program that lists all ways people
python 3
Write a program that lists all ways people can line up for a photo (all permutations of a list of strings). The program will read a list of one word names, then use a recursive method to create and output all possible orderings of those names, one ordering per line.
When the input is:
Julia Lucas Mia
then the output is (must match the below ordering):
Julia Lucas Mia
Julia Mia Lucas
Lucas Julia Mia
Lucas Mia Julia
Mia Julia Lucas
Mia Lucas Julia
question: is it any way that i can use ('if' statement as base case, and 'else' statement as recursive case) in the code below?
thanks.
code:
def all_permutations(permList, nameList):
# TODO: Implement method to create and output all permutations of the list of names.
def createPermutationsList(nameList):
f = len(nameList)
if f == 0:
return []
if f == 1:
return [nameList]
permList = []
for i in range(f):
newList = nameList[i]
remaining = nameList[:i] + nameList[i+1:]
for p in createPermutationsList(remaining):
permList.append([newList] + p)
return permList
permList = createPermutationsList(nameList)
for p in permList:
for j in p:
print(j, end = " ")
print()
if __name__ == "__main__":
nameList = input().split(' ')
permList = []
all_permutations(permList, nameList)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

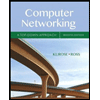
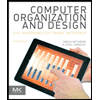
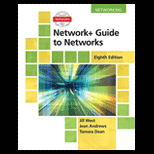
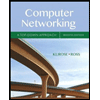
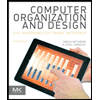
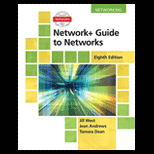
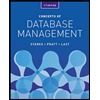
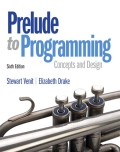
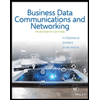