} public int getLegs () { return numLegs; public boolean haswings() { } return haswings; public String getName() { return name; } te an equals method for the Insect class to compare two Insect objects to one another:
} public int getLegs () { return numLegs; public boolean haswings() { } return haswings; public String getName() { return name; } te an equals method for the Insect class to compare two Insect objects to one another:
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I need answer in java

Transcribed Image Text:### Code Example: Insect Class
Consider the following Java code segment which defines an `Insect` class:
```java
public class Insect
{
private String name;
private int numLegs;
private boolean hasWings;
public Insect(String theName, int legs, boolean wings)
{
name = theName;
numLegs = legs;
hasWings = wings;
}
public int getLegs()
{
return numLegs;
}
public boolean hasWings()
{
return hasWings;
}
public String getName()
{
return name;
}
}
```
This class represents insects with the following attributes:
- `name`: A `String` representing the name of the insect.
- `numLegs`: An `int` representing the number of legs.
- `hasWings`: A `boolean` indicating whether the insect has wings.
### Methods:
- **Constructor**: Initializes the insect's name, number of legs, and wing status.
- **`getLegs()`**: Returns the number of legs.
- **`hasWings()`**: Returns `true` if the insect has wings, otherwise `false`.
- **`getName()`**: Returns the name of the insect.
### Task:
Write an `equals` method for the `Insect` class to compare two `Insect` objects to one another:
```java
public boolean equals(Insect other)
{
// Method logic to be implemented
}
```
The `equals` method should determine if two `Insect` objects are equal based on their attributes.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
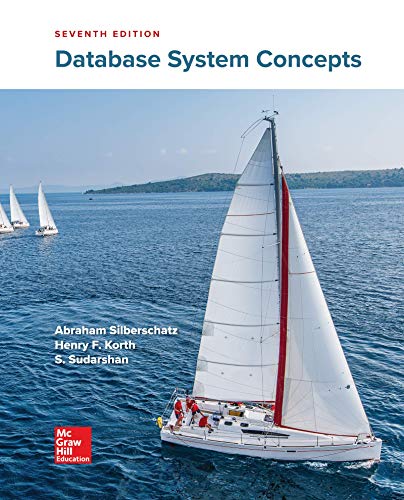
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
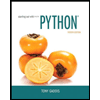
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
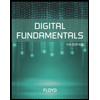
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
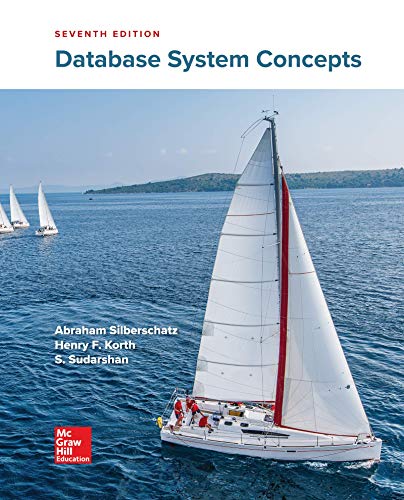
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
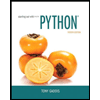
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
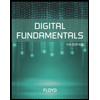
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
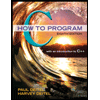
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
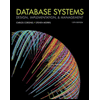
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
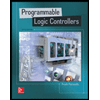
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education