Q5: a- create a class with four integer member variables x, y, z and w; it also has the following member functions: parameterized constructor to set the values of the private variables. friend function to calculate and return the value of p as follows: (5x + y – z)3 хуz |x – y| if 1
write in c++


#include <iostream>
#include<cmath>
using namespace std;
//creating class
class Calculate{
//declaring private variables
private:
int x;
int y;
int z;
int w;
public:
//create constructor and set values
Calculate(int a,int b,int c,int d){
x=a;
y=b;
z=c;
w=d;
}
//creating friend function
friend float calculateP(Calculate &c);
};
//define function
float calculateP(Calculate &c){
float p;
//check if w in range [1-5]
if(c.w>=1 && c.w<=5){
//if yes find its value
p=(5*(c.x)+c.y-c.z);
p=p*p*p;
}
//check if w in range [6-10]
else if(c.w>=6 && c.w<=10){
//if yes find its value
p=sqrt(c.x*c.y*c.z);
}
//other wise calculate default value
else{
p=abs(c.x-c.y)/(float)c.z;
}
return p;
}
int main()
{
//create 3 calculate object
Calculate c1(1,2,3,4);
Calculate c2(2,3,4,6);
Calculate c3(2,3,4,11);
// call function and print result
cout<<"Value of p : "<<calculateP(c1)<<"\n";
cout<<"Value of p : "<<calculateP(c2)<<"\n";
cout<<"Value of p : "<<calculateP(c3)<<"\n";
return 0;
}
Step by step
Solved in 3 steps with 3 images

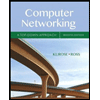
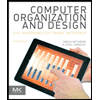
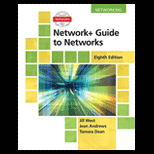
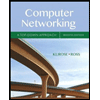
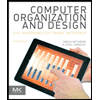
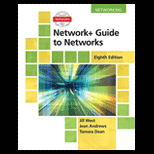
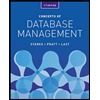
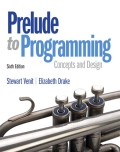
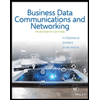