Programming Exercise 03 - Math, Random, Enums Authors: Ananay, Owen, Chloe, Ruchi Problem Description Water. Earth. Fire. Air. While the Avatar cycle has seemingly ended, the 4 nations are preparing for war and it is up to you, fellow 1331 student, to help determine who will win! As each nation gathers their armies, the fire nation decides to attack the 3 other nations, forcing the remaining 3 to ally with each other. As all the nations summon heroes to help the m defeat the enemy, and you will have to write a program to determine which army has the strongest support from their heroes. Your assignment will consist of 2 main parts: Determining how many heroes are summoned, which nation they belong to and what statistics they have. Printing out the hero information and averaging it out for the hero's kingdom to see who wins. Some notes for all variables in the assignment: I. When asked to generate a random variable, you must use java.util.Random When asked to generate a floating-point value, round the value to 2 decimal places. You can only use Math.round () to round the value, but remember that you have operators like *,-, and / to help you I. achieve proper rounding. Solution Description 1. Create a public enum called BendingType. a. This special "class" should be defined in a separate file. 2. Populate the enum with the values AIR, WATER, FIRE, EARTH, NON_BENDER. Create a public class called BendingBattlefield 3. Create the main method 5. Inside, create the following variables: 4. An instance of Random named rand, An int called numHeroes and assign to it a random value in the range [50, 100]. A double called boomerangBoost and assign to it a random value in the range [3, 7). а. b. с. Note the interval notation: [a, b] means a number x such that a <= x <= b. (a, b] means a number x such that a < x <= b. [a, b) means a number x such that a <= x < b. (a, b) means a number x such that a < x < b. 6. Create four double variables: averageFirePower, averageFireHealth, averageAlliancePower, and averageAllianceHealth. 7. Print numHeroes with the following format: "Selecting heroes." 8. Create an int named numFire that will keep a running total of the number of heroes belonging to the FIRE nation. 9. Create an int named numAlliance that will keep a running total of the number of heroes belonging to the AIR, WATER, EARTH, Or NON BENDER nations.
Programming Exercise 03 - Math, Random, Enums Authors: Ananay, Owen, Chloe, Ruchi Problem Description Water. Earth. Fire. Air. While the Avatar cycle has seemingly ended, the 4 nations are preparing for war and it is up to you, fellow 1331 student, to help determine who will win! As each nation gathers their armies, the fire nation decides to attack the 3 other nations, forcing the remaining 3 to ally with each other. As all the nations summon heroes to help the m defeat the enemy, and you will have to write a program to determine which army has the strongest support from their heroes. Your assignment will consist of 2 main parts: Determining how many heroes are summoned, which nation they belong to and what statistics they have. Printing out the hero information and averaging it out for the hero's kingdom to see who wins. Some notes for all variables in the assignment: I. When asked to generate a random variable, you must use java.util.Random When asked to generate a floating-point value, round the value to 2 decimal places. You can only use Math.round () to round the value, but remember that you have operators like *,-, and / to help you I. achieve proper rounding. Solution Description 1. Create a public enum called BendingType. a. This special "class" should be defined in a separate file. 2. Populate the enum with the values AIR, WATER, FIRE, EARTH, NON_BENDER. Create a public class called BendingBattlefield 3. Create the main method 5. Inside, create the following variables: 4. An instance of Random named rand, An int called numHeroes and assign to it a random value in the range [50, 100]. A double called boomerangBoost and assign to it a random value in the range [3, 7). а. b. с. Note the interval notation: [a, b] means a number x such that a <= x <= b. (a, b] means a number x such that a < x <= b. [a, b) means a number x such that a <= x < b. (a, b) means a number x such that a < x < b. 6. Create four double variables: averageFirePower, averageFireHealth, averageAlliancePower, and averageAllianceHealth. 7. Print numHeroes with the following format: "Selecting heroes." 8. Create an int named numFire that will keep a running total of the number of heroes belonging to the FIRE nation. 9. Create an int named numAlliance that will keep a running total of the number of heroes belonging to the AIR, WATER, EARTH, Or NON BENDER nations.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Please write in Java code
![Programming Exercise 03 – Math, Random, Enums
Authors: Ananay, Owen, Chloe, Ruchi
Problem Description
Water. Earth. Fire. Air. While the Avatar cycle has seemingly ended, the 4 nations are preparing for war and it is up
to you, fellow 1331 student, to help determine who will win! As each nation gathers their armies, the fire nation
decides to attack the 3 other nations, forcing the remaining 3 to ally with each other. As all the nations summon
heroes to help them defeat the enemy, and you will have to write a program to determine which army has the
strongest support from their heroes.
Your assignment will consist of 2 main parts:
Determining how many heroes are summoned, which nation they belong to and what statistics they have.
Printing out the hero information and averaging it out for the hero's kingdom to see who wins.
Some notes for all variables in the assignment:
When asked to generate a random variable, you must use java.util.Random
II. When asked to generate a floating-point value, round the value to 2 decimal places. You can only use
Math.round () to round the value, but remember that you have operators like * ,-, and / to help you
achieve proper rounding.
I.
Solution Description
1. Create a public enum called BendingType.
This special "class" should I
defined in a separate file.
a.
2. Populate the enum with the values AIR, WATER, FIRE, EARTH, NON_BENDER.
3. Create a public class called BendingBattlefield
4. Create the main method
CS133
5. Inside, create the following variables:
An instance of Random named rand.
An int called numHeroes and assign to it a random value in the range [50, 100].
A double called boomerangBoost and assign to it a random value in the range [3, 7).
a.
b.
с.
Note the interval notation:
[а, b]
(a, b] means a number x such that a <
[a, b) means a number x such that a <= x < b.
means a number x such that a <= x <= b.
x <= b.
(a, b) means a number x such that a < x < b.
6. Create four double variables: averageFirePower, averageFireHealth,
averageAlliancePower, and averageAllianceHealth.
7. Print numHeroes with the following format:
"Selecting <numHeroes> heroes."
8. Create an int named numFire that will keep a running total of the number of heroes belonging to the
FIRE nation.
Create an int named numAlliance that will keep a running total of the number of heroes belonging to
the AIR, WATER, EARTH, or NON BENDER nations.
10. Create a loop that terminates after numHeroes iterations. Perform steps 11 to 17 inside the loop.
9.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5a4b1b2d-f64c-4e21-ba10-42f22541b73f%2Ff20f1444-426c-435c-b8b1-422ef61b9a09%2F2xtfyo_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Programming Exercise 03 – Math, Random, Enums
Authors: Ananay, Owen, Chloe, Ruchi
Problem Description
Water. Earth. Fire. Air. While the Avatar cycle has seemingly ended, the 4 nations are preparing for war and it is up
to you, fellow 1331 student, to help determine who will win! As each nation gathers their armies, the fire nation
decides to attack the 3 other nations, forcing the remaining 3 to ally with each other. As all the nations summon
heroes to help them defeat the enemy, and you will have to write a program to determine which army has the
strongest support from their heroes.
Your assignment will consist of 2 main parts:
Determining how many heroes are summoned, which nation they belong to and what statistics they have.
Printing out the hero information and averaging it out for the hero's kingdom to see who wins.
Some notes for all variables in the assignment:
When asked to generate a random variable, you must use java.util.Random
II. When asked to generate a floating-point value, round the value to 2 decimal places. You can only use
Math.round () to round the value, but remember that you have operators like * ,-, and / to help you
achieve proper rounding.
I.
Solution Description
1. Create a public enum called BendingType.
This special "class" should I
defined in a separate file.
a.
2. Populate the enum with the values AIR, WATER, FIRE, EARTH, NON_BENDER.
3. Create a public class called BendingBattlefield
4. Create the main method
CS133
5. Inside, create the following variables:
An instance of Random named rand.
An int called numHeroes and assign to it a random value in the range [50, 100].
A double called boomerangBoost and assign to it a random value in the range [3, 7).
a.
b.
с.
Note the interval notation:
[а, b]
(a, b] means a number x such that a <
[a, b) means a number x such that a <= x < b.
means a number x such that a <= x <= b.
x <= b.
(a, b) means a number x such that a < x < b.
6. Create four double variables: averageFirePower, averageFireHealth,
averageAlliancePower, and averageAllianceHealth.
7. Print numHeroes with the following format:
"Selecting <numHeroes> heroes."
8. Create an int named numFire that will keep a running total of the number of heroes belonging to the
FIRE nation.
Create an int named numAlliance that will keep a running total of the number of heroes belonging to
the AIR, WATER, EARTH, or NON BENDER nations.
10. Create a loop that terminates after numHeroes iterations. Perform steps 11 to 17 inside the loop.
9.
![11. Create a local BendingType variable called heroType and assign a random BendingType to it.
You can do this using this line:
BendingType heroType = BendingType.values () [rand.nextInt (5)];
a.
12. Create a double variable called health and assign a random value in the range [50, 150).
Make sure all your floating-point values are rounded to 2 decimal places using Math.round ().
а.
13. Create a double variable called power and assign a random value in the range (40, 120] to it.
Make sure all your floating-point values are rounded to 2 decimal places using Math.round ().
14. If the heroType is FIRE, then health and power will be added to averageFireHealth and
а.
averageFirePower, respectively. Increment numFire appropriately.
15. If heroType is NON_BENDER, multiply power by boomerangBoost.
16. If the heroType is NON BENDER, AIR, WATER, or EARTH, then health and power will be added to
averageAllianceHealth and averageAlliancePower, respectively. Increment
numAlliance appropriately.
17. Print the status of the hero. You can follow the following format:
"<heroType> hero has been summoned by his army, adding <power> power
and <health> health to the army."
18. Once the loop has completed, divide each of the averages, averageFirePower,
averageFireHealth, averageAlliancePower, and averageAllianceHealth by their total
number of respective heroe
numFire or numAlliance, to get the correct average.
19. Print out the following statistics:
а.
"The Fire Nation has an average of <averageFirePower> power and
<averageFireHealth> health."
133
b. "The Alliance has an average of <averageAlliancePower> power and
<averageAllianceHealth> health."
20. Calculate whether the Fire nation or the Alliance won by comparing 2 * averagePower + 3 *
averageHealth of each side and checking which one is larger, If they are equal, then the Alliance wins.
If the Fire Nation won, print
C.
а.
"The Fire Nation won!"
b.
else if the Alliance won, print
"The Alliance won!"
Example Outputs
Please refer to the PE clarification thread on Ed for examples.
HINT: To help debug your code, try inserting print statements in places where variables are changed.
Feature Restrictions
There are a few features and methods in Java that overly simplify the concepts we are trying to teach or break our
auto grader. For that reason, do not use any of the following in your final submission:
var (the reserved keyword)
System.exit
Import Restrictions
You can import only the following classes:
java.util. Random](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5a4b1b2d-f64c-4e21-ba10-42f22541b73f%2Ff20f1444-426c-435c-b8b1-422ef61b9a09%2F72uncx_processed.jpeg&w=3840&q=75)
Transcribed Image Text:11. Create a local BendingType variable called heroType and assign a random BendingType to it.
You can do this using this line:
BendingType heroType = BendingType.values () [rand.nextInt (5)];
a.
12. Create a double variable called health and assign a random value in the range [50, 150).
Make sure all your floating-point values are rounded to 2 decimal places using Math.round ().
а.
13. Create a double variable called power and assign a random value in the range (40, 120] to it.
Make sure all your floating-point values are rounded to 2 decimal places using Math.round ().
14. If the heroType is FIRE, then health and power will be added to averageFireHealth and
а.
averageFirePower, respectively. Increment numFire appropriately.
15. If heroType is NON_BENDER, multiply power by boomerangBoost.
16. If the heroType is NON BENDER, AIR, WATER, or EARTH, then health and power will be added to
averageAllianceHealth and averageAlliancePower, respectively. Increment
numAlliance appropriately.
17. Print the status of the hero. You can follow the following format:
"<heroType> hero has been summoned by his army, adding <power> power
and <health> health to the army."
18. Once the loop has completed, divide each of the averages, averageFirePower,
averageFireHealth, averageAlliancePower, and averageAllianceHealth by their total
number of respective heroe
numFire or numAlliance, to get the correct average.
19. Print out the following statistics:
а.
"The Fire Nation has an average of <averageFirePower> power and
<averageFireHealth> health."
133
b. "The Alliance has an average of <averageAlliancePower> power and
<averageAllianceHealth> health."
20. Calculate whether the Fire nation or the Alliance won by comparing 2 * averagePower + 3 *
averageHealth of each side and checking which one is larger, If they are equal, then the Alliance wins.
If the Fire Nation won, print
C.
а.
"The Fire Nation won!"
b.
else if the Alliance won, print
"The Alliance won!"
Example Outputs
Please refer to the PE clarification thread on Ed for examples.
HINT: To help debug your code, try inserting print statements in places where variables are changed.
Feature Restrictions
There are a few features and methods in Java that overly simplify the concepts we are trying to teach or break our
auto grader. For that reason, do not use any of the following in your final submission:
var (the reserved keyword)
System.exit
Import Restrictions
You can import only the following classes:
java.util. Random
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 3 images

Recommended textbooks for you
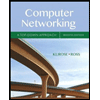
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
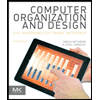
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
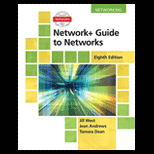
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
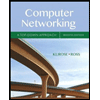
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
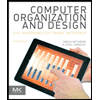
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
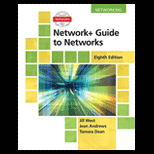
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
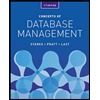
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
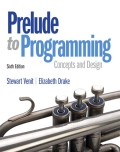
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
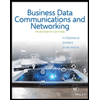
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY