Purpose: Python parallel list implementations Program Specifications: 1. Write a program that simulates a lottery. The program should have a list of 6 integers named winning_list, with a randomly generated number in the range of 1 through 9 inclusive for each element in the list. 2. The program should ask user to enter 6 numbers and store them in another integer list named player_list. 3. The program then compares the numbers in 2 lists to find out how many numbers match. If two numbers are at the same position in both lists, it is a match. (This is using parallel list concept to compare two lists.) 4. The program output should display the winning numbers, player's numbers, and how many numbers matched. For instance, if your winning numbers are 3,5,9,1,4,7 and your player's numbers are 2,5, 7,1, 9,8, then you have two matches (5 and 1). 5 is on the second position and 1 is on the fourth position in both lists. 5. Input Validation: The guessing number entered by user must be between 1-9 inclusive. To Solve this problem, we will create the following functions. get_winning_number. get_player_number get_matches print_numbers main ● ● ● This function generates random number between 1-9, stored in the random list and return the list of 6 random numbers. This function does not have parameters and returns the random list. This function prompts the user to enter lottery guessing number and stored them into a list. This function does not have any parameters and returns the user guessing list. Make sure to validate the number, so it is in the range of 1-9 inclusive. This function compares two list position by position to see if there is a match. This function passes the random list and user guessing list. This function returns total count of matches. ● This function passes two list and the matches count and display them in the console. (See Program output) ● main function will call all the functions above.
Purpose: Python parallel list implementations Program Specifications: 1. Write a program that simulates a lottery. The program should have a list of 6 integers named winning_list, with a randomly generated number in the range of 1 through 9 inclusive for each element in the list. 2. The program should ask user to enter 6 numbers and store them in another integer list named player_list. 3. The program then compares the numbers in 2 lists to find out how many numbers match. If two numbers are at the same position in both lists, it is a match. (This is using parallel list concept to compare two lists.) 4. The program output should display the winning numbers, player's numbers, and how many numbers matched. For instance, if your winning numbers are 3,5,9,1,4,7 and your player's numbers are 2,5, 7,1, 9,8, then you have two matches (5 and 1). 5 is on the second position and 1 is on the fourth position in both lists. 5. Input Validation: The guessing number entered by user must be between 1-9 inclusive. To Solve this problem, we will create the following functions. get_winning_number. get_player_number get_matches print_numbers main ● ● ● This function generates random number between 1-9, stored in the random list and return the list of 6 random numbers. This function does not have parameters and returns the random list. This function prompts the user to enter lottery guessing number and stored them into a list. This function does not have any parameters and returns the user guessing list. Make sure to validate the number, so it is in the range of 1-9 inclusive. This function compares two list position by position to see if there is a match. This function passes the random list and user guessing list. This function returns total count of matches. ● This function passes two list and the matches count and display them in the console. (See Program output) ● main function will call all the functions above.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
In Python please

Transcribed Image Text:**Program Output:**
- **Guess 1-9 for player number 1:** 80
*Invalid number, enter 1-9: -10*
*Invalid number, enter 1-9: 6*
- **Guess 1-9 for player number 2:** 8
- **Guess 1-9 for player number 3:** 3
- **Guess 1-9 for player number 4:** 9
- **Guess 1-9 for player number 5:** 2
- **Guess 1-9 for player number 6:** 1
**Winning Numbers:** 9 1 6 8 2 2
**Player Numbers:** 6 8 3 9 2 1
**Total matches:** 1

Transcribed Image Text:**Purpose:**
Python parallel list implementations
**Program Specifications:**
1. **Write a program that simulates a lottery.** The program should have a list of 6 integers named `winning_list`, with a randomly generated number in the range of 1 through 9 inclusive for each element in the list.
2. **The program should ask the user to enter 6 numbers and store them** in another integer list named `player_list`.
3. **The program then compares the numbers in 2 lists** to find out how many numbers match. If two numbers are at the same position in both lists, it is a match. (This is using parallel list concept to compare two lists.)
4. **The program output should display the winning numbers, player’s numbers, and how many numbers matched.** For instance, if your winning numbers are 3,5,9,1,4,7 and your player’s numbers are 2,5,7,1,9,8, then you have two matches (5 and 1). 5 is on the second position and 1 is on the fourth position in both lists.
5. **Input Validation:** The guessing number entered by user must be between 1-9 inclusive.
**To Solve this problem, we will create the following functions.**
| **Function Name** | **Description** |
|-----------------------|-----------------|
| **get_winning_number** | - This function generates random numbers between 1-9, stored in the random list and returns the list of 6 random numbers.<br>- This function does not have parameters and returns the random list. |
| **get_player_number** | - This function prompts the user to enter lottery guessing numbers and stores them into a list.<br>- This function does not have any parameters and returns the user guessing list.<br>- Make sure to validate the numbers, so they are in the range of 1-9 inclusive. |
| **get_matches** | - This function compares two lists position by position to see if there is a match.<br>- This function passes the random list and user guessing list.<br>- This function returns total count of matches. |
| **print_numbers** | - This function passes two lists and the matches count and displays them in the console. |
- **main:** The main function will call all the functions above.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

Recommended textbooks for you
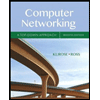
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
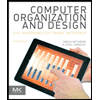
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
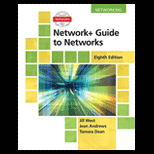
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
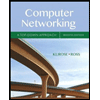
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
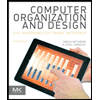
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
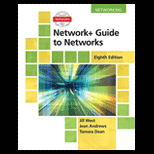
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
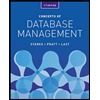
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
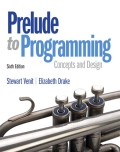
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
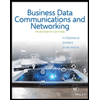
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY