public class Recursive{ public static void main(String[] args){ System.out.println("Summation of 5 System.out.println("Summation of 1 System.out.println("Summation of 10 System.out.println("Summation of 1 = System.out.println("Summation of 7 = "+summation(7)); "+summation(5)); "+summation(1)); '+summation(10)); "+summation(1)); System.out.println("The first position value in the Fibonacci Sequence is "+fibonacciSequence(1)); System.out.println("The third position value in the Fibonacci Sequence is "+fibonacciSequence(3)); System.out.println("The seventh position value in the Fibonacci Sequence is "+fibonacciSequence(7)); System.out.println("The twentith position value in the Fibonacci Sequence is "+fibonacciSequence(20)); } public static int summation(int n){ //TODO: Create a recursive method that will find the summation of n for all positive n return -1; } public static int fibonacciSequence(int positionN){ //TODO: Create a recursive method that will find the Nth position of the Fibonacci sequence return -1;
public class Recursive{ public static void main(String[] args){ System.out.println("Summation of 5 System.out.println("Summation of 1 System.out.println("Summation of 10 System.out.println("Summation of 1 = System.out.println("Summation of 7 = "+summation(7)); "+summation(5)); "+summation(1)); '+summation(10)); "+summation(1)); System.out.println("The first position value in the Fibonacci Sequence is "+fibonacciSequence(1)); System.out.println("The third position value in the Fibonacci Sequence is "+fibonacciSequence(3)); System.out.println("The seventh position value in the Fibonacci Sequence is "+fibonacciSequence(7)); System.out.println("The twentith position value in the Fibonacci Sequence is "+fibonacciSequence(20)); } public static int summation(int n){ //TODO: Create a recursive method that will find the summation of n for all positive n return -1; } public static int fibonacciSequence(int positionN){ //TODO: Create a recursive method that will find the Nth position of the Fibonacci sequence return -1;
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
![## Java Recursive Methods Example
### Overview
Below is a Java class illustrating the use of recursion to solve two common problems: summation and finding a position in the Fibonacci sequence.
### Code Explanation
#### Class: Recursive
```java
public class Recursive {
public static void main(String[] args) {
System.out.println("Summation of 5 = " + summation(5));
System.out.println("Summation of 1 = " + summation(1));
System.out.println("Summation of 10 = " + summation(10));
System.out.println("Summation of 1 = " + summation(1));
System.out.println("Summation of 7 = " + summation(7));
System.out.println("The first position value in the Fibonacci Sequence is " + fibonacciSequence(1));
System.out.println("The third position value in the Fibonacci Sequence is " + fibonacciSequence(3));
System.out.println("The seventh position value in the Fibonacci Sequence is " + fibonacciSequence(7));
System.out.println("The twentieth position value in the Fibonacci Sequence is " + fibonacciSequence(20));
}
public static int summation(int n) {
//TODO: Create a recursive method that will find the summation of n for all positive n
return -1;
}
public static int fibonacciSequence(int positionN) {
//TODO: Create a recursive method that will find the Nth position of the Fibonacci sequence
return -1;
}
}
```
### Functional Description
1. **Main Method:**
- The `main` method demonstrates calls to two recursive methods which are intended to compute the summation of positive integers and to get the Nth position value in the Fibonacci sequence.
2. **Summation Method:**
- `public static int summation(int n)`
- Intended to create a recursive approach to calculate the summation of `n`, where `n` is a positive integer.
- Current implementation returns `-1` as a placeholder.
3. **Fibonacci Sequence Method:**
- `public static int fibonacciSequence(int positionN)`
- Meant to determine the Fibonacci sequence value at position `N` using recursion.
- Current implementation returns `-1` as a placeholder.
### Learning Objective
- This example is aimed at understanding the concept of recursion in Java programming. Recursive functions call themselves with different](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa50218e1-9020-47a4-b189-6a79dd77425b%2F0d4aeb3d-11ab-457a-afc3-91d806dafd65%2Fzyvytgq_processed.png&w=3840&q=75)
Transcribed Image Text:## Java Recursive Methods Example
### Overview
Below is a Java class illustrating the use of recursion to solve two common problems: summation and finding a position in the Fibonacci sequence.
### Code Explanation
#### Class: Recursive
```java
public class Recursive {
public static void main(String[] args) {
System.out.println("Summation of 5 = " + summation(5));
System.out.println("Summation of 1 = " + summation(1));
System.out.println("Summation of 10 = " + summation(10));
System.out.println("Summation of 1 = " + summation(1));
System.out.println("Summation of 7 = " + summation(7));
System.out.println("The first position value in the Fibonacci Sequence is " + fibonacciSequence(1));
System.out.println("The third position value in the Fibonacci Sequence is " + fibonacciSequence(3));
System.out.println("The seventh position value in the Fibonacci Sequence is " + fibonacciSequence(7));
System.out.println("The twentieth position value in the Fibonacci Sequence is " + fibonacciSequence(20));
}
public static int summation(int n) {
//TODO: Create a recursive method that will find the summation of n for all positive n
return -1;
}
public static int fibonacciSequence(int positionN) {
//TODO: Create a recursive method that will find the Nth position of the Fibonacci sequence
return -1;
}
}
```
### Functional Description
1. **Main Method:**
- The `main` method demonstrates calls to two recursive methods which are intended to compute the summation of positive integers and to get the Nth position value in the Fibonacci sequence.
2. **Summation Method:**
- `public static int summation(int n)`
- Intended to create a recursive approach to calculate the summation of `n`, where `n` is a positive integer.
- Current implementation returns `-1` as a placeholder.
3. **Fibonacci Sequence Method:**
- `public static int fibonacciSequence(int positionN)`
- Meant to determine the Fibonacci sequence value at position `N` using recursion.
- Current implementation returns `-1` as a placeholder.
### Learning Objective
- This example is aimed at understanding the concept of recursion in Java programming. Recursive functions call themselves with different
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
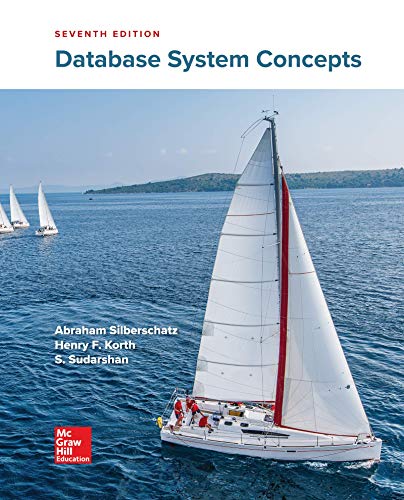
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
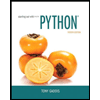
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
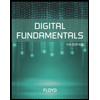
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
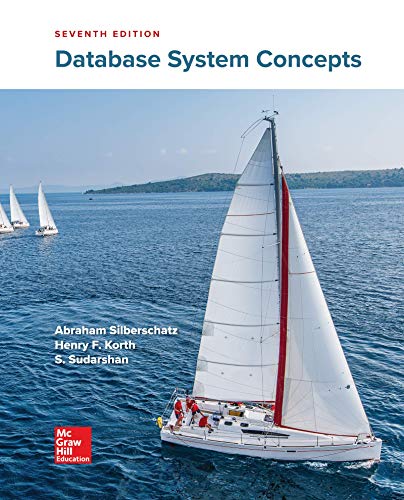
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
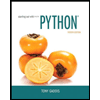
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
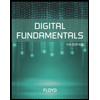
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
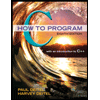
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
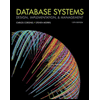
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
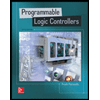
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education