //Declare required variables //excitable statements printf("< Your name >\n"); printf("< Your ID >\n"); return 0; } question1.c Output Screenshot Question #2: Rewrite the following C program using if-else statements. #include int main(void){ int score; printf("Please enter a score (between 100 and 0)> "); scanf("%d" , &score); score = score - (score%10) ; switch(score){ case 100: printf("Extraordinary\n"); break; case 90: case 80: printf("Excellent\n"); break; case 70: case 60: case 50: printf("Not bad\n"); break; case 40: case 30: case 20: case 10: case 0: printf("Weak\n"); break; default: printf("Invalid input!\n"); } printf("< Your name >\n"); printf("< Your ID >\n"); return 0; } Question2-1.c //Question2.1.c using if-else statements Question2-2.c Output Screenshot Question #3: Write a C program that repeatedly asks the user to enter real numbers from the keyboard then it calculates and prints the average of the entered numbers. The program continuously asks the user till the user responds by ‘N’. #include int main(void) { //Declare required variables //code for reading real numbers continuously till the user responds by ‘N’ //code for calculating the average of the entered numbers and printing it printf("< Your name >\n"); printf("< Your ID >\n"); return 0; } Question3.c Output Screenshot Question #4: Use for statement to write a C program that asks the user to enter a positive integer number n. Then the program prints the following sequence: n , (n - 3) , (n – 6) , (n – 9), ……. , till the smallest non-negative number Example: If the user entered 14, the program should print the sequence: 14 , 11 , 8 , 5 , 2 , If the user entered 12, the program should print the sequence: 12 , 9 , 6 , 3 , 0 , #include int main(void) { //Declare required variables //read a positive integer number n from the keyboard //use for loop to generate and print the sequence printf("< Your name >\n"); printf("< Your ID >\n"); return 0; } Question4.c Output Screenshot
Question #1:
Write a C program that implements the following pseudocode:
1. Input an integer number 2. If the number is odd, then a. Add 1 to it b. Divide it by 2 c. Print the result 3. Else if the number is even, then a. Multiply it by 2 b. Print the result 4. Print your name and ID |
|
#include <stdio.h> int main(void) { //Declare required variables
//excitable statements
printf("< Your name >\n"); printf("< Your ID >\n"); return 0; } |
question1.c
|
|
Output Screenshot
|
Question #2:
Rewrite the following C program using if-else statements.
#include<stdio.h> int main(void){ int score; printf("Please enter a score (between 100 and 0)> "); scanf("%d" , &score); score = score - (score%10) ; switch(score){ case 100: printf("Extraordinary\n"); break; case 90: case 80: printf("Excellent\n"); break; case 70: case 60: case 50: printf("Not bad\n"); break; case 40: case 30: case 20: case 10: case 0: printf("Weak\n"); break; default: printf("Invalid input!\n"); } printf("< Your name >\n"); printf("< Your ID >\n"); return 0; } |
Question2-1.c
|
//Question2.1.c using if-else statements
|
Question2-2.c
|
|
Output Screenshot |
Question #3:
Write a C program that repeatedly asks the user to enter real numbers from the keyboard then it calculates and prints the average of the entered numbers. The program continuously asks the user till the user responds by ‘N’.
#include <stdio.h> int main(void) { //Declare required variables
//code for reading real numbers continuously till the user responds by ‘N’
//code for calculating the average of the entered numbers and printing it
printf("< Your name >\n"); printf("< Your ID >\n"); return 0; }
|
Question3.c
|
|
Output Screenshot
|
Question #4:
Use for statement to write a C program that asks the user to enter a positive integer number n. Then the program prints the following sequence:
n , (n - 3) , (n – 6) , (n – 9), ……. , till the smallest non-negative number
Example:
If the user entered 14, the program should print the sequence:
14 , 11 , 8 , 5 , 2 ,
If the user entered 12, the program should print the sequence:
12 , 9 , 6 , 3 , 0 ,
#include <stdio.h> int main(void) { //Declare required variables
//read a positive integer number n from the keyboard
//use for loop to generate and print the sequence
printf("< Your name >\n"); printf("< Your ID >\n"); return 0; }
|
Question4.c
|
|
Output Screenshot
|

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

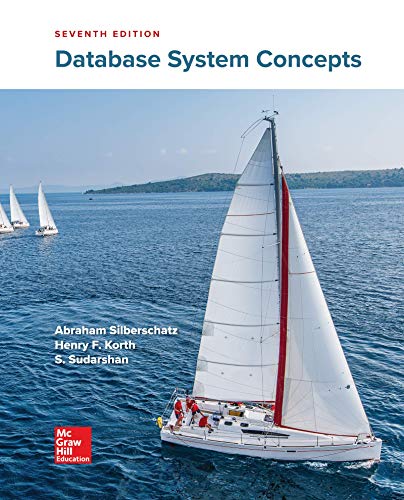
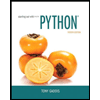
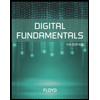
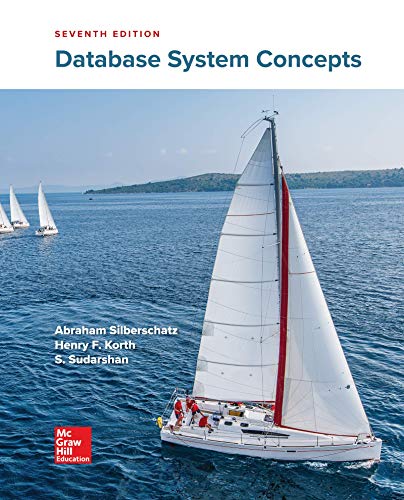
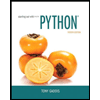
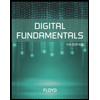
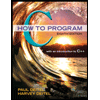
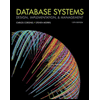
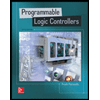