Produce a flowchart for the following code : import string print('Binary Addition') #getting inputs from user x = input('x| enter 4 digits: ') y = input('y| enter 4 digits: ') #store the values of x and y in list and print the lists list1 = list(x) print(list1) list2 = list(y) print(list2) #defining all the logic gates # XOR gate def xorgate(x,y): if x == y: return '0' else: return '1' #AND gate def andgate (x,y): if x == '1' and y == '1': return '1' else: return '0' #ORgate def orgate (x,y): if x == '1' or y == '1': return '1' else: return '0' #define the circuit and provide all the list of inputs to the circuit. #include the outputs depending on the gates function defined above. def circuit(x,y,ci): #x and y are binary digits and ci is the output of the circuit a = 3 #starting the value for while loop,to get appropriate position for our list adder = ['0','0','0','0','0'] #adder list contains strings of zero.the list has to hold the binary numbers obtained by adding circuit b = 4 #check a digit is >=0 while a >= 0: #perform XOR,OR,AND in circuit here output of each operation is given to the next operation xorout = xorgate(x[a],y[a]) print("xor:",(xorout)) s = xorgate(ci,xorout) print("Ci:",ci) andout = andgate(xorout, ci) print("and1:",andout) and2out = andgate(x[a],y[a]) print("and2:",and2out) co = orgate(andout,and2out) print('s:',s) print('co:',co) print('-----------------') ci = co adder[b] = str(s) a-=1 b-=1 adder[0]=ci #print Final Binary output print('The Final Binary Output is:', adder) adder = adder[::-1] #Performing BINARY to decimal conversion outputfinal = ((int(adder[4]) * 16) + (int(adder[3]) * 8)+(int(adder[2]) * 4)+(int(adder[1]) * 2)+(int(adder[0]) * 1)) print('The Final Decimal Output is:', outputfinal) hold = '0' circuit(list1,list2,hold)# 3 value for circuit
Produce a flowchart for the following code :
import string
print('Binary Addition')
#getting inputs from user
x = input('x| enter 4 digits: ')
y = input('y| enter 4 digits: ')
#store the values of x and y in list and print the lists
list1 = list(x)
print(list1)
list2 = list(y)
print(list2)
#defining all the logic gates
# XOR gate
def xorgate(x,y):
if x == y:
return '0'
else:
return '1'
#AND gate
def andgate (x,y):
if x == '1' and y == '1':
return '1'
else:
return '0'
#ORgate
def orgate (x,y):
if x == '1' or y == '1':
return '1'
else:
return '0'
#define the circuit and provide all the list of inputs to the circuit.
#include the outputs depending on the gates function defined above.
def circuit(x,y,ci): #x and y are binary digits and ci is the output of the circuit
a = 3 #starting the value for while loop,to get appropriate position for our list
adder = ['0','0','0','0','0'] #adder list contains strings of zero.the list has to hold the binary numbers obtained by adding circuit
b = 4
#check a digit is >=0
while a >= 0:
#perform XOR,OR,AND in circuit here output of each operation is given to the next operation
xorout = xorgate(x[a],y[a])
print("xor:",(xorout))
s = xorgate(ci,xorout)
print("Ci:",ci)
andout = andgate(xorout, ci)
print("and1:",andout)
and2out = andgate(x[a],y[a])
print("and2:",and2out)
co = orgate(andout,and2out)
print('s:',s)
print('co:',co)
print('-----------------')
ci = co
adder[b] = str(s)
a-=1
b-=1
adder[0]=ci
#print Final Binary output
print('The Final Binary Output is:', adder)
adder = adder[::-1]
#Performing BINARY to decimal conversion
outputfinal = ((int(adder[4]) * 16) + (int(adder[3]) * 8)+(int(adder[2]) * 4)+(int(adder[1]) * 2)+(int(adder[0]) * 1))
print('The Final Decimal Output is:', outputfinal)
hold = '0'
circuit(list1,list2,hold)# 3 value for circuit

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

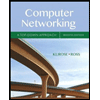
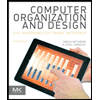
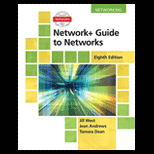
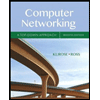
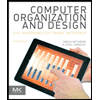
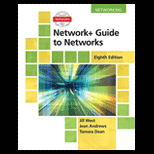
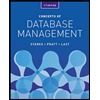
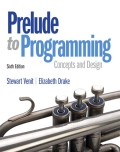
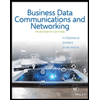