Python splitting code(Second picture) into as many functions as possible so that can run pytest for this program. How to put line "guess = input("\nEnter a letter or word:") and guess = guess.upper()" in def main(): part?
Python splitting code(Second picture) into as many functions as possible so that can run pytest for this program. How to put line "guess = input("\nEnter a letter or word:") and guess = guess.upper()" in def main(): part?
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Python splitting code(Second picture) into as many functions as possible so that can run pytest for this program.
How to put line "guess = input("\nEnter a letter or word:") and guess = guess.upper()" in def main(): part?
![```python
def game(word_list, word_index):
rounds = 6
guess_letter = ""
result = False
while rounds > 0:
word = word_list[word_index]
failed = 0
for char in word:
if char in guess_letter:
print(char, end="")
else:
print("_", end="")
failed += 1
guess = input("\nEnter a letter or word:")
guess = guess.upper()
if (failed == 1) or (guess == word):
print("\nYou win!")
result = True
break
if len(guess) == 1:
guess_letter += guess
rounds -= 1
if guess_letter.count(guess) > 1:
print("\nYou've already guessed that letter!")
# If the user guesses a letter they have already guessed.
if guess not in word:
guess_letter = "".join(set(guess_letter))
print("\nYour guess so far: " + guess_letter)
if rounds == 0:
print("\nYou lose! The word was " + word)
break
# Move on to the next round
return result
```
### Explanation
This is a Python function designed for a word guessing game. Here is a step-by-step explanation of how it works:
1. **Initialization**:
- `rounds` is set to 6, indicating the maximum number of allowed incorrect attempts.
- `guess_letter` is initialized as an empty string to store guessed letters.
- `result` is initialized as `False`.
2. **While Loop**:
- This loop continues as long as there are remaining `rounds`.
3. **Word Selection**:
- `word` is selected from `word_list` using `word_index`.
4. **Letter Checking**:
- For each character in `word`, it checks if the character is in `guess_letter`.
- If true, the character is displayed; otherwise, an underscore (`_`) is displayed, and a `failed` counter is incremented.
5. **User Input**:
- Prompts the user to enter a letter or a word and converts the input to uppercase.
6. **Win Condition**:
- If all letters are guessed (`failed == 1`) or the entire word is guessed](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F39628135-d8f9-472b-a48d-a4792a06b79c%2F0c2ed0eb-2bf0-4a12-bfb5-4929c4502fbb%2Fd2irql_processed.png&w=3840&q=75)
Transcribed Image Text:```python
def game(word_list, word_index):
rounds = 6
guess_letter = ""
result = False
while rounds > 0:
word = word_list[word_index]
failed = 0
for char in word:
if char in guess_letter:
print(char, end="")
else:
print("_", end="")
failed += 1
guess = input("\nEnter a letter or word:")
guess = guess.upper()
if (failed == 1) or (guess == word):
print("\nYou win!")
result = True
break
if len(guess) == 1:
guess_letter += guess
rounds -= 1
if guess_letter.count(guess) > 1:
print("\nYou've already guessed that letter!")
# If the user guesses a letter they have already guessed.
if guess not in word:
guess_letter = "".join(set(guess_letter))
print("\nYour guess so far: " + guess_letter)
if rounds == 0:
print("\nYou lose! The word was " + word)
break
# Move on to the next round
return result
```
### Explanation
This is a Python function designed for a word guessing game. Here is a step-by-step explanation of how it works:
1. **Initialization**:
- `rounds` is set to 6, indicating the maximum number of allowed incorrect attempts.
- `guess_letter` is initialized as an empty string to store guessed letters.
- `result` is initialized as `False`.
2. **While Loop**:
- This loop continues as long as there are remaining `rounds`.
3. **Word Selection**:
- `word` is selected from `word_list` using `word_index`.
4. **Letter Checking**:
- For each character in `word`, it checks if the character is in `guess_letter`.
- If true, the character is displayed; otherwise, an underscore (`_`) is displayed, and a `failed` counter is incremented.
5. **User Input**:
- Prompts the user to enter a letter or a word and converts the input to uppercase.
6. **Win Condition**:
- If all letters are guessed (`failed == 1`) or the entire word is guessed
![```python
def main():
rounds = 6
word_list = ['APPLE', 'OBVIOUS', 'XYLOPHONE']
word_index = 0
win = 0
while word_index < 3:
if game(word_list, word_index) is True:
win += 1
word_index += 1
print("You won " + str(win) + " out of 3")
# When the user has played all 3 rounds
```
**Explanation:**
This code is a simple Python script simulating a word game. Here's a breakdown of its components:
- **Function Definition**: The `main()` function contains the game logic.
- **Variables**:
- `rounds` is initialized to 6, though it is not used elsewhere in the code.
- `word_list` is a list containing three strings: `'APPLE'`, `'OBVIOUS'`, and `'XYLOPHONE'`.
- `word_index` is a counter initialized to 0, used to iterate over `word_list`.
- `win` counts the number of successful game plays, initialized to 0.
- **Loop**: A `while` loop runs as long as `word_index` is less than 3. This ensures the loop iterates over each word in `word_list`.
- **Conditional Statement**: If `game(word_list, word_index)` returns `True` for a word, the `win` counter is incremented.
- **Output**: After the loop concludes, a message prints the number of rounds won out of 3.
- **Comment**: Provides a note that output occurs after all three rounds have been played.
**Note**: The function `game(word_list, word_index)` is assumed to be defined elsewhere in the program and determines if a round is won based on the words.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F39628135-d8f9-472b-a48d-a4792a06b79c%2F0c2ed0eb-2bf0-4a12-bfb5-4929c4502fbb%2Fbjntn0c_processed.png&w=3840&q=75)
Transcribed Image Text:```python
def main():
rounds = 6
word_list = ['APPLE', 'OBVIOUS', 'XYLOPHONE']
word_index = 0
win = 0
while word_index < 3:
if game(word_list, word_index) is True:
win += 1
word_index += 1
print("You won " + str(win) + " out of 3")
# When the user has played all 3 rounds
```
**Explanation:**
This code is a simple Python script simulating a word game. Here's a breakdown of its components:
- **Function Definition**: The `main()` function contains the game logic.
- **Variables**:
- `rounds` is initialized to 6, though it is not used elsewhere in the code.
- `word_list` is a list containing three strings: `'APPLE'`, `'OBVIOUS'`, and `'XYLOPHONE'`.
- `word_index` is a counter initialized to 0, used to iterate over `word_list`.
- `win` counts the number of successful game plays, initialized to 0.
- **Loop**: A `while` loop runs as long as `word_index` is less than 3. This ensures the loop iterates over each word in `word_list`.
- **Conditional Statement**: If `game(word_list, word_index)` returns `True` for a word, the `win` counter is incremented.
- **Output**: After the loop concludes, a message prints the number of rounds won out of 3.
- **Comment**: Provides a note that output occurs after all three rounds have been played.
**Note**: The function `game(word_list, word_index)` is assumed to be defined elsewhere in the program and determines if a round is won based on the words.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
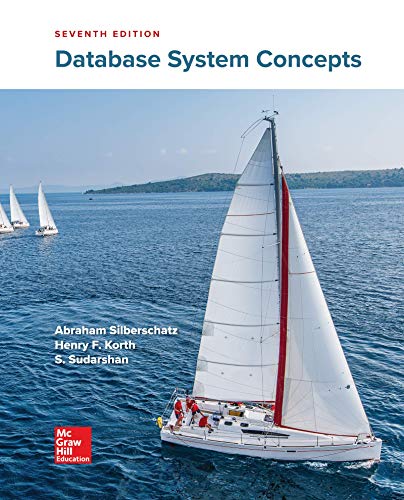
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
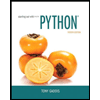
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
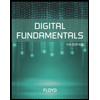
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
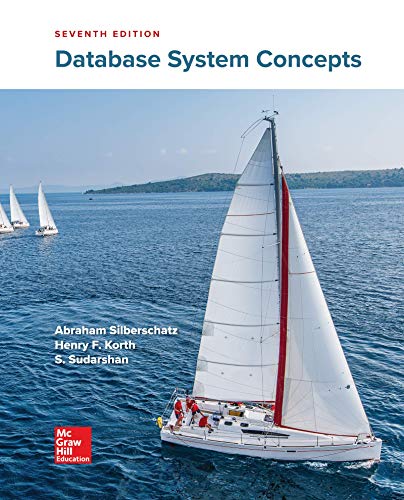
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
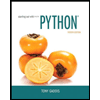
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
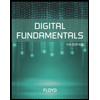
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
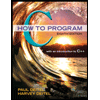
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
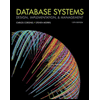
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
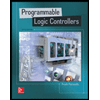
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education