**PYTHON*** I need a program written that can do the following: use the list of tuples given as a starting point for data. option 1 should print the full list of tuples, and the printing needs to be formatted so that the data lines up neatly. option 2 needs to work so that a user can enter the name, destination, and mileage for a new trip, and have that data be added as a tuple to the list. options 3 need to allow filtering the list by name option 4 just needs to allow the program to end if the user does not enter 1, 2, 3 or 4 as an option, you need to print an error message and have them re-enter their choice. there needs to be a main function that kicks things off, and you need to write a function to print the whole list, a function that allows inputting a new trip, and a function that filters by name and prints all the trips for that given name. These functions are called when the user types in a given option from the menu. if you add a new trip, everything should still work The menu options must be: Program Options. Display all trips Input new trip Display trips by Name Exit Each of the first 3 options should call a function to accomplish the proper task. Tuples: trips = [ (“alice”, ‘chicago’, 302), (“bruce”, ‘chicago’, 309), (“david”, ‘chicago’, 307), (“carol”, ‘columbus’, 212), (“alice”, ‘chicago’, 304), (“bruce”, ‘chicago’, 301), (“david”, ‘columbus’, 215), (“alice”, ‘chicago’, 302), (“carol”, ‘chicago’, 305), (“bruce”, ‘chicago’, 304), (“carol”, ‘columbus’, 218), (“alice”, ‘columbus’, 217), (“carol”, ‘chicago’, 309) (“david”, columbus, 219)
***PYTHON*** I need a
- use the list of tuples given as a starting point for data.
-
option 1 should print the full list of tuples, and the printing needs to be
formatted so that the data lines up neatly.
-
option 2 needs to work so that a user can enter the name, destination,
and mileage for a new trip, and have that data be added as a tuple to the
list.
-
options 3 need to allow filtering the list by name
- option 4 just needs to allow the program to end
-
if the user does not enter 1, 2, 3 or 4 as an option, you need to print an
error message and have them re-enter their choice.
-
there needs to be a main function that kicks things off, and you need to
write a function to print the whole list, a function that allows inputting a
new trip, and a function that filters by name and prints all the trips for
that given name. These functions are called when the user types in a
given option from the menu.
-
if you add a new trip, everything should still work
The menu options must be:
Program Options.
- Display all trips
- Input new trip
- Display trips by Name
- Exit
Each of the first 3 options should call a function to accomplish the proper task.
Tuples:
trips = [
(“alice”, ‘chicago’, 302),
(“bruce”, ‘chicago’, 309),
(“david”, ‘chicago’, 307),
(“carol”, ‘columbus’, 212),
(“alice”, ‘chicago’, 304),
(“bruce”, ‘chicago’, 301),
(“david”, ‘columbus’, 215),
(“alice”, ‘chicago’, 302),
(“carol”, ‘chicago’, 305),
(“bruce”, ‘chicago’, 304),
(“carol”, ‘columbus’, 218),
(“alice”, ‘columbus’, 217),
(“carol”, ‘chicago’, 309)
(“david”, columbus, 219)

Step-1: Start
Step-2: Define function display_trips(trips)
Step-2.1: Start loop for name, destination, mileage in trips
Step-2.1.1: Print Name, Destination and Mileage
Step-3: Define function input_new_trip(trips)
Step-3.1: Declare variable name and take input from the user
Step-3.2: Declare variable destination and take input from the user
Step-3.3: Declare variable mileage and take input from the user
Step-3.4: In trips append (name, destination, mileage)
Step-3.5: Print "Trip added successfully!"
Step-4: Define function display_trips_by_name(trips)
Step-4.1: Declare variable name_to_filter and take input from the user
Step-4.2: Declare variable filtered_trips and assign [(name, destination, mileage) for name, destination, mileage in trips if name == name_to_filter]
Step-4.3: If filtered_trips
Step-4.3.1: Print "Trips for (name_to_filter)"
Step-4.3.2: Call function display_trips and pass argument filtered_trips
Step-4.4: Else print "No trips found for (name_to_filter)."
Step-5: Define main function
Step-5.1: Declare variable trips and assign [
("alice", 'chicago', 302),
("bruce", 'chicago', 309),
("david", 'chicago', 307),
("carol", 'columbus', 212),
("alice", 'chicago', 304),
("bruce", 'chicago', 301),
("david", 'columbus', 215),
("alice", 'chicago', 302),
("carol", 'chicago', 305),
("bruce", 'chicago', 304),
("carol", 'columbus', 218),
("alice", 'columbus', 217),
("carol", 'chicago', 309),
("david", 'columbus', 219)
]
Step-5.2: Start loop while True
Step-5.2.1: Print "Program Options:"
Step-5.2.2: Print "1. Display all trips"
Step-5.2.3: Print "2. Input new trip"
Step-5.2.4: Print "3. Display trips by Name"
Step-5.2.5: Print "4. Exit"
Step-5.2.6: Declare variable choice and take input from the user
Step-5.2.7: If choice is equal to 1 then call function display_trips and pass argument trips
Step-5.2.8: Elif choice is equal to 2 then call function input_new_trip and pass argument trips
Step-5.2.9: Elif choice is equal to 3 then call function display_trips_by_name and pass argument trips
Step-5.2.10: Elif choice is equal to 4 then print "Exiting the program." and break
Step-5.2.11: Else print "Invalid choice. Please enter 1, 2, 3, or 4."
Step-6: if __name__ == "__main__":
Step-6.1: Call function main()
Step-7: Stop
Step by step
Solved in 4 steps with 5 images

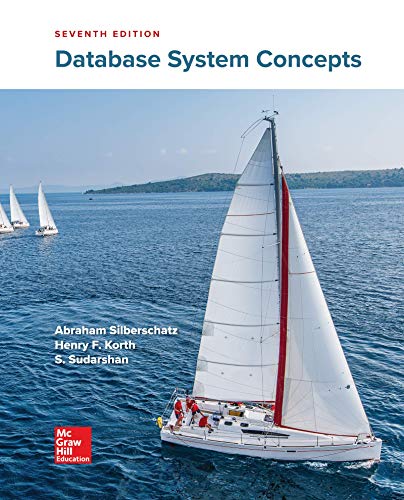
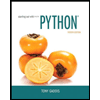
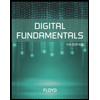
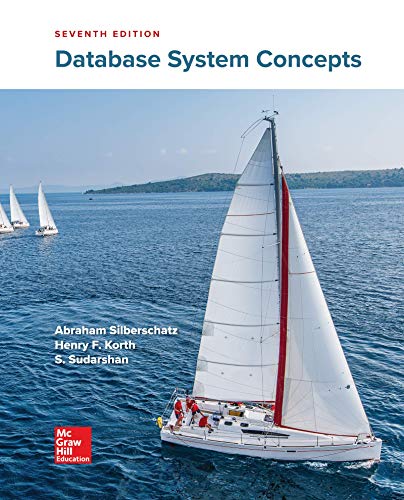
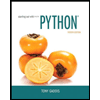
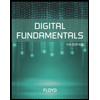
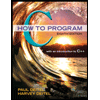
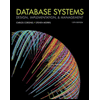
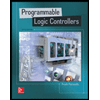