Project 3 (SUBMISSIONS ADDED! (3)) In this project, you will design a simple word processor: Your word processor will take a line of input and align it left, right, or centered. It will also report word count and average word length. To have an idea of what the end result will be, an example execution is shown below. The first four lines are prompts for input, the other lines are outputs of the program: Input: CS Column width: 4 Alignment: center Autocorrect: no CS Words: 1 Avg word length: 2.000000 All input will be less than 1000 characters. You can also assume all inputs are valid, except for column width, which may be an invalid value that you will detect More details are discussed in the following sections. Alignment The text to align is entered via the Input: prompt. Then the user will select a column width with the Column width: prompt and a text alignment via the Alignment: prompt The user will enter a column width, and you will align the text within this column. For example, if the entered text was OS and the column width was 6. you would center it as Note the six hyphens output to show each column CS As seen above, each column number is shown, followed by a separating line of dashes, and then the final output. In the above example, the text is padded with two spaces on each side to center it. The two spaces on the right are not needed and should not be output. In the event that you find yourself with an odd number of spaces to divide, place the extra space on the left. For example, if the column width was 7: CS Examples of the three alignments given a column spacing of 6 are shown below: Left CS Right
Project 3 (SUBMISSIONS ADDED! (3)) In this project, you will design a simple word processor: Your word processor will take a line of input and align it left, right, or centered. It will also report word count and average word length. To have an idea of what the end result will be, an example execution is shown below. The first four lines are prompts for input, the other lines are outputs of the program: Input: CS Column width: 4 Alignment: center Autocorrect: no CS Words: 1 Avg word length: 2.000000 All input will be less than 1000 characters. You can also assume all inputs are valid, except for column width, which may be an invalid value that you will detect More details are discussed in the following sections. Alignment The text to align is entered via the Input: prompt. Then the user will select a column width with the Column width: prompt and a text alignment via the Alignment: prompt The user will enter a column width, and you will align the text within this column. For example, if the entered text was OS and the column width was 6. you would center it as Note the six hyphens output to show each column CS As seen above, each column number is shown, followed by a separating line of dashes, and then the final output. In the above example, the text is padded with two spaces on each side to center it. The two spaces on the right are not needed and should not be output. In the event that you find yourself with an odd number of spaces to divide, place the extra space on the left. For example, if the column width was 7: CS Examples of the three alignments given a column spacing of 6 are shown below: Left CS Right
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
USING C, NOT C++ PLEASE

Transcribed Image Text:15.7 Project 3: Word Processor
Project 3
(SUBMISSIONS ADDED! (3))
In this project, you will design a simple word processor. Your word processor will take a line of input and align it left, right, or centered. It will
also report word count and average word length.
To have an idea of what the end result will be, an example execution is shown below. The first four lines are prompts for input, the other
lines are outputs of the program:
Input: CS
Column width: 4
Alignment: center
Autocorrect: no
----
CS
Words: 1
Aug word length: 2.000000
All input will be less than 1000 characters. You can also assume all inputs are valid, except for column width, which may be an invalid value
that you will detect.
More details are discussed in the following sections.
Alignment
The text to align is entered via the Input: prompt. Then the user will select a column width with the Column width: prompt and a text
alignment via the Alignment: prompt.
The user will enter a column width, and you will align the text within this column. For example, if the entered text was CS and the column
width was 6. you would center it as:
Note the six hyphens output to show each column
C3
As seen above, each column number is shown, followed by a separating line of dashes, and then the final output.
In the above example, the text is padded with two spaces on each side to center it. The two spaces on the right are not needed and should
not be output.
In the event that you find yourself with an odd number of spaces to divide, place the extra space on the left. For example, if the column
width was 7:
Examples of the three alignments given a column spacing of 6 are shown below:
Left
CS
CS
Right
C8

Transcribed Image Text:Centered
If the column width is less than the length of the input, your program should print out the follow text (ends with newline) and exit by
returning 1:
C8
Invalid column width
Additional Details
In addition to alignment, your program will also implement a rudimentary autocorrect feature, count the number of words, and report the
average word length.
Autocorrect
Due to OOR errors, or in an attempt to avoid content filters, words may contain errors such as H3110 (H three one one zero) instead of
Hello. Your program should provide the user the option to correct these errors. If the user chooses to correct them, you will:
.
.
Replace each 3 (number 3) with an e (lowercase letter E)
Replace each 1 (number 1) with an 1 (lowercase letter L)
• Replace each e (number 0) with an o (lowercase letter O)
Replace each 7 (number 7) with a t (lowercase letter T)
When you prompt the user with Autocorrect:, they should be able to enter yes or no.
In addition to these replacements, when autocorrect is on, you should also capitalize the first character of every sentence. This will be the
first character of the input and any character following. (period and a space). Finally, ensure the output ends with a period if it doesn't
already. Example:
hallo world. this is a sentence => Hello world. This is a sentence.
Here you will see the first h was capitalized, the 3 was replaced with an e, the t in this was capitalized, and a period was added to the end
of the sentence.
If you add a period to the end, increase the column width by 1 as well to compensate for the increase in string length.
Word count and average length
Word count: For simplicity, we will define word count as the number of spaces plus 1.
Average word length: Output the average word length as %1f. A word's length is defined as the count of alphabet characters (a-z, A-Z) in
the word:
Example
A complete example of how to handle input and display output is shown below:
Input: Hi world
Column width: 20
Alignment: center
Autocorrect: no
Hi world
Words: 2
Aug word length: 3.500000
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
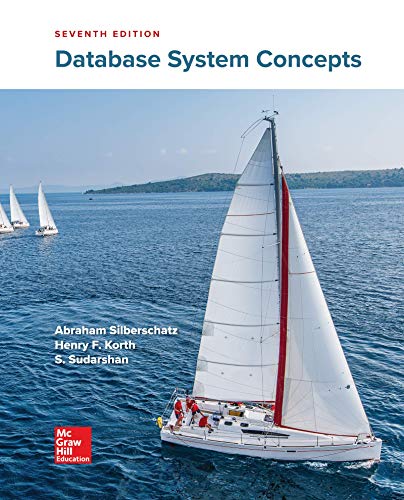
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
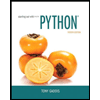
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
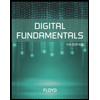
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
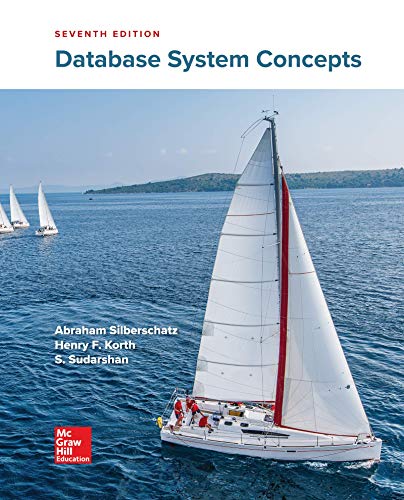
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
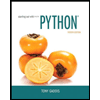
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
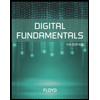
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
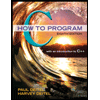
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
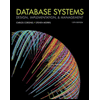
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
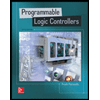
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education