public class Dog { private String name; private double weight; private static int numDogs = 0; public Dog(String name, double weight) { this.name = name; this.weight = weight; numDogs++; } public String getName() { return name; } public double getWeight() { return weight; } public static int getNumDogs() { return numDogs; } public static void increaseNumDogs() { numDogs++; } public void printGreeting() { System.out.println("Woof!"); } @Override public String toString() { return "Name: " + name + "\nWeight: " + weight; } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
(Java)
Q6
Explain the answer step-by-step and include verbal explanation. Thank you!
- Given the below class:
public class Dog {
private String name;
private double weight;
private static int numDogs = 0;
public Dog(String name, double weight) {
this.name = name;
this.weight = weight;
numDogs++;
}
public String getName() {
return name;
}
public double getWeight() {
return weight;
}
public static int getNumDogs() {
return numDogs;
}
public static void increaseNumDogs() {
numDogs++;
}
public void printGreeting() {
System.out.println("Woof!");
}
@Override public String toString() {
return "Name: " + name + "\nWeight: " + weight;
}
}
Call the printGreeting method. What is different about the way you call this method compared to the prior two methods.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

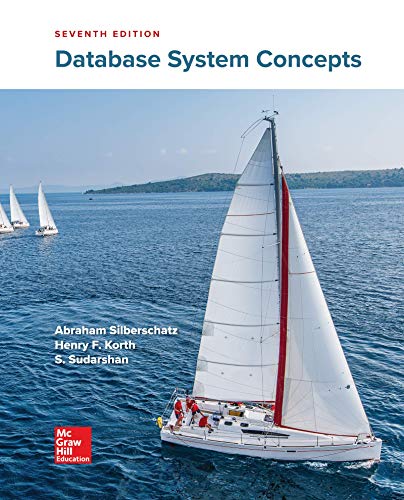
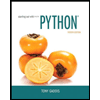
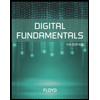
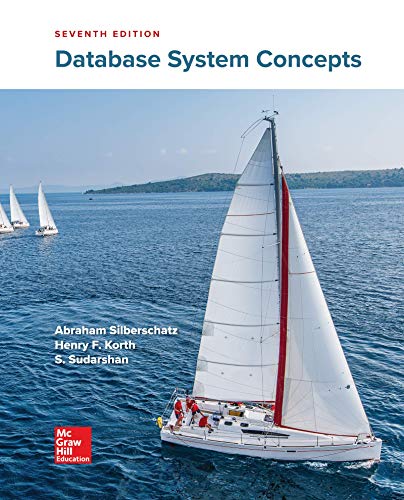
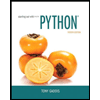
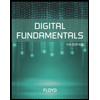
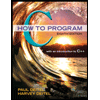
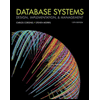
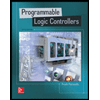