PROVIDE PSEUDOCODE, MANUAL COMPUTATION, FLOWCHART PLS THANKS! make a simple banking system. assume that the bank has three (3) tellers that take turns in serving the customers. Each customer should have at least the following parameters: Account Number, Account Name, Transaction Type (Withdraw, Deposit), Transaction Amount (in Php), and Average Transaction Time (in minutes). The program should ask first the number of customers to serve, then asks the details for each customer. Input at least 10 customer details. The program should output the transaction log of the 3 tellers. Each transaction log entry should contain the customer's account number, account name, the amount withdrawn/deposited, and the remaining account balance. Note: Each customer account must have an initial balance of Php 10,000.00.
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
modify the provided C++ code below and add this:
- PROVIDE PSEUDOCODE, MANUAL COMPUTATION, FLOWCHART PLS THANKS!
- make a simple banking system. assume that the bank has three (3) tellers that take turns in serving the customers. Each customer should have at least the following parameters: Account Number, Account Name, Transaction Type (Withdraw, Deposit), Transaction Amount (in Php), and Average Transaction Time (in minutes).
- The
program should ask first the number of customers to serve, then asks the details for each customer. Input at least 10 customer details. The program should output the transaction log of the 3 tellers. Each transaction log entry should contain the customer's account number, account name, the amount withdrawn/deposited, and the remaining account balance. - Note: Each customer account must have an initial balance of Php 10,000.00.
C++ code:
#include <iostream>
#include <cstdlib>
#include <ctime>
class student_queue {
public:
struct node {
int num;
node* prev;
node* next;
};
student_queue() {
head = nullptr;
tail = nullptr;
count = 0;
}
~student_queue() {
clear();
}
node* front() const {
return head;
}
node* back() const {
return tail;
}
void push(int num) {
node* newNode = new node;
newNode->num = num;
newNode->prev = tail;
newNode->next = nullptr;
if (tail == nullptr) {
head = newNode;
}
else {
tail->next = newNode;
}
tail = newNode;
count++;
}
void pop() {
if (head == nullptr) {
return;
}
node* oldHead = head;
head = head->next;
if (head == nullptr) {
tail = nullptr;
}
else {
head->prev = nullptr;
}
count--;
delete oldHead;
}
bool empty() const {
return count == 0;
}
int size() const {
return count;
}
void clear() {
while (head != nullptr) {
node* temp = head;
head = head->next;
delete temp;
}
tail = nullptr;
count = 0;
}
private:
node* head;
node* tail;
int count;
};
int main() {
// Create a student_queue object
student_queue q;
// Add some random values to the queue
std::srand(std::time(nullptr));
for (int i = 0; i < 10; i++) {
int num = std::rand() % 20 - 10;
q.push(num);
}
// Print the queue forward
std::cout << "Queue elements: ";
student_queue::node* cur = q.front();
while (cur != nullptr) {
std::cout << cur->num << " ";
cur = cur->next;
}
std::cout << std::endl;
// Print the front and back elements
std::cout << "Front element: " << q.front()->num << std::endl;
std::cout << "Back element: " << q.back()->num << std::endl;
// Remove some elements from the queue
std::cout << "Removing some elements from the queue..." << std::endl;
q.pop();
q.pop();
// Print the updated queue forward
std::cout << "Updated queue elements: ";
cur = q.front();
while (cur != nullptr) {
std::cout << cur->num << " ";
cur = cur->next;
}
std::cout << std::endl;
// Print the size of the queue
std::cout << "Queue size: " << q.size() << std::endl;
// Clear the queue
q.clear();
std::cout << "Queue cleared. Size is now: " << q.size() << std::endl;
return 0;
}

Step by step
Solved in 7 steps with 9 images

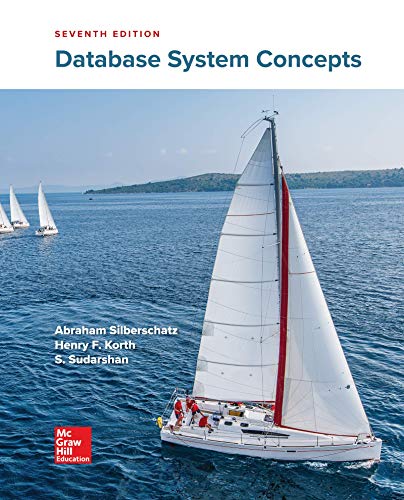
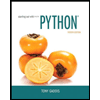
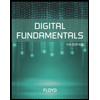
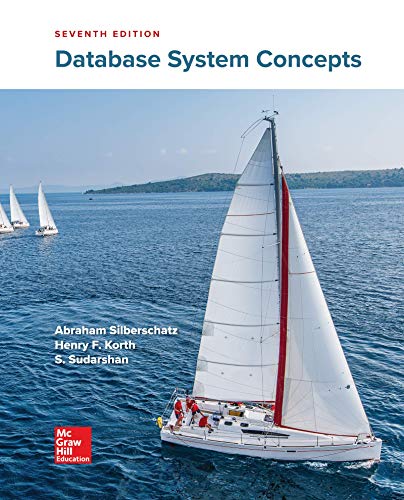
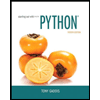
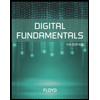
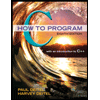
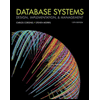
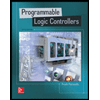