Prototypes for your user defined functions are: 1. void openFile (ifstream&, string); 2. void readFile (ifstream&, PurchaseType[]); 3. void printPurchaseData (PurchaseType [], int);
Prototypes for your user defined functions are: 1. void openFile (ifstream&, string); 2. void readFile (ifstream&, PurchaseType[]); 3. void printPurchaseData (PurchaseType [], int);
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please write in C++. Please look at the example and the example output.
![**Introduction**
In this lab, you will practice using basic input/output, structs, and arrays of structs. Be sure to read this lab thoroughly, especially the Hand-in Procedure.
**Lab**
To start your lab, you will need a struct to read data into. The struct definition should be placed above `main()` and below `using namespace std`. The struct should be called `PurchaseType` and contain the following fields:
1. `string customerID`
2. `string productName`
3. `double price`
4. `int qtyPurchased`
5. `double taxRate`
Write a complete program that:
1. Uses a user-defined function:
```cpp
void openFile(ifstream& iFile, string prompt)
```
Which gets the name of a file from the user and opens it into `iFile` by:
a. Prompting the user for a filename using `prompt` and reading the filename into a string.
b. Opening the file name obtained in (1a) into `iFile`, and verifying that it opened. If the file does not open, the program should output an error message and continue from (1a) until a valid file name is entered. See the example output for error formatting.
i. The file contains strings, integers, and doubles with 5 values per line with 10 lines of data in the file.
ii. Each line of data represents a different item purchased by a customer.
2. Creates an array of instances of the `PurchaseType` struct to hold the data from the file obtained in (1).
3. Uses a user-defined function:
```cpp
void readFile(ifstream& iFile, PurchaseType purchases[])
```
Which reads the data from `iFile` into `purchases` by:
a. `iFile` contains rows of data which correspond to the elements of the `PurchaseType` struct. Iterate through `iFile` inserting the rows of data, using `str.find()` `str.substr()`, into `purchases` until eof is hit.
4. Uses a user-defined function:
```cpp
void printPurchaseData(PurchaseType purchases[], int size)
```
Which prints the data contained in `purchases` by:
a. Using `iomanip` to nicely output all the data in a tabular format. You will need to calculate the total price for each product purchased by](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff3d40ab3-26fa-4cc6-a20f-30a7f32ffb5d%2F4e11a68e-3b8d-4f42-94fa-c1c04887c1be%2Fp89frpi_processed.jpeg&w=3840&q=75)
Transcribed Image Text:**Introduction**
In this lab, you will practice using basic input/output, structs, and arrays of structs. Be sure to read this lab thoroughly, especially the Hand-in Procedure.
**Lab**
To start your lab, you will need a struct to read data into. The struct definition should be placed above `main()` and below `using namespace std`. The struct should be called `PurchaseType` and contain the following fields:
1. `string customerID`
2. `string productName`
3. `double price`
4. `int qtyPurchased`
5. `double taxRate`
Write a complete program that:
1. Uses a user-defined function:
```cpp
void openFile(ifstream& iFile, string prompt)
```
Which gets the name of a file from the user and opens it into `iFile` by:
a. Prompting the user for a filename using `prompt` and reading the filename into a string.
b. Opening the file name obtained in (1a) into `iFile`, and verifying that it opened. If the file does not open, the program should output an error message and continue from (1a) until a valid file name is entered. See the example output for error formatting.
i. The file contains strings, integers, and doubles with 5 values per line with 10 lines of data in the file.
ii. Each line of data represents a different item purchased by a customer.
2. Creates an array of instances of the `PurchaseType` struct to hold the data from the file obtained in (1).
3. Uses a user-defined function:
```cpp
void readFile(ifstream& iFile, PurchaseType purchases[])
```
Which reads the data from `iFile` into `purchases` by:
a. `iFile` contains rows of data which correspond to the elements of the `PurchaseType` struct. Iterate through `iFile` inserting the rows of data, using `str.find()` `str.substr()`, into `purchases` until eof is hit.
4. Uses a user-defined function:
```cpp
void printPurchaseData(PurchaseType purchases[], int size)
```
Which prints the data contained in `purchases` by:
a. Using `iomanip` to nicely output all the data in a tabular format. You will need to calculate the total price for each product purchased by

Transcribed Image Text:## Example 3
### Command Line Execution
- **Command**: `g++ -Wall -pedantic -Werror main.cpp`
- **Execution**: `./a.out`
### File Input Attempts
1. **File Name Entered**: *wrong*
- **Error**: Invalid File
2. **File Name Entered**: *incorrect.csv*
- **Error**: Invalid File
3. **File Name Entered**: *invalid.txt*
- **Error**: Invalid File
4. **File Name Entered**: *err*
- **Error**: Invalid File
5. **File Name Entered**: *purchases1.csv*
### Purchase Data Table
| **Customer ID** | **Product Name** | **Price** | **Quantity** | **Tax Rate** | **Total** |
|----------------|------------------|-----------|--------------|--------------|-----------|
| A134CK2 | shirt | 10.00 | 1 | 8.25 | 10.82 |
| A134CK2 | pants | 15.95 | 2 | 8.25 | 34.53 |
| A134CK2 | jacket | 24.49 | 1 | 8.25 | 26.51 |
| 4J37IZ1 | blouse | 19.95 | 1 | 8.25 | 21.64 |
| 4J37IZ1 | pants | 14.98 | 1 | 8.25 | 16.22 |
| 4J37IZ1 | mittens | 9.99 | 3 | 8.25 | 32.44 |
| 4J37IZ1 | socks | 10.99 | 3 | 8.25 | 35.66 |
| B834621 | gloves | 9.99 | 2 | 7.15 | 21.41 |
| B834621 | cap | 24.99 | 2 | 7.15 | 80.33 |
| 27G8ZZY | earrings |
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
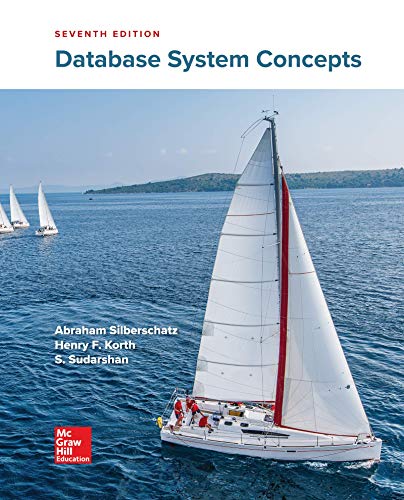
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
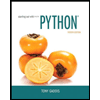
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
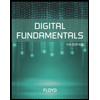
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
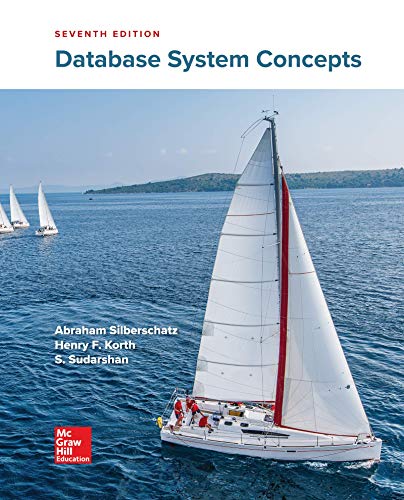
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
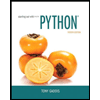
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
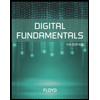
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
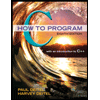
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
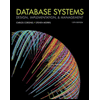
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
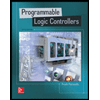
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education