Python Modules Create the module file of a program that is intended to analyze a user’s input for any palindromes. PROGRAM DESIGN The module should have two functions: palindrome_check() and print_output(). The palindrome_check() function should accept one parameter named inp which is the user’s input, and count the total number of palindromes in the input string. This function should return the total number of palindromes. This number should be passed back to the main.py file where it would be passed to the print_output() function. This function should print the appropriate output as stated in the sample outputs. For this task, you’re only tasked to create the modules.py file which is imported by the given main.py file. Name this exercise as modules.py . You’re only allowed to create modules.py and not edit main.py note: palindromes for this activity aren’t case sensitive. INPUT The input is a string of words that may or may not contain palindromes. OUTPUT Once the user enters the input, the program should be able to pass the input to palindrome_check(). Here the program should traverse through the words in the passed input and count the total number of palindromes in the input. This count would then be returned to the main file, and passed to the print_output() function where the following outputs would be printed – depending on the total count. (see sample outputs). SAMPLE INPUT Hello there 02022020 Hello anna madam we are here since noon time wow you are good at this I mean look at your stats you have to level up your wow character if you want to pop off sis Hannah is the name of the nun who talked to mom SAMPLE OUTPUT There were no palindromes. There was one palindrome. There were 3 palindromes. There were 2 palindromes. There were 3 palindromes. There were 4 palindromes. main.py contents: from modules import palindrome_check as pc, print_output as po inp = input() count = pc(inp) po(count)
Python Modules
Create the module file of a program that is intended to analyze a user’s input for any
palindromes.
PROGRAM DESIGN
The module should have two functions: palindrome_check() and print_output(). The
palindrome_check() function should accept one parameter named inp which is the user’s
input, and count the total number of palindromes in the input string. This function should
return the total number of palindromes. This number should be passed back to the main.py
file where it would be passed to the print_output() function. This function should print the
appropriate output as stated in the sample outputs. For this task, you’re only tasked to create
the modules.py file which is imported by the given main.py file.
Name this exercise as modules.py . You’re only allowed to create modules.py and not edit
main.py
note: palindromes for this activity aren’t case sensitive.
INPUT
The input is a string of words that may or may not contain palindromes.
OUTPUT
Once the user enters the input, the program should be able to pass the input to
palindrome_check(). Here the program should traverse through the words in the passed
input and count the total number of palindromes in the input. This count would then be
returned to the main file, and passed to the print_output() function where the following
outputs would be printed – depending on the total count. (see sample outputs).
SAMPLE INPUT
Hello there
02022020
Hello anna madam we are here since noon time
wow you are good at this I mean look at your stats
you have to level up your wow character if you want to pop off
sis Hannah is the name of the nun who talked to mom
SAMPLE OUTPUT
There were no palindromes.
There was one palindrome.
There were 3 palindromes.
There were 2 palindromes.
There were 3 palindromes.
There were 4 palindromes.
main.py contents:
from modules import palindrome_check as pc, print_output as po
inp = input()
count = pc(inp)
po(count)


Step by step
Solved in 4 steps with 4 images

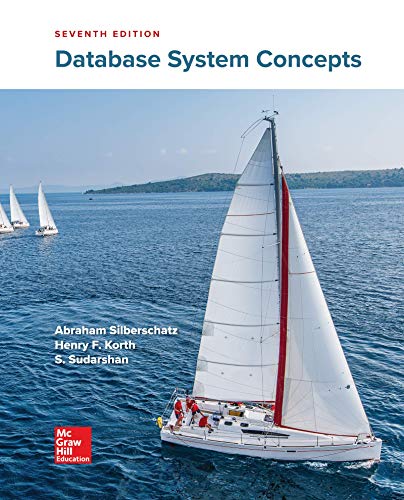
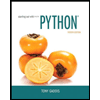
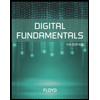
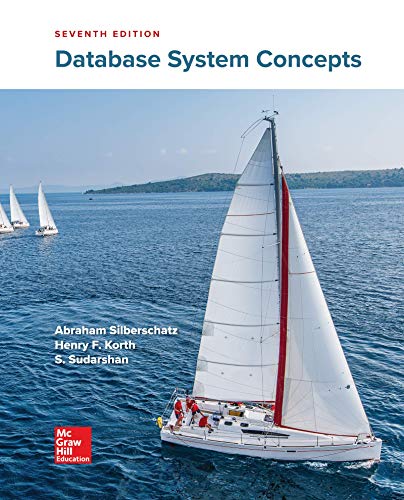
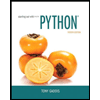
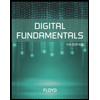
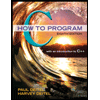
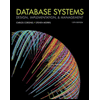
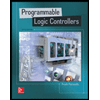